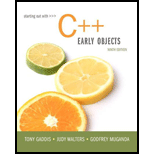
Concept explainers
What will the following
#include <iostream>
#include <memory>
using namespace std;
class Base
{
public:
Base () { cout << "Entering the base .\n"; }
virtual ~Base () { cout << "Leaving the base.\n"; }
} ;
class Camp : public Base
{
public :
Camp() { cout << "Entering the camp.\n"; }
virtual ~Camp() { cout << "Leaving the camp.\n"; }
};
int main()
{
shared _ptr<Camp> outpost = make_shared<Camp> ();
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Starting Out with C++: Early Objects (9th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Modern Database Management
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Introduction To Programming Using Visual Basic (11th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- I want to solve 13.2 using matlab please helparrow_forwarda) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
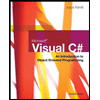
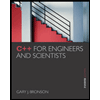
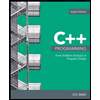
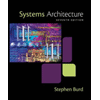
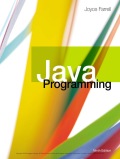