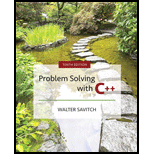
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 11.3, Problem 22STE
Program Plan Intro
- Declare an “double” constant function named “getTemperature()” in the class “TemperatureList”.
- Define a “getTemperature()” function to return the temperature position on the list.
- “if” statement to check the “position” is greater than or equal to “size” or “position” is lesser than “0”.
- The condition is true, print the error message.
- Otherwise, return the temperature position on the list.
- The function having the keyword “const”.
- The keyword “const” will tell the compiler that this member function should not change the value of its calling object.
- “if” statement to check the “position” is greater than or equal to “size” or “position” is lesser than “0”.
- The condition is true, print the error message.
- Otherwise, return the temperature position on the list.
- The function having the keyword “const”.
- The keyword “const” will tell the compiler that this member function should not change the value of its calling object.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
1. Using one of the method described in class and/or textbook (Section 9.1) convert the following
regular expression into a state transition diagram:
(0+ 10*1)* (01 + 10)
Indicate in your answer how did you arrive at the result as follows: Write down all the state
transition diagrams that you constructed for all the subexpressions and clearly indicate which
diagram corresponds to which expression. Do not simplify any state transition diagram.
2. Consider the following state transition diagram over Σ = {a,b}:
b
A
a
a
C
b
B
a
a
b
D
За
a
Using the method described in class and in the textbook (Section 9.2) convert the diagram
into an equivalent regular expression. Include all the intermediate steps in your answer.
3. Are the languages L1, L2, and L3 below over the alphabet Σ = {a, b, c} regular or non-regular?
Justify your answer carefully.
(a) L₁ = {a¹b2jc²i : i ≥ 0, j > 2}
(b) L₂ = L₁n {akbm c³p: k,m,p≥ 0}
(c) L3 = {a²ib²j+1 : i,j ≥ 0}^{akbm c³p : k,m,p ≥ 0}
(1 point)
By dragging statements from the left column to the right column below, give a proof by induction of the following statement:
an
=
= 9" - 1 is a solution to the recurrence relation an = 9an-18 with ao
=
: 0.
The correct proof will use 8 of the statements below.
Statements to choose from:
Note that a₁ =
9a0 + 8.
Now assume that P(n) is true for all n ≥ 0.
Your Proof: Put chosen statements in order in this
column and press the Submit Answers button.
Let P(n) be the predicate, "a = 9″ – 1".
απ = 90 − 1 =
Note that
Let P(n) be the predicate, "an
9" - 1 is a
solution to the recurrence relation an = 9an-1 +8
with ao = 0."
-
Now assume that P(k + 1) is true.
Thus P(k) is true for all k.
Thus P(k+1) is true.
Then ak+1
=
9ak +8, so P(k + 1) is true.
= 1 − 1 = 0, as required.
Then = 9k — 1.
ak
Now assume that P(k) is true for an arbitrary
integer k ≥ 1.
By the recurrence relation, we have
ak+1 =
ak+1 =
= 9ak + 8 = 9(9k − 1) + 8
This simplifies to
9k+19+8 = 9k+1 − 1
Then 9k+1 − 1 = 9(9*…
Given the following request to an AI chatbot, which response is more accurate? (You should use Google to verify that the factual claims in each response are accurate.)
Prompt
Is this true? The first recorded use of the phrase "when pigs fly" in print is in a 1616 play by Ben Jonson called "The Devil is an Ass," in which a character says, "When pigs fly, good-night!!"
Response
Response A
Response B
Yes, that's true! The phrase "when pigs fly" indeed appears in Ben Jonson's 1616 play The Devil is an Ass and was said during the second act in a conversation between Fitzdottrel and Pug. The phrase was first used to express skepticism or disbelief, suggesting that something is as unlikely to happen as the fanciful idea of pigs flying. This usage is often cited as one of the earliest instances of the phrase in print.
The claim that the phrase "when pigs fly" was first recorded in Ben Jonson's 1616 play "The Devil is an Ass" is not accurate. There is no evidence from the…
Chapter 11 Solutions
Problem Solving with C++ (10th Edition)
Ch. 11.1 - Write a function definition for a function called...Ch. 11.1 - What is the difference between a friend function...Ch. 11.1 - Suppose you wish to add a friend function to the...Ch. 11.1 - Prob. 4STECh. 11.1 - Notice the member function output in the class...Ch. 11.1 - Notice the definition of the member function input...Ch. 11.1 - The Pitfall section entitled Leading Zeros in...Ch. 11.1 - Give the complete definition of the member...Ch. 11.1 - Why would it be incorrect to add the modifier...Ch. 11.1 - What are the differences and the similarities...
Ch. 11.1 - Given the following definitions: const int x = 17;...Ch. 11.2 - What is the difference between a (binary) operator...Ch. 11.2 - Prob. 13STECh. 11.2 - Suppose you wish to overload the operator = so...Ch. 11.2 - Prob. 15STECh. 11.2 - Give the definition for the constructor discussed...Ch. 11.2 - Here is a definition of a class called Pairs....Ch. 11.2 - Following is the definition for a class called...Ch. 11.3 - Give a type definition for a structure called...Ch. 11.3 - Write a program that reads in five amounts of...Ch. 11.3 - Change the class TemperatureList given in Display...Ch. 11.3 - Prob. 22STECh. 11.3 - If a class is named MyClass and it has a...Ch. 11.4 - Prob. 24STECh. 11.4 - The following is the first line of the copy...Ch. 11.4 - Answer these questions about destructors. a. What...Ch. 11.4 - a. Explain carefully why no overloaded assignment...Ch. 11 - Modify the definition of the class Money shown in...Ch. 11 - Self-Test Exercise 17 asked you to overload the...Ch. 11 - Self-Test Exercise 18 asked you to overload the...Ch. 11 - Prob. 1PPCh. 11 - Define a class for rational numbers. A rational...Ch. 11 - Define a class for complex numbers. A complex...Ch. 11 - Enhance the definition of the class StringVar...Ch. 11 - Define a class called List that can hold a list of...Ch. 11 - Define a class called StringSet that will be used...Ch. 11 - This programming project requires you to complete...Ch. 11 - Redo Programming Project 6 from Chapter 9 (or do...Ch. 11 - Solution to Programming Project 11.12 To combat...Ch. 11 - Repeat Programming Project 11 from Chapter 10 but...Ch. 11 - Do Programming Project 19 from Chapter 8 except...
Knowledge Booster
Similar questions
- This is for my Computer Organization & Assembly Language Classarrow_forwardPlease answer the homework scenario below and make a JAVA OOP code. You have been hired by GMU to create and manage their course registration portal. Your first task is to develop a program that will create and track different courses in the portal. Each course has the following properties: • a course number ex. IT 106, IT 206, • A course description, ex. Intro to Programming • Total credit hour ex. 3.0, and • current enrollment ex. 30 Each course must have at least a course number and credit hours. The maximum enrollment for each course is 40 students. The current enrollment should be no greater than the maximum enrollment. A course can have a maximum of 4 credit hour. The DDC should calculate the number of seats remaining for the course. Design an object-oriented solution to create a data definition class for the course object. The course class must define all the constructors, mutators with proper validation, accessors, and special purpose methods. The DDC should calculate the…arrow_forwardFor this case study, students will analyze the ethical considerations surrounding artificial intelligence and big data in healthcare, as explored in the case study found in the textbook (pages 34-36) and in the extended version available here There will also be additional articles in this weeks learning module to show both sides of the coin. https://www.delftdesignforvalues.nl/wp-content/uploads/2018/03/Saving-the-life-of-medical-ethics-in-the-age-of-AI-and-Big-Data.pdf Students should refer to the syllabus for specific guidelines regarding length, format, and content requirements. Reflection Questions to Consider: What are the key ethical dilemmas presented in the case? How does AI challenge traditional medical ethics principles such as autonomy, beneficence, and confidentiality? In what ways can responsible innovation help address moral overload in healthcare decision-making? What are the potential risks and benefits of integrating AI-driven decision-making into patient care?…arrow_forward
- Can you please solve this. Thanksarrow_forwardcan you solve this pleasearrow_forwardIn the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forward
- In the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forwardSend me the lexer and parserarrow_forwardHere is my code please draw a transition diagram and nfa on paper public class Lexer { private static final char EOF = 0; private static final int BUFFER_SIZE = 10; private Parser yyparser; // parent parser object private java.io.Reader reader; // input stream public int lineno; // line number public int column; // column // Double buffering implementation private char[] buffer1; private char[] buffer2; private boolean usingBuffer1; private int currentPos; private int bufferLength; private boolean endReached; // Keywords private static final String[] keywords = { "int", "print", "if", "else", "while", "void" }; public Lexer(java.io.Reader reader, Parser yyparser) throws Exception { this.reader = reader; this.yyparser = yyparser; this.lineno = 1; this.column = 0; // Initialize double buffering buffer1 = new char[BUFFER_SIZE]; buffer2 = new char[BUFFER_SIZE]; usingBuffer1 = true; currentPos = 0; bufferLength = 0; endReached = false; // Initial buffer fill fillBuffer(); } private…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
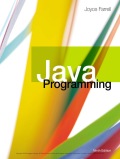
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
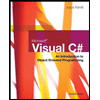
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
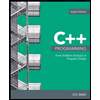
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
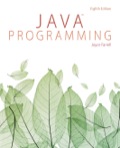
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage