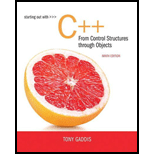
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 11, Problem 1RQE
Explanation of Solution
Primitive data type:
Primitive data types are pre-defined data types, which can be used directly by the programmers to declare the variables.
Some of the primitive data types available in C++
Integer:
- Integer is one of the predefined data types. The keyword used for declaring integer data type is “int”.
- It takes up to 4 bytes of memory space to store data. It ranges from -147483648 to 2147483647.
- Example for declaring integer data type is as follows:
- int variable1 = 10;
Floating point:
- Floating point is a predefined data type. The keyword used for declaring floating point is “float”.
- It takes up to 4 bytes of memory space to store data. It ranges from +/- 3.4e +/- 38.
- Example for declaring floating point data type is as follows:
- float variable1 = 10.3;
Character:
- Character is a predefined data type. The keyword used for declaring character data type is “char”.
- It takes 1 byte of memory space to store data. It ranges from -127 to 127 or 0 to 255
- Example for declaring character data type is as follows:
- char variable1 = 'a';
Boolean:
- Boolean is a predefined data type. The keyword used for declaring Boolean data type is “bool”. It stores data in the form of “true” or “false”.
- Example for declaring Boolean data type is as follows:
- bool variable1 = true;
Double Floating point:
- Double Floating point is a predefined data type. The keyword used for declaring double is “double”.
- It takes up to 8 bytes of memory space to store data. It ranges from +/- 1.7e +/- 308
- Example for declaring double data type is as follows:
- double variable1 = 11.34;
Void:
- Void represents null value. The keyword used for void is “void”.
Wide Character:
- Wide Character is a predefined data type. The keyword used for declaring wide character is “wchar_t”.
Example:
The below program demonstrates the usage of primitive data type “integer”:
//Include the header files
#include<iostream>
using namespace std;
//Main function
int main()
{
//Variable declaration
int i, n = 5;
//for loop to print 1 to 5
for (i = 1; i <= n; i++)
{
//print the value of “i”
cout << i << "\n";
}
//Print a new line
cout << "\n";
//Return the value 0
return 0;
}
Expert Solution & Answer

Sample Output
1
2
3
4
5
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Students have asked these similar questions
solve this questions for me .
a) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?
Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as the
Chapter 11 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 11.4 - Prob. 11.1CPCh. 11.4 - Write a definition statement for a variable of the...Ch. 11.4 - Prob. 11.3CPCh. 11.6 - For Questions 11.4-11.7 below, assume the Product...Ch. 11.6 - Write a loop that will step through the entire...Ch. 11.6 - Prob. 11.6CPCh. 11.6 - Prob. 11.7CPCh. 11.6 - Write a structure declaration named Measurement,...Ch. 11.6 - Write a structure declaration named Destination,...Ch. 11.6 - Write statements that store the following data in...
Ch. 11.10 - Prob. 11.11CPCh. 11.10 - Write a function that uses a Rectangle structure...Ch. 11.10 - Prob. 11.13CPCh. 11.10 - Prob. 11.14CPCh. 11.10 - Prob. 11.15CPCh. 11.11 - Look at the following declaration: enum Flower {...Ch. 11.11 - What will the following code display? enum {...Ch. 11.11 - Prob. 11.18CPCh. 11.11 - What will the following code display? enum Letters...Ch. 11.11 - Prob. 11.20CPCh. 11.11 - Prob. 11.21CPCh. 11 - Prob. 1RQECh. 11 - Prob. 2RQECh. 11 - Prob. 3RQECh. 11 - Look at the following structure declaration:...Ch. 11 - Look at the following structure declaration:...Ch. 11 - Look at the following code: struct PartData {...Ch. 11 - Look at the following code: struct Town { string...Ch. 11 - Look at the following code: structure Rectangle {...Ch. 11 - Prob. 9RQECh. 11 - Look at the following declaration: enum Person {...Ch. 11 - Prob. 11RQECh. 11 - The ______ is the name of the structure type.Ch. 11 - The variables declared inside a structure...Ch. 11 - A(n) ________ is required after the closing brace...Ch. 11 - In the definition of a structure variable, the...Ch. 11 - Prob. 16RQECh. 11 - Prob. 17RQECh. 11 - Prob. 18RQECh. 11 - Prob. 19RQECh. 11 - Prob. 20RQECh. 11 - Declare a structure named TempScale, with the...Ch. 11 - Write statements that will store the following...Ch. 11 - Write a function called showReading. It should...Ch. 11 - Write a function called findReading. It should use...Ch. 11 - Write a function called getReading, which returns...Ch. 11 - Prob. 26RQECh. 11 - Prob. 27RQECh. 11 - Look at the following statement: enum Color { RED,...Ch. 11 - A per store sells dogs, cats, birds, and hamsters....Ch. 11 - T F A semicolon is required after the closing...Ch. 11 - T F A structure declaration does not define a...Ch. 11 - T F The contents of a structure variable can be...Ch. 11 - T F Structure variables may not be initialized.Ch. 11 - Prob. 34RQECh. 11 - Prob. 35RQECh. 11 - T F The following expression refers to element 5...Ch. 11 - T F An array of structures may be initialized.Ch. 11 - Prob. 38RQECh. 11 - T F A structure member variable may be passed to a...Ch. 11 - T F An entire structure may not be passed to a...Ch. 11 - T F A function may return a structure.Ch. 11 - T F when a function returns a structure, it is...Ch. 11 - T F The indirection operator has higher precedence...Ch. 11 - Prob. 44RQECh. 11 - Find the Errors Each of the following...Ch. 11 - Prob. 46RQECh. 11 - struct TwoVals { int a, b; }; int main () {...Ch. 11 - #include iostream using namespace std; struct...Ch. 11 - #include iostream #include string using namespace...Ch. 11 - struct FourVals { int a, b, c, d; }; int main () {...Ch. 11 - Prob. 51RQECh. 11 - struct ThreeVals { int a, b, c; }; int main () {...Ch. 11 - Prob. 1PCCh. 11 - Movie Profit Modify the program written for...Ch. 11 - Prob. 3PCCh. 11 - Weather Statistics Write a program that uses a...Ch. 11 - Weather Statistics Modification Modify the program...Ch. 11 - Soccer Scores Write a program that stores the...Ch. 11 - Customer Accounts Write a program that uses a...Ch. 11 - Search Function for Customer Accounts Program Add...Ch. 11 - Speakers Bureau Write a program that keeps track...Ch. 11 - Prob. 10PCCh. 11 - Prob. 11PCCh. 11 - Course Grade Write a program that uses a structure...Ch. 11 - Drink Machine Simulator Write a program that...Ch. 11 - Inventory Bins Write a program that simulates...
Knowledge Booster
Similar questions
- whats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forward
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
- What are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
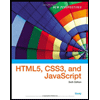
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
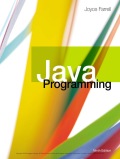
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
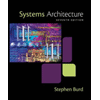
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
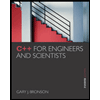
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage