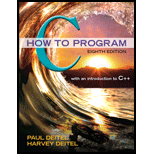
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 12.8E
Program Plan Intro
Program plan:
- Item, start variablesare used for input. There is structure listnode having data, nextPtr member variables which represents the linked list node.
- void insert(node **head, int value) function inserts the node in the a linked list.
- void calculate (node *flist) functioncalculate the sum of the contents of the linked list and then calculate the average value and display the result.
- void printList(node *head) function display the contents of the linked list.
Program description:
The main purpose of the program is to create linked list of 25 values which are generated randomly. Then sum and average of these values are calculated and displayed.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I need help to solve the following case, thank you
hi I would like to get help to resolve the following case
Could you help me to know features of the following concepts:
- defragmenting.
- dynamic disk.
- hardware RAID
Chapter 12 Solutions
C How to Program (8th Edition)
Ch. 12 - Prob. 12.6ECh. 12 - (Merging Ordered Lists) Write a program that...Ch. 12 - Prob. 12.8ECh. 12 - (Creating a Linked List, Then Reversing Its...Ch. 12 - Prob. 12.10ECh. 12 - Prob. 12.11ECh. 12 - Prob. 12.12ECh. 12 - Prob. 12.13ECh. 12 - Prob. 12.14ECh. 12 - (Supermarket Simulation) Write a program that...
Knowledge Booster
Similar questions
- what is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forward
- Show all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
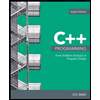
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
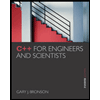
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
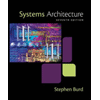
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
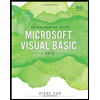
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
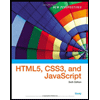
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning