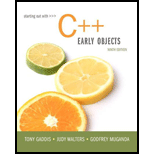
Check Writing
Design a class Numbers that can be used to translate whole dollar amounts in the range 0 through 9999 into an English description of the number. For example, the number 713 would be translated into the string seven hundred thirteen, and 8203 would be translated into eight thousand two hundred three.
The class should have a single integer member variable
int number;
and a collection of static string members that specify how to translate key dollar amounts into the desired format. For example, you might use static strings such as
string 1essThan20[ ] =
{"zero", "one", …, "eighteen", "nineteen" };
string hundred = "hundred";
string thousand = "thousand";
The class should have a constructor that accepts a non-negative integer and uses it to initialize the Numbers object. It should have a member function print ( ) that prints the English description of the Numbers object. Demonstrate the class by writing a main

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Starting Out with C++: Early Objects (9th Edition)
Additional Engineering Textbook Solutions
Concepts Of Programming Languages
Mechanics of Materials (10th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
- Can this be donbe in Java and not C++arrow_forwardC++ Programmingarrow_forwardThe Spider Game Introduction: In this assignment you will be implementing a game that simulates a spider hunting for food using python. The game is played on a varying size grid board. The player controls a spider. The spider, being a fast creature, moves in the pattern that emulates a knight from the game of chess. There is also an ant that slowly moves across the board, taking steps of one square in one of the eight directions. The spider's goal is to eat the ant by entering the square it currently occupies, at which point another ant begins moving across the board from a random starting location. Game Definition: The above Figure illustrates the game. The yellow box shows the location of the spider. The green box is the current location of the ant. The blue boxes are the possible moves the spider could make. The red arrow shows the direction that the ant is moving - which, in this case, is the horizontal X-direction. When the ant is eaten, a new ant is randomly placed on one of the…arrow_forward
- int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // *** returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // *** move the center of the circle to the new coordinates +intersects(Circle other): bool //*** returns true if the center of the other circle lies inside this circle else returns false +resize(double…arrow_forward-int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // * returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // * move the center of the circle to the new coordinates +intersects(Circle other): bool //* returns true if the center of the other circle lies inside this circle else returns false +resize(double scale):void// * multiply the…arrow_forwardObject oriented programming C++ Use Classes Write a C++ program in which each flight is required to have a date of departure, a time of departure, a date of arrival and a time of arrival. Furthermore, each flight has a unique flight ID and the information whether the flight is direct or not.Create a C++ class to model a flight. (Note: Make separate classes for Date and Time and use composition).add two integer data members to the Flight class, one to store the total number of seats in the flight and the other to store the number of seats that have been reserved. Provide all standard functions in each of the Date, Time and Flight classes (Constructors, set/get methods and display methods etc.).Add a member function delayFlight(int) in the Flight class to delay the flight by the number of minutes pass as input to this function. (For simplicity, assume that delaying a flight will not change the Date). Add a member function reserveSeat(int) which reserves the number of seats passed as…arrow_forward
- JAVA PROGRAMMING Write a class named ToDoItem. The class represents a single item in a list of things that a person wants to get done. This class would be useful in an app that helps a person organize their daily activities. The app might show a list of things that need to be done and allow the user to check them off as they are completed. The class must have three member variables: a String that describes the item an integer that stores the priority. (The higher the int value, the higher the priority.) a boolean that stores whether or not the item is completed. The Class should have three constructors: ToDoItem(String description, int priority, boolean isCompleted) ToDoItem(String description, int priority) ToDoItem(String description) In the second constructor isCompleted should be set to false. In the third constructor isCompleted should be set to false, and priority should be set to 0. Your class should have getters, but not setters, for the description, priority, and…arrow_forwardC++arrow_forwardAssignment 4 - Car Maintenance This assignment will assess your understanding of void and value returning static methods. Your cousin owns an automotive maintenance shop that performs routine maintenance on cars. He has asked you to write a program that will provide a list of services (and their respective prices) to users and allow them to choose any, or all, services they would like and display the final price, including a 28% labor charge, a 9% markup if the car is an import, and an 8% sales tax. Task To accomplish the above, do the following: • Write the two methods outlined below. • Test your program and screenshot your successful test. carMaintenance method This method should accept the make of the car as a parameter (e.g., BMW, Ford, Ferrari, etc.). It should display the services and their prices as follows: Services: Oil Change, Tune up, Brake Job, Transmission Service, respectively: 59.99, 111.99, 189.99, 149.99 These should be stored in parallel arrays. Ask the user to…arrow_forward
- Integer userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward2. Car Class Write a class named car that has the following fields: ▪ yearModel. The year Model field is an int that holds the car's year model. ▪ make. The make field is a String object that holds the make of the car, such as "Ford", "Chevrolet", "Honda", etc. ▪ speed. The speed field is an int that holds the car's current speed. In addition, the class should have the following methods. ■ Constructor. The constructor should accept the car's year model and make as arguments. These values should be assigned to the object's year Model and make fields. The constructor should also assign 0 to the speed field.arrow_forwardPlease help me with this using java. Please use explain each li e of code using commentsarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
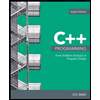
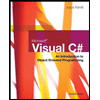