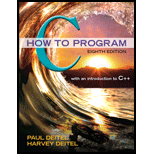
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 12, Problem 12.16E
Program Plan Intro
Program plan:
- item variable is used for input. There is structure Treenode having data, leftptr, rightptr member variables which represents the tree node.
- void insertnode(node **ptr, char *value) function inserts the node in the tree.
- void preorder(node *ptr) function displays the contents of the tree in a preorder fashion.
- void inorder(node *ptr) function displays the contents of the tree in an in-order fashion.
- void postorder(node *ptr) function display the contents of the tree in a post-order fashion.
Program description:
The main purpose of the program is to create a tree from the input entered by user. This violates the binary tree insertion rule and allows the user to enter the duplicate values as well. Then the created tree contents are displayed in preorder, inorder and postorder fashion.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
make a screen capture showing the StegExpose results
Which of the following is not one of the recommended criteria for strategic objectives?
Multiple Choice
a) realistic
b) appropriate
c) sustainable
d) measurable
Management innovations such as total quality, benchmarking, and business process reengineering always lead to sustainable competitive advantage because everyone else is doing them.
a) True
b) False
Chapter 12 Solutions
C How to Program (8th Edition)
Ch. 12 - Prob. 12.6ECh. 12 - (Merging Ordered Lists) Write a program that...Ch. 12 - Prob. 12.8ECh. 12 - (Creating a Linked List, Then Reversing Its...Ch. 12 - Prob. 12.10ECh. 12 - Prob. 12.11ECh. 12 - Prob. 12.12ECh. 12 - Prob. 12.13ECh. 12 - Prob. 12.14ECh. 12 - (Supermarket Simulation) Write a program that...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Vision statements are more specific than strategic objectives. a) True b) Falsearrow_forwardThe three components of the __________ approach to corporate accounting include financial, environmental, and social performance measures. Multiple Choice a) stakeholder b) triple dimension c) triple bottom line d) triple efficiencyarrow_forwardCompetitors, as internal stakeholders, should be included in the stakeholder management consideration of a company and in its mission statement. a) True b) Falsearrow_forward
- At what level in the organization should the strategic management perspective be emphasized? Multiple Choice a) throughout the organization b) from the bottom up in an organization c) at the top of the organization d) at the middle of the organizationarrow_forwardA good manager can be flexible when it comes to sticking to the original plan; to get good results, the intended strategy has to become the realized strategy. a) True b) Falsearrow_forward________ tend to be quite enduring and seldom change. Multiple Choice a) Strategic objectives b) Vision statements c) Strategic plans d) Mission statementsarrow_forward
- The idea that organizations are not only accountable to stockholders but also to the community-at-large is known as social responsibility. a) True b) Falsearrow_forwardAmong the leaders needed for an effective strategic management process are ________, who, although they have little positional power and formal authority, generate their power through the conviction and clarity of their ideas. Multiple Choice a) executive leaders b) triple bottom line advocates c) internal networkers d) local line leadersarrow_forwardI would like to get help to resolve the following casearrow_forward
- Last Chance Securities The IT director opened the department staff meeting today by saying, "I've got some good news and some bad news. The good news is that management approved the payroll system project this morning. The new system will reduce clerical time and errors, improve morale in the payroll department, and avoid possible fines and penalties for noncompliance. The bad news is that the system must be installed by January 1st in order to meet new federal reporting rules, all expenses from now on must be approved in advance, the system should have a modular design if possible, and the vice president of finance would like to announce the new system in a year-end report if it is ready by mid-December." Tasks 1. Why is it important to define the project scope? How would you define the scope of the payroll project in this case? 2. Review each constraint and identify its characteristics: present versus future, internal versus exter- nal, and mandatory versus desirable. 3. What…arrow_forward2. Signed Integers Unsigned binary numbers work for natural numbers, but many calculations use negative numbers as well. To deal with this, a number of different methods have been used to represent signed numbers, but we will focus on two's complement, as it is the standard solution for representing signed integers. 2.1 Two's complement • Most significant bit has a negative value, all others are positive. So, the value of an n-digit -2 two's complement number can be written as: Σ2 2¹ di 2n-1 dn • Otherwise exactly the same as unsigned integers. i=0 - • A neat trick for flipping the sign of a two's complement number: flip all the bits (0 becomes 1, or 1 becomes 0) and then add 1 to the least significant bit. • Addition is exactly the same as with an unsigned number. 2.2 Exercises For questions 1-3, answer each one for the case of a two's complement number and an unsigned number, indicating if it cannot be answered with a specific representation. 1. (15 pts) What is the largest integer…arrow_forwardcan u solve this questionarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
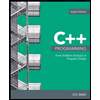
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
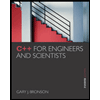
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
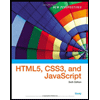
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
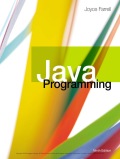
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT