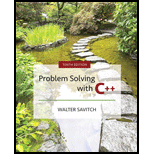
Self-Test Exercise 17 asked you to overload the operator >> and the operator << for a class Pairs. Complete and test this exercise. Implement the default constructor and the constructors with one and two int parameters. The one-parameter constructor should initialize the first member of the pair; the second member of the pair is to be 0. Overload binary operator+ to add pairs according to the rule
(a, b) + (c, d) = (a + c, b + d)
Overload operator– analogously.
Overload operator* on Pairs and int according to the rule
(a, b) * c = (a * c, b * c)
Write a program to test all the member functions and overloaded operators in your class definition.

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
Degarmo's Materials And Processes In Manufacturing
Java: An Introduction to Problem Solving and Programming (8th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- LocalResource(String date, String sector)- Actions of the constructor include accepting a date in the format “dd/mm/yyyy”, initializing the base class, storing the sector and storing an id as a consecutively increasing integer. Note that the constructor must ensure that the date of birth information is recorded. b. getId():Integer - returns the ID of the current instance of LocalResource c. getSector():String – returns the sector associated with the current instance of localResource d. getTRN():String – returns the trn number of the current instance of LocalResource. The process used to determine the TRN is to add the id to the number 100000000 and then returning the string equivalent. e. Update the NineToFiver class to ensure it properly extends the LocalResource class f. In method getContact() of NineToFiver, remove the comments so that the method returns "Local Employee #"+and the id of the contact. Write a concrete class LocalConsultant that extends LocalResource, and implements…arrow_forwardPython Program: Auction Housearrow_forward1- Write a class that allows you to define complex numbers in C++ and call it complex. The class contains two private members named real and img. It also has a constructor to initialize its members to values other than zero. Then, write a four methods that implement the basic mathematical operations (+,-.*) for two complex numbers. 2- The purpose of the method resize() in the IntegerArray class below is to dynamically change the size of the IntegerArray instance. This methid is implemented as follows: 1. create a new array with the desired size 2. copy the data form the old to new one 3. delete the old data 4. Make the old data pointer points to the newly created data 5. update the size field your task is to write the resize() method based on the above five steps. #include int main() { using namespace std; IntegerArray a(2); a.data[0] = 4; class IntegerArray { public: a.data[1] = 2; int *data; a.resize(5); int size; cout size = size; solve number 2 } -IntegerArray()) { delete[] data;…arrow_forward
- C++ program Implement a class with the required data fields, constructor, and setter/getter for each of the private data members, and a print() member function. In your program, the class must be fully tested. Implement a class Book. A book has a title, author, year of publication, and ISBN number. Implement a member function toBibtex() that returns a BibTeX string that describes the book. Also please if you are able give me documentation of your work because i need it too.arrow_forwardWrite the definitions of the member functions of the classes arrayListType and unorderedArrayListType that are not given in this chapter. The specific methods that need to be implemented are listed below. Implement the following methods in arrayListType.h: isEmpty isFull listSize maxListSize clearList Copy constructor Implement the following method in unorderedArrayListType.h insertAt Also, write a program (in main.cpp) to test your function.arrow_forwardSUBJECT: OOPPROGRAMMING LANGUAGE: C++ ALSO ADD SCREENSHOTS OF OUTPUT. Write a class Distance to measure distance in meters and kilometers. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class Time to measure time in hours and minutes. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class which has appropriate functions for taking objects of the Distance class and Time class to store time and distance in a file. Make the data members and functions in your program const where applicablearrow_forward
- Talking: Heba Abde QUESTION 1: Implement class myTime, this class should: 1) Encapsulate information about the hours (integer), minutes (integer), seconds (integer), AM PM (String) 2) Forbid invalid times, where the following criteria should be considered: - hours are in the range [1 – 12], default is 12. - minutes are in the range [0 – 59], default is 0. - seconds are in the range [0-59], default is 0. - AM PM can only be either “AM" or "PM", default is "AM" If any forbidden value entered, you should set the value to default value. 3) Two different constructors (default and parameterized) 4) A print function that will print the time as (hours : minutes : seconds PM/AM) Example: 02:45:30 PM 6) implement function ticktack, which adds one to the seconds, notice that if seconds is 59 and you add one, it should become 0 and the minutes should be incremented by one, also, if after incrementing minutes by one it becomes 60, it should be set to 0 and hours must be incremented by 1, if after…arrow_forwardCPSC 131: Introduction to Computer Programming IIProgram 3: Inheritance and Interface1 Description of the ProgramIn this assignment, you will make two classes, Student and Instructor, that inherit from asuperclass Person. The implementation of class Person is given. You will also need to writea test program to test the methods you write for these two classes. The implementationdetails are described as follows.Stage 1: In the first file Student.java, you should include the following additional instancevariables and methods (other than all instance variables and methods inherited from classPerson):• Private instance variables studentID, and major;• A constructor takes four inputs (name, age, studentID and major);• Two additional getter methods to return each of instance variables (accessor);• Two setter methods to change each of instance variables (mutator);• A method toString that converts a student’s information into string form. Thestring should have the format as shown in Figure 1.…arrow_forwardSo form code should be established in a functional way and outcome also.arrow_forward
- Nutritional information (classes/constructors) PYTHON ONLY Complete the FoodItem class by adding a constructor to initialize a food item. The constructor should initialize the name (a string) to "None" and all other instance attributes to 0.0 by default. If the constructor is called with a food name, grams of fat, grams of carbohydrates, and grams of protein, the constructor should assign each instance attribute with the appropriate parameter value. The given program accepts as input a food item name, fat, carbs, and protein and the number of servings. The program creates a food item using the constructor parameters' default values and a food item using the input values. The program outputs the nutritional information and calories per serving for both food items. Ex: If the input is: M&M's10.034.02.01.0 where M&M's is the food name, 10.0 is the grams of fat, 34.0 is the grams of carbohydrates, 2.0 is the grams of protein, and 1.0 is the number of servings, the output is:…arrow_forwardWrite a class ‘Box’ which has three members height, width and depth; To set values and use these members, write mutator and accessor functions for these members. (Hint: there would be 3 Accessor and 3 Mutator functions).arrow_forwardshows the complete anagram.cpp program. use a class to represent theword to be anagrammed. Member functions of the class allow the word to be displayed, anagrammed, and rotated. The main() routine gets a word from the user, creates a word object with this word as an argument to the constructor, and calls the anagram() member function to anagram the word.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
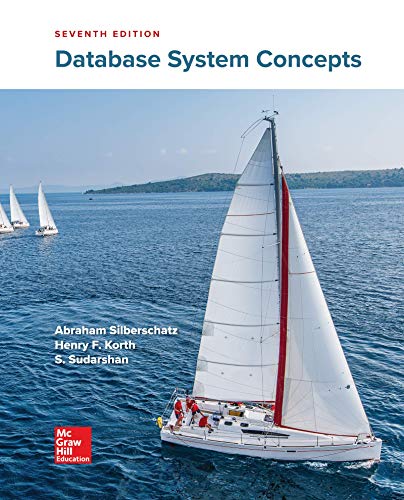
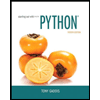
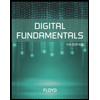
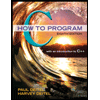
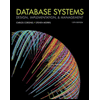
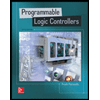