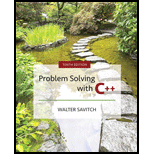
Concept explainers
Define a class for complex numbers. A complex number is a number of the form
a + b * i |
where, for our purposes, a and b are numbers of type double, and i is a number that represents the quantity. Represent a complex number as two values of type double. Name the member variables real and imaginary. (The variable for the number that is multiplied by i is the one called imaginary.)
Call the class Complex. Include a constructor with two parameters of type double that can be used to set the member variables of an object to any values. Also include a constructor that has only a single parameter of type double; call this parameter realPart and define the constructor so that the object will be initialized to realPart+0*i. Also include a default constructor that initializes an object to 0 (that is, to 0+0*i). Overload all of the following operators so that they correctly apply to the type Complex: ==, +, −, *, >>, and <<. You should write a test program to test your class.
(Hints: To add or subtract two complex numbers, you add or subtract the two member variables of type double. The product of two complex numbers is given by the following formula:
(a + b*i)*(c + d*i) == (a*c – b*d) + (a*d + b*c)*i |
In the interface file, you should define a constant i as follows:
const Complex i(0, 1); |
This defined constant i will be the same as the i discussed earlier.
delete p; |

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
SURVEY OF OPERATING SYSTEMS
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forwardwhats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forward
- What is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forwardConsider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forward
- assume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forwardConsider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forward
- Given the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forwardWhat are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
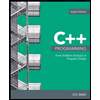
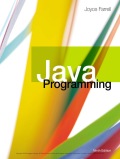
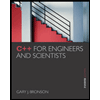
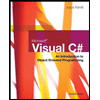
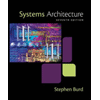