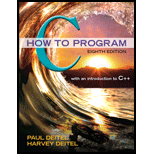
Program plan:
- Item, start variablesare used for input. There is structure listnode having data, nextPtr member variables which represents the linked list node.
- void insert(node **head, int value) function inserts the node in the a linked list.
- node *concat(node *flist, node *slist) function concat the two linked list and return the resultant linked list.
- void printList(node *head) function display the contents of the linked list.
Program description:
The main purpose of the program is to perform the concatenation of two linked list by implanting the concept which is same as strcat() function of string.

Explanation of Solution
Program:
#include <stdio.h> #include <conio.h> #include <alloc.h> #include <ctype.h> //structure of node of the linked list typedefstruct listnode { int data; struct listnode *nextPtr; } node; //function to insert a node in a linked list void insert(node &head,int value); //recursive function to concate the linked list node *concat(node *flist, node *slist); //function to print the content of linked list void printList(node *head); //main starts here void main() { int item; node *flist,*slist; clrscr(); //initialization of start node of linked list flist =NULL; slist =NULL; //loop to getting input from the user for first linked list while(1) { printf("\nEnter value to insert in a First List: 0 to End "); scanf("%d",&item); //check condition to terminate while loop if(item ==0) break; //insert value in a linked list insert(&flist, item); } //loop to getting input from the user for second linked list while(1) { printf("\nEnter value to insert in a Second List: 0 to End "); scanf("%d",&item); //check condition to terminate while loop if(item ==0) break; //insert value in a linked list insert(&slist, item); } // print the contents of linked list printf("\n Content of First List are as follows : "); printList(flist); // print the contents of linked list printf("\n Content of Second List are as follows : "); printList(slist); //call the function to reverse the linked list // and store the address of first node flist = concat(flist, slist); //print the contents of concated list printf("\n Concatenated List contents are as follows : "); printList(flist); getch(); } //function definition to insert node in a linked list void insert(node &head,int value) { node *ptr,*tempnode; //memory allocation for the new node ptr = malloc(sizeof(node)); //copy the value to the new node ptr->data = value; //set node's pointer to NULL ptr->nextPtr =NULL; //if the list is empty if(*head ==NULL) //make the first node *head = ptr; else { //copy the address of first node tempnode =*head; //traverse the list using loop until it reaches //to the last node while(tempnode->nextPtr !=NULL) tempnode = tempnode->nextPtr; //make the new node as last node tempnode->nextPtr = ptr; } } //function defintion to concat the list node *concat(node *flist, node *slist) { node *ptr; //check if list is empty if(flist ==NULL|| slist ==NULL) { printf("\none of the lists is empty"); //return the node returnNULL; } else { //store first node address ptr = flist; //traverse list until last node is not encountered while(ptr->nextPtr !=NULL) ptr = ptr->nextPtr; //store the address of first node of second list ptr->nextPtr = slist; } //return new list return flist; } //function definition to display linked list contents void printList(node *head) { node *ptr; //stores the address of first node ptr = head; //traverse the list until it reaches to the NULL while(ptr !=NULL) { //print the content of current node printf("%d ", ptr->data); //goto the next node ptr = ptr->nextPtr; } }
Explanation:In the above code, a structure is created which represents the node of the linked list. Two starting nodes are initialized which contains the address of first node of each list. User is asked to enter the values for first linked list and linked list is created using insert() function by passing the starting pointer and value. This process is repeated to create the second linked list. printList() function is used to display the contents of both lists. Both lists are concatenated using the concat() function by passing the both list. In this function first list is traversed up to last node and then last node pointer points to the first node of second list. Starting node address is returned and stored in the pointer. Finally, concatenated list is displayed using printList() function.
Sample output:
Want to see more full solutions like this?
Chapter 12 Solutions
C How to Program (8th Edition)
- using r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forwardusing r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forward
- using r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardA. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forward
- Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forwardGiven the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forward
- Considering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forwardGiven the FSA below, answer the following questions. b 1 3 a a b b с 2 A. Write a RegEx that is equivalent to this FSA as it is; B. Write a RegEx that is equivalent to this FSA removing the states and edges corresponding to the letter c. Note: To both items feel free to use any method you want, including analyzing which words are accepted by the FSA, to generate your RegEx.arrow_forward3) Finite State Automata Given the FSA below, answer the following questions. a b a b 0 1 2 b b 3 A. Give three 4-letter words that can be accepted by this FSA; B. Give three 4-letter words that cannot be accepted by this FSA; C. How could you describe the words accepted by this FSA? D. Is this FSA deterministic or non-deterministic?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
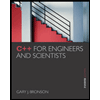
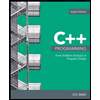
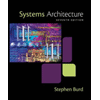
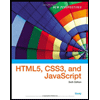