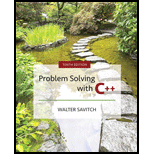
Concept explainers
Notice the member function output in the class definition of Money given in Display 11.3. In order to write a value of type Money to the screen, you call output with cout as an argument. For example, if purse is an object of type Money, then to output the amount of money in purse to the screen, you write the following in your program:
purse.output(cout);
It might be nicer not to have to list the stream cout when you send output to the screen.
Rewrite the class definition for the type Money given in Display 11.3. The only change is that this rewritten version overloads the function name output so that there are two versions of output. One version is just like the one shown in Display 11.3; the other version of output takes no arguments and sends its output to the screen. With this rewritten version of the type Money, the following two calls are equivalent:
purse.output(cout);
and
purse.output();
but the second is simpler. Note that since there will be two versions of the function output, you can still send output to a file. If outs is an output file stream that is connected to a file, then the following will output the money in the object purse to the file connected to outs:
purse.output(outs);

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Modern Database Management
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Starting Out with C++: Early Objects (9th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- What is the type of the stream cin? What is the type of the stream cout?arrow_forwardThe objective of this problem is to show that you can write a program that reads a text file and uses string methods to manipulate the text. File cart.txt contains shopping cart type data from golfsmith.com representing the gifts you have purchased for your dad for this past Fathers Day. Good for you. You have been very generous! The first item in each row of the file is the part number, the second the quantity, the third the price and the fourth the description. Your program will read the file line by line and compute the total cost of all of the items in the list. You will then display a message that looks like this, assuming the cost of each item adds up to 168.42: Here's what your output should look like: The total price for the awesome Fathers Day gifts you bought is $168.42. The shopping cart can be found here: cart.txt. Right click on the link and save the file to the folder where you have your Python files.arrow_forwardDo the following program in Java Eclipse:A veterinarian services many pets and their owners. As new pets are added to the population of pets being serviced, their information is entered into a flat text file. Each month, the vet requests a listing of all pets sorted by their outstanding bill balance. You have to write a program to produce a report of animals and their owners sorted by theiroutstanding bill balances from the data in the flat text file. Below is a description of the information on the text file:• The first entry is the number of animals on the file (numeric)• The fields below repeat for each animal:o Owner name (String)o Birth year (numeric)o Bill balance (numeric)o Species (String)o Special feature (numeric or String)The animals serviced by the veterinarian are of four types: mammals, fish, birds, and reptiles.For a mammal, the special feature field on the flat file is the number of legs of the animal (numeric).For a fish, the special feature field is the type, bony,…arrow_forward
- Write in C++ a class Restaurants to manage a vector of Restaurant objects. The class Restaurants should have: • A private vector to store Restaurant objects • A public add method to add a Restaurant to the Restaurants • A public average_max_rating method that returns the average of max ratings of the restaurants. • A public get_max method that returns the Restaurant that has highest max rating.arrow_forwardWrite a program that contains two classes a Main class and a Student class: • • The Student class has three data fields which are: name, GPA, and SID. It has a constructor that takes SID as a parameter. The Main class is used to declare objects from the Student class. The Main method should take student of type Student then display student ’ ’ s data from the console, store them in an object s data in the console.arrow_forwardWrite a program that accomplishes each of the : " Write a main function that tests input and output of userdefined class Point , using the overloaded stream extraction and stream insertion operators. "arrow_forward
- IN C++ In your previous class, move the member variables (student name and grades) as private. Create the proper functions to fill data in them. In main, create an instance of that array and fill that instance with data. Call the DisplayInfo() function from main so it prints the content of the object. A screenshot showing the code in Visual Studio and the output (on the console screen). previous activity class code:- #include <iostream>using namespace std;class student{ public: string Name; string grade[3]; void DisplayInfo() { cout<<"Student Name:"<<Name; cout<<"\nGrade:"<<grade[1]; }};int main(){ cout << "Welcome to my world..." << endl; system("pause"); student obj; obj.Name="Rahul"; obj.grade[1]="A++"; obj.DisplayInfo(); return 0;}arrow_forwardSolve this in C++arrow_forwardCan you write a new code in C ++ language with the values I sent you, just like this output? There are two files named group1.txt and group2.txt that contain course information and grades of each student for each class. I will calculate each course average for each group and show in simple bar graph. Use "*" and "#"characters for group1 and group2, respectively. I will see the number -999 at the end of each line in the input files. This value is used for line termination and you can use it to verify that you have arrived at the end of the line. The averages of each group should also be calculated and printed at the end of the file. All of the results will be printed to the file. There will be no screen output. Sample output is shown in Figure 1. Group 1: CSC 80 100 70 80 72 90 89 100 83 70 90 73 85 90 -999ENG 80 90 80 94 90 74 78 63 83 80 90 -999HIS 90 70 80 70 90 50 89 83 90 68 90 60 80 -999MTH 74 80 75 89 90 73 90 82 74 90 84 100 90 79 -999PHY 100 83 93 80 63 78 88…arrow_forward
- Can you write a new code in C++ language with the values I sent you, just like this output? There are two files named group1.txt and group2.txt that contain course information and grades of each student for each class. I will calculate each course average for each group and show in simple bar graph. Use "*" and "#"characters for group1 and group2, respectively. I will see the number -999 at the end of each line in the input files. This value is used for line termination and you can use it to verify that you have arrived at the end of the line. The averages of each group should also be calculated and printed at the end of the file.arrow_forwardSUBJECT: OOPPROGRAMMING LANGUAGE: C++ ADD SS OF OUTPUT TOO. Create a class Student with attributes StudentName, Enrollment, semester, section, course MarksObtained and Grade. Write appropriate constructors, get and set functions for data members. Read data from file and print number of Grade occurrences in the following format: A grade: 2 B grade: 1 C grade: 3 D grade: 2 F grade: 3arrow_forwardYou have to write a program to store information of university students. There can be many students in university, so you can make a Student class and objects of class Student to store information. The university is interested in storing information about student’s name, enrollment, department, class, CGPA and gender. You can store this information as data member values for each object.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
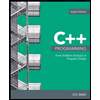