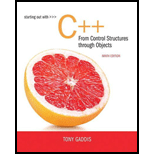
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 11, Problem 18RQE
Program Plan Intro
Structure array:
- The structure needs an array if the items needs to be grouped together.
- Array is a “derived” data type and it contains similar elements.
Syntax:
struct_name array_name[size];
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
reminder it an exercice not a grading work
GETTING STARTED
Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website.
Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”.
If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically.
With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet.
If cell B6 does not display your name, delete the file and download a new copy from the SAM website.
Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…
Need help completing this algorithm here in coding! 2
Whats wrong the pseudocode here??
Chapter 11 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 11.4 - Prob. 11.1CPCh. 11.4 - Write a definition statement for a variable of the...Ch. 11.4 - Prob. 11.3CPCh. 11.6 - For Questions 11.4-11.7 below, assume the Product...Ch. 11.6 - Write a loop that will step through the entire...Ch. 11.6 - Prob. 11.6CPCh. 11.6 - Prob. 11.7CPCh. 11.6 - Write a structure declaration named Measurement,...Ch. 11.6 - Write a structure declaration named Destination,...Ch. 11.6 - Write statements that store the following data in...
Ch. 11.10 - Prob. 11.11CPCh. 11.10 - Write a function that uses a Rectangle structure...Ch. 11.10 - Prob. 11.13CPCh. 11.10 - Prob. 11.14CPCh. 11.10 - Prob. 11.15CPCh. 11.11 - Look at the following declaration: enum Flower {...Ch. 11.11 - What will the following code display? enum {...Ch. 11.11 - Prob. 11.18CPCh. 11.11 - What will the following code display? enum Letters...Ch. 11.11 - Prob. 11.20CPCh. 11.11 - Prob. 11.21CPCh. 11 - Prob. 1RQECh. 11 - Prob. 2RQECh. 11 - Prob. 3RQECh. 11 - Look at the following structure declaration:...Ch. 11 - Look at the following structure declaration:...Ch. 11 - Look at the following code: struct PartData {...Ch. 11 - Look at the following code: struct Town { string...Ch. 11 - Look at the following code: structure Rectangle {...Ch. 11 - Prob. 9RQECh. 11 - Look at the following declaration: enum Person {...Ch. 11 - Prob. 11RQECh. 11 - The ______ is the name of the structure type.Ch. 11 - The variables declared inside a structure...Ch. 11 - A(n) ________ is required after the closing brace...Ch. 11 - In the definition of a structure variable, the...Ch. 11 - Prob. 16RQECh. 11 - Prob. 17RQECh. 11 - Prob. 18RQECh. 11 - Prob. 19RQECh. 11 - Prob. 20RQECh. 11 - Declare a structure named TempScale, with the...Ch. 11 - Write statements that will store the following...Ch. 11 - Write a function called showReading. It should...Ch. 11 - Write a function called findReading. It should use...Ch. 11 - Write a function called getReading, which returns...Ch. 11 - Prob. 26RQECh. 11 - Prob. 27RQECh. 11 - Look at the following statement: enum Color { RED,...Ch. 11 - A per store sells dogs, cats, birds, and hamsters....Ch. 11 - T F A semicolon is required after the closing...Ch. 11 - T F A structure declaration does not define a...Ch. 11 - T F The contents of a structure variable can be...Ch. 11 - T F Structure variables may not be initialized.Ch. 11 - Prob. 34RQECh. 11 - Prob. 35RQECh. 11 - T F The following expression refers to element 5...Ch. 11 - T F An array of structures may be initialized.Ch. 11 - Prob. 38RQECh. 11 - T F A structure member variable may be passed to a...Ch. 11 - T F An entire structure may not be passed to a...Ch. 11 - T F A function may return a structure.Ch. 11 - T F when a function returns a structure, it is...Ch. 11 - T F The indirection operator has higher precedence...Ch. 11 - Prob. 44RQECh. 11 - Find the Errors Each of the following...Ch. 11 - Prob. 46RQECh. 11 - struct TwoVals { int a, b; }; int main () {...Ch. 11 - #include iostream using namespace std; struct...Ch. 11 - #include iostream #include string using namespace...Ch. 11 - struct FourVals { int a, b, c, d; }; int main () {...Ch. 11 - Prob. 51RQECh. 11 - struct ThreeVals { int a, b, c; }; int main () {...Ch. 11 - Prob. 1PCCh. 11 - Movie Profit Modify the program written for...Ch. 11 - Prob. 3PCCh. 11 - Weather Statistics Write a program that uses a...Ch. 11 - Weather Statistics Modification Modify the program...Ch. 11 - Soccer Scores Write a program that stores the...Ch. 11 - Customer Accounts Write a program that uses a...Ch. 11 - Search Function for Customer Accounts Program Add...Ch. 11 - Speakers Bureau Write a program that keeps track...Ch. 11 - Prob. 10PCCh. 11 - Prob. 11PCCh. 11 - Course Grade Write a program that uses a structure...Ch. 11 - Drink Machine Simulator Write a program that...Ch. 11 - Inventory Bins Write a program that simulates...
Knowledge Booster
Similar questions
- Help! how do I fix my python coding question for this? (my code also provided)arrow_forwardNeed help with coding in this in python!arrow_forwardIn the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forward
- Please provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forwardWhy I need ?arrow_forwardHere are two diagrams. Make them very explicit, similar to Example Diagram 3 (the Architecture of MSCTNN). graph LR subgraph Teacher_Model_B [Teacher Model (Pretrained)] Input_Teacher_B[Input C (Complete Data)] --> Teacher_Encoder_B[Transformer Encoder T] Teacher_Encoder_B --> Teacher_Prediction_B[Teacher Prediction y_T] Teacher_Encoder_B --> Teacher_Features_B[Internal Features F_T] end subgraph Student_B_Model [Student Model B (Handles Missing Labels)] Input_Student_B[Input C (Complete Data)] --> Student_B_Encoder[Transformer Encoder E_B] Student_B_Encoder --> Student_B_Prediction[Student B Prediction y_B] end subgraph Knowledge_Distillation_B [Knowledge Distillation (Student B)] Teacher_Prediction_B -- Logits Distillation Loss (L_logits_B) --> Total_Loss_B Teacher_Features_B -- Feature Alignment Loss (L_feature_B) --> Total_Loss_B Partial_Labels_B[Partial Labels y_p] -- Prediction Loss (L_pred_B) --> Total_Loss_B Total_Loss_B -- Backpropagation -->…arrow_forward
- Please provide me with the output image of both of them . below are the diagrams code I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder --> Student_A_Features[Student A Features F_A] end subgraph Knowledge_Distillation_A [Knowledge Distillation (Student A)] Teacher_Prediction -- Logits Distillation Loss (L_logits_A) --> Total_Loss_A Teacher_Features -- Feature Alignment Loss (L_feature_A) --> Total_Loss_A Ground_Truth_A[Ground Truth y_gt] -- Prediction Loss (L_pred_A)…arrow_forwardI'm reposting my question again please make sure to avoid any copy paste from the previous answer because those answer did not satisfy or responded to the need that's why I'm asking again The knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges…arrow_forwardThe knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges (missing values vs. missing labels), they should not be combined in the same diagram. Instead, create two separate diagrams for clarity. For reference, I will attach a second image…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
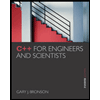
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
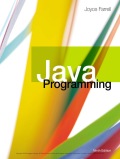
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
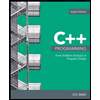
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
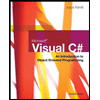
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
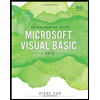
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning