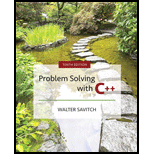
Here is a definition of a class called Pairs. Objects of type Pairs can be used in any situation where ordered pairs are needed. Your task is to write implementations of the overloaded operator >> and the overloaded operator << so that objects of class Pairs are to be input and output in the form (5,6)(5,−4)(−5,4) or (−5,−6). You need not implement any constructor or other member, and you need not do any input format checking.
#include <iostream> using namespace std; class Pairs { public: Pairs( ); Pairs(int first, int second); //other members and friends friend istream& operator >>(istream& ins, Pairs& second); friend ostream& operator <<(ostream& outs, const Pairs& second); private: int f; int s; }; |

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Introduction To Programming Using Visual Basic (11th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with Python (4th Edition)
Mechanics of Materials (10th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
- What is the goal of using a chart in excel, and how is a chart useful and what is the goal of using sparklines in excel, and how are sparklines useful?arrow_forwardProve for each pair of expression f(n) and g(n) whether f(n) is big O, little o Ω,ω or Θ of g(n). Use limits to find these. For each case it is possible that more than one of these conditions is satisfied:1. f(n) =log(n2^n), g(n) = log(sqrt(n)2^(n^2))2. f(n) =nsqrt(n) +log(n^n), g(n) =n + sqrt(n)lognarrow_forwardNeed this expression solved for mu. This can be done using a symbolic toolbox, however it needs to end up being mu = function (theta, m, L, g). If using MATLAB or something similar, run the code to make sure it works.arrow_forward
- A business case scenario and asked to formulate an appropriate software design solution. Theyshould complete the case and upload the solution. will be required to read the case,identify and document the key issues, problems, and opportunities presented, and then design,and develop an appropriate integrated design solution to the problem. mustdemonstrate good spreadsheet, database, analytical, and word-processing skills whendeveloping solutions. Additionally, must be creative and demonstrate synthesising andapplying Database Management and Data Analytics Principles learned in the course. They willalso need to research some aspects of the assessment. CASE BACKGROUNDMGMT SS STATS, an umbrella body that facilitates and serves various Social SecurityOrganizations/Departments within the Caribbean territories, stoodpoised to meet the needs of its stakeholders by launching an onlinedatabase at www.SSDCI.gov. The database will provide membersand the public access to the complete set of…arrow_forwardA business case scenario and asked to formulate an appropriate software design solution. Theyshould complete the case and upload the solution. will be required to read the case,identify and document the key issues, problems, and opportunities presented, and then design,and develop an appropriate integrated design solution to the problem. mustdemonstrate good spreadsheet, database, analytical, and word-processing skills whendeveloping solutions. Additionally, must be creative and demonstrate synthesising andapplying Database Management and Data Analytics Principles learned in the course. They willalso need to research some aspects of the assessment. CASE BACKGROUNDMGMT SS STATS, an umbrella body that facilitates and serves various Social SecurityOrganizations/Departments within the Caribbean territories, stoodpoised to meet the needs of its stakeholders by launching an onlinedatabase at www.SSDCI.gov. The database will provide membersand the public access to the complete set of…arrow_forwardUsing MATLAB symbolic toolbox, given these 3 equations, how would you solve for mu = function(theta), making sure that there are no mu's on the right hand side, making sure theta-dot-dot, theta-dot-squared- and N aren't in the final answer either.arrow_forward
- After playing our giving implementation, your task is to implement Dinning Philosophers with semaphore in C, by including and Your implementation will require creating five philosophers, each identified by a number 0.4. Each philosopher will run as a separate thread. Create threads using Pthreads as discussed in the Lecture slides on Chapter 4 and Practice Lab on Threads. Your solution needs to accomplish the following: Implement in C (15 points) 1. dp1.c - You are to provide your solution to this assignment as a single C program named 'dp1.c using semaphore. Explain in you code (as comments) that the dead lock will happen or not. If there is a possible deadlock, you can simply solve the deadlock by pick the fork in order like the first solution in our slides. Solve Deadlock by Footman (15 points) 1. Here is a new solution to overcome the deadlock. The Dining Philosophers decide to hire a footman whose task to allow only four philosophers to sit on the table. When entering and…arrow_forward8.4 Self-Bias Configuration 20. Determine Zi. Zo. and A,, for the network of Fig. 8.73 if gf, = 3000 μS and gos = 50 μs. 21. Determine Z, Zo, and A, for the network of Fig. 8.73 if the 20-uF capacitor is removed and the parameters of the network are the same as in Problem 20. Compare results with those of Problem 20. +12 V 3.3 ΚΩ HE C₂ Vo Z Zo C₁ 10 ΜΩ Z₁ 1.1 ΚΩ Cs 20 µF FIG. 8.73 Problems 20, 21, 22, and 59.arrow_forward21. Determine Zi, Zo, and A, for the network of Fig. 8.73 if the 20-μF capacitor is removed and the parameters of the network are the same as in Problem 20. Compare results with those of Problem 20. +12 V 3.3 ΚΩ +6 C₂ C₁ Z₁ 10 ΜΩ 1.1 ΚΩ Cs 20 μF FIG. 8.73 Zoarrow_forward
- Ninth Edition Determine Zi, Zo and Av 20 V Zi + 1 ΜΩ 2 ΚΩ HH Z IDSS= 6MA Vp=-6V Yos = 40μS 20 and 47arrow_forwardWhat is the worst case time complexity of the following algorithm for i = 1 to x do for j = 2^((i-1)x) to 2^(in) do print(i,j)arrow_forwardProve for each pair of expression f(n) and g(n) whether f(n) is big O, little o Ω,ω or Θ of g(n). For each case it is possible that more than one of these conditions is satisfied:1. f(n) =log(n2^n), g(n) = log(sqrt(n)2^(n^2))2. f(n) =nsqrt(n) +log(n^n), g(n) =n + sqrt(n)lognarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
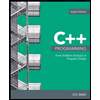
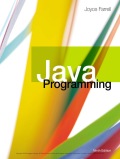
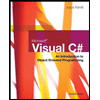
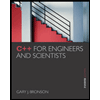
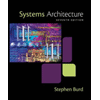