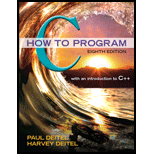
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 12.21E
Program Plan Intro
Program plan:
- Item, start, result variablesare used for input. There is structure listnode havingdata, nextPtrmember variables which represents the linked listnode.
- void insert(node **head, int value) function inserts the node in the a linked list.
- node *recsearch(node *head, int value) function searches the passing value in the linked list using recursion.
- void printList(node *head) function display the contents of the linked list.
Program description:
The main purpose of the program is to create a linked list using the value input by the user and it asks the user to enter the value to search in the linked list and it uses the recursive function to search the value and return the result.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Design and draw a high-level "as-is" process diagram that illustrates a current process related to a product or service offered through the SSDCI.gov database.
Compare last-mile connections for connecting homes and businesses to the Internet
Explain wireless networking standards
Chapter 12 Solutions
C How to Program (8th Edition)
Ch. 12 - Prob. 12.6ECh. 12 - (Merging Ordered Lists) Write a program that...Ch. 12 - Prob. 12.8ECh. 12 - (Creating a Linked List, Then Reversing Its...Ch. 12 - Prob. 12.10ECh. 12 - Prob. 12.11ECh. 12 - Prob. 12.12ECh. 12 - Prob. 12.13ECh. 12 - Prob. 12.14ECh. 12 - (Supermarket Simulation) Write a program that...
Knowledge Booster
Similar questions
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
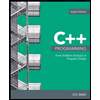
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
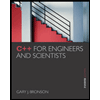
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
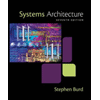
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
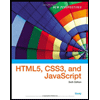
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
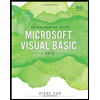
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage