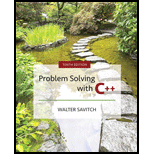
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 11.1, Problem 4STE
Explanation of Solution
Function declaration:
// Declaration of subtract() function
friend Money subtract(Money amount1, Money amount2);
The above function declaration is the “subtract()” friend function.
- In the class “Money”, add the declaration part in the “public” section below the “equal()” function declaration.
Function definition:
// Definition of substract() function
Money subtract(Money amount1, Money amount2)
{
// Create an object for Money class
Money temp;
// Compute amount and assign to temp...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Q4: Consider the following MAILORDER relational schema describing the data for a mail order
company. (Choose five only).
PARTS(Pno, Pname, Qoh, Price, Olevel)
CUSTOMERS(Cno, Cname, Street, Zip, Phone)
EMPLOYEES(Eno, Ename, Zip, Hdate)
ZIP CODES(Zip, City)
ORDERS(Ono, Cno, Eno, Received, Shipped)
ODETAILS(Ono, Pno, Qty)
(10 Marks)
I want a detailed explanation to
understand the mechanism how it is
Qoh stands for quantity on hand: the other attribute names are self-explanatory. Specify and
execute the following queries using the RA interpreter on the MAILORDER database schema.
a. Retrieve the names of parts that cost less than $20.00.
b. Retrieve the names and cities of employees who have taken orders for parts costing more than
$50.00.
c. Retrieve the pairs of customer number values of customers who live in the same ZIP Code.
d. Retrieve the names of customers who have ordered parts from employees living in Wichita.
e. Retrieve the names of customers who have ordered parts costing less…
Q4: Consider the following MAILORDER relational schema describing the data for a mail order
company. (Choose five only).
(10 Marks)
PARTS(Pno, Pname, Qoh, Price, Olevel)
CUSTOMERS(Cno, Cname, Street, Zip, Phone)
EMPLOYEES(Eno, Ename, Zip, Hdate)
ZIP CODES(Zip, City)
ORDERS(Ono, Cno, Eno, Received, Shipped)
ODETAILS(Ono, Pno, Qty)
Qoh stands for quantity on hand: the other attribute names are self-explanatory. Specify and
execute the following queries using the RA interpreter on the MAILORDER database schema.
a. Retrieve the names of parts that cost less than $20.00.
b. Retrieve the names and cities of employees who have taken orders for parts costing more than
$50.00.
c. Retrieve the pairs of customer number values of customers who live in the same ZIP Code.
d. Retrieve the names of customers who have ordered parts from employees living in Wichita.
e. Retrieve the names of customers who have ordered parts costing less than$20.00.
f. Retrieve the names of customers who have not placed…
ut
da
Q4: Consider the LIBRARY relational database schema shown in Figure below
a. Edit Author_name to new variable name where previous
surname was 'Al - Wazny'.
Update book_Authors
set Author_name = 'alsaadi'
where Author_name = 'Al - Wazny'
b. Change data type of Phone to string instead
of numbers.
عرفنه شنو الحل
BOOK
Book id Title Publisher_name
BOOK AUTHORS
Book id Author_name
PUBLISHER
Name Address Phone
e u
al b
are
rage
c. Add two Publishers Company existed in UK.
insert into publisher(name, address, phone)
value('ali','uk',78547889), ('karrar', 'uk', 78547889)
d. Remove all books author when author name contains second character 'D' and ending by
character 'i'.
es inf
rmar
nce 1
tic
عرفته شنو الحل
e. Add one book as variables data?
عرفته شنو الحل
Chapter 11 Solutions
Problem Solving with C++ (10th Edition)
Ch. 11.1 - Write a function definition for a function called...Ch. 11.1 - What is the difference between a friend function...Ch. 11.1 - Suppose you wish to add a friend function to the...Ch. 11.1 - Prob. 4STECh. 11.1 - Notice the member function output in the class...Ch. 11.1 - Notice the definition of the member function input...Ch. 11.1 - The Pitfall section entitled Leading Zeros in...Ch. 11.1 - Give the complete definition of the member...Ch. 11.1 - Why would it be incorrect to add the modifier...Ch. 11.1 - What are the differences and the similarities...
Ch. 11.1 - Given the following definitions: const int x = 17;...Ch. 11.2 - What is the difference between a (binary) operator...Ch. 11.2 - Prob. 13STECh. 11.2 - Suppose you wish to overload the operator = so...Ch. 11.2 - Prob. 15STECh. 11.2 - Give the definition for the constructor discussed...Ch. 11.2 - Here is a definition of a class called Pairs....Ch. 11.2 - Following is the definition for a class called...Ch. 11.3 - Give a type definition for a structure called...Ch. 11.3 - Write a program that reads in five amounts of...Ch. 11.3 - Change the class TemperatureList given in Display...Ch. 11.3 - Prob. 22STECh. 11.3 - If a class is named MyClass and it has a...Ch. 11.4 - Prob. 24STECh. 11.4 - The following is the first line of the copy...Ch. 11.4 - Answer these questions about destructors. a. What...Ch. 11.4 - a. Explain carefully why no overloaded assignment...Ch. 11 - Modify the definition of the class Money shown in...Ch. 11 - Self-Test Exercise 17 asked you to overload the...Ch. 11 - Self-Test Exercise 18 asked you to overload the...Ch. 11 - Prob. 1PPCh. 11 - Define a class for rational numbers. A rational...Ch. 11 - Define a class for complex numbers. A complex...Ch. 11 - Enhance the definition of the class StringVar...Ch. 11 - Define a class called List that can hold a list of...Ch. 11 - Define a class called StringSet that will be used...Ch. 11 - This programming project requires you to complete...Ch. 11 - Redo Programming Project 6 from Chapter 9 (or do...Ch. 11 - Solution to Programming Project 11.12 To combat...Ch. 11 - Repeat Programming Project 11 from Chapter 10 but...Ch. 11 - Do Programming Project 19 from Chapter 8 except...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Add a timer in the following code. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private Timer timer; private long elapsedTime; public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; String playerName = JOptionPane.showInputDialog("Enter your name:"); player.setName(playerName); elapsedTime = 0; timer = new Timer(1000, e -> { elapsedTime++; repaint(); }); timer.start(); } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); int cellSize = Math.min(getWidth() / labyrinth.getSize(), getHeight() / labyrinth.getSize());}arrow_forwardChange the following code so that when player wins the game, the game continues by creating new GameGUI with the same player. However the player's starting position is same, everything else should be reseted. public static void main(String[] args) { Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Random rand = new Random(); Dragon dragon = new Dragon(rand.nextInt(10), 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.setLayout(new BorderLayout()); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(gui, BorderLayout.CENTER); frame.pack(); frame.setResizable(false); frame.setVisible(true); } public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private Timer timer; private long…arrow_forwardCreate a menu item which restarts the game. Also add a timer, which counts the elapsed time since the start of the game level. When the restart is pressed, the restarted game should ask the player' name (in the GameGUI constructor) and set the score of player to 0 (player.setScore(0)), and the timer should restart again. And create a logic so that if the player loses his life (checkGame if the condition is false), then save this number together with his name into two variables. And display two buttons where one quits the game altogether (System.exit(0)) and the other restarts the game. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private final ImageIcon playerIcon = new ImageIcon("data/images/player.png"); private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png"); private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png"); private final ImageIcon…arrow_forward
- Please original work Analyze the complexity issues of processing big data What are five complexities and talk about the reasons they make the implementation complex. Please cite in text references and add weblinksarrow_forwardCreate a Database in JAVA Netbeans that saves name of the player and how many labyrinths did the player solve. Record the number of how many labyrinths did the player solve, and if he loses his life, then save this number together with his name into the database. Create a menu item, which displays a highscore table of the players for the 10 best scores public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over."); System.exit(0); } } } public…arrow_forwardCreate a Database in JAVA OOP that saves name of the player and how many labyrinths did the player solve. Record the number of how many labyrinths did the player solve, and if he loses his life, then save this number together with his name into the database. Create a menu item, which displays a highscore table of the players for the 10 best scores. Also, create a menu item which restarts the game. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over.");…arrow_forward
- Change the following code so that the player can see only the neighboring fields at a distance of 3 units. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private final ImageIcon playerIcon = new ImageIcon("data/images/player.png"); private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png"); private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png"); private final ImageIcon emptyIcon = new ImageIcon("data/images/empty.png"); public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; setFocusable(true); addKeyListener(new KeyAdapter() { @Override public void keyPressed(KeyEvent e) { char move = switch (e.getKeyCode()) { case KeyEvent.VK_W -> 'W'; case…arrow_forwardQ/ Fill in the table below with the correct answers for the network devices and components required, then draw the topology diagram for the network of this three-story building: I want a drawing Cisco Packet tracer Ground Floor: This floor will house the main server room, reception area, and a few conference rooms. The server room should contain the core network devices that will connect the entire building. The reception area and conference rooms need network access require access for mobile devices for visitors and guests with devices. First Floor: This floor is dedicated to offices with workstations, each needing reliable network connectivity for staff computers, IP phones, IP camera, and printers. Second Floor (Education Department): This floor consists of educational spaces requiring controlled internet access. Only the educational website (https://uokerbala.edu.iq) should be accessible, with all other sites restricted.. Device Media type Location floor Type of IP Static/dynamic…arrow_forwardProblem Statement You are working as a Devops Administrator. Y ou’ve been t asked to deploy a multi - tier application on Kubernetes Cluster. The application is a NodeJS application available on Docker Hub with the following name: d evopsedu/emp loyee This Node JS application works with a mongo database. MongoDB image is available on D ockerHub with the following name: m ongo You are required to deploy this application on Kubernetes: • NodeJS is available on port 8888 in the container and will be reaching out to por t 27017 for mongo database connection • MongoDB will be accepting connections on port 27017 You must deploy this application using the CL I . Once your application is up and running, ensure you can add an employee from the NodeJS application and verify by going to Get Employee page and retrieving your input. Hint: Name the Mongo DB Service and deployment, specifically as “mongo”.arrow_forward
- I need help in server client project. It is around 1200 lines of code in both . I want to meet with the expert online because it is complicated. I want the server send a menu to the client and the client enters his choice and keep on this until the client chooses to exit . the problem is not in the connection itself as far as I know.I tried while loops but did not work. please help its emergentarrow_forwardI need help in my server client in C languagearrow_forwardExercise docID document text docID document text 1 hot chocolate cocoa beans 7 sweet sugar 2345 9 cocoa ghana africa 8 sugar cane brazil beans harvest ghana 9 sweet sugar beet cocoa butter butter truffles sweet chocolate 10 sweet cake icing 11 cake black forest Clustering by k-means, with preprocessing tokenization, term weighting TFIDF. Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
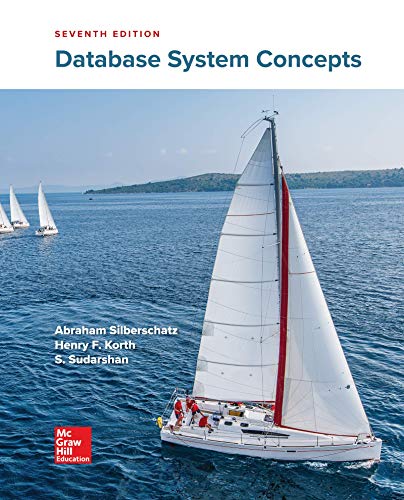
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
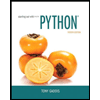
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
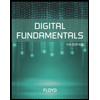
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
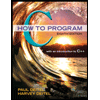
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
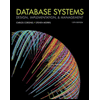
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
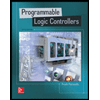
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education