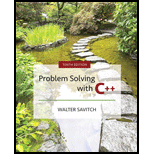
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 10.2, Problem 12STE
Given the following class definition, write an appropriate definition for the member function set:
class Temperature { public: void set(double newDegrees, char newScale); //Sets the member variables to the values given as //arguments. double degrees; char scale; //'F' for Fahrenheit or 'C' for Celsius. }; |
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Among the leaders needed for an effective strategic management process are ________, who, although they have little positional power and formal authority, generate their power through the conviction and clarity of their ideas.
Multiple Choice
a) executive leaders
b) triple bottom line advocates
c) internal networkers
d) local line leaders
I would like to get help to resolve the following case
Last Chance Securities
The IT director opened the department staff meeting today by saying, "I've got some good news and
some bad news. The good news is that management approved the payroll system project this morning.
The new system will reduce clerical time and errors, improve morale in the payroll department, and avoid
possible fines and penalties for noncompliance. The bad news is that the system must be installed by
January 1st in order to meet new federal reporting rules, all expenses from now on must be approved in
advance, the system should have a modular design if possible, and the vice president of finance would
like to announce the new system in a year-end report if it is ready by mid-December."
Tasks
1. Why is it important to define the project scope? How would you define the scope of the payroll
project in this case?
2. Review each constraint and identify its characteristics: present versus future, internal versus exter-
nal, and mandatory versus desirable.
3. What…
Chapter 10 Solutions
Problem Solving with C++ (10th Edition)
Ch. 10.1 - Given the following structure and structure...Ch. 10.1 - Consider the following type definition: struct...Ch. 10.1 - What is the error in the following structure...Ch. 10.1 - Given the following struct definition: struct A {...Ch. 10.1 - Here is an initialization of a structure type....Ch. 10.1 - Write a definition for a structure type for...Ch. 10.1 - Prob. 7STECh. 10.1 - Prob. 8STECh. 10.1 - Give the structure definition for a type named...Ch. 10.1 - Declare a variable of type StockRecord (given in...
Ch. 10.2 - Below we have redefined the class DayOfYear from...Ch. 10.2 - Given the following class definition, write an...Ch. 10.2 - Prob. 13STECh. 10.2 - The private member function DayOfYear::checkDate...Ch. 10.2 - Suppose your program contains the following class...Ch. 10.2 - Suppose you change Self-Test Exercise 15 so that...Ch. 10.2 - Explain what public: and private: do in a class...Ch. 10.2 - a. How many public: sections are required in a...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Suppose your program contains the following class...Ch. 10.2 - How would you change the definition of the class...Ch. 10.2 - Prob. 24STECh. 10.3 - When you define an ADT as a C++ class, should you...Ch. 10.3 - When you define an ADT as a C++ class, what items...Ch. 10.3 - Suppose your friend defines an ADT as a C++ class...Ch. 10.3 - Redo the three- and two-parameter constructors in...Ch. 10.4 - How does inheritance support code reuse and make...Ch. 10.4 - Can a derived class directly access by name a...Ch. 10.4 - Suppose the class SportsCar is a derived class of...Ch. 10 - Solution to Practice Program 10.1 Redefine...Ch. 10 - Redo your definition of the class CDAccount from...Ch. 10 - Define a class for a type called CounterType. An...Ch. 10 - Write a grading program for a class with the...Ch. 10 - Redo Programming Project 1 (or do it for the first...Ch. 10 - Define a class called Month that is an abstract...Ch. 10 - Redefine the implementation of the class Month...Ch. 10 - My mother always took a little red counter to the...Ch. 10 - Write a rational number class. This problem will...Ch. 10 - Define a class called Odometer that will be used...Ch. 10 - Redo Programming Project 7 from Chapter 5 (or do...Ch. 10 - The U.S. Postal Service printed a bar code on...Ch. 10 - Consider a class Movie that contains information...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Determine the magnitude of the resultant force acting on the screw eye and its direction measured clockwise fro...
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Consider the following C program void fun (void) { int a, b, c; / defiinition.1 / . . . while (. . .) int b, c,...
Concepts Of Programming Languages
In the following exercises, write a program to carry out the task. The program should use variables for each of...
Introduction To Programming Using Visual Basic (11th Edition)
What output is produced by the following code? for (int n = 1; n = 5; n++) { if (n == 3) break; System.out.prin...
Java: An Introduction to Problem Solving and Programming (8th Edition)
In chemical machining, should the etchant be applied by spraying or immersion?
Degarmo's Materials And Processes In Manufacturing
This operator can be used to determine whether a reference variable references an object of a particular class....
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 2. Signed Integers Unsigned binary numbers work for natural numbers, but many calculations use negative numbers as well. To deal with this, a number of different methods have been used to represent signed numbers, but we will focus on two's complement, as it is the standard solution for representing signed integers. 2.1 Two's complement • Most significant bit has a negative value, all others are positive. So, the value of an n-digit -2 two's complement number can be written as: Σ2 2¹ di 2n-1 dn • Otherwise exactly the same as unsigned integers. i=0 - • A neat trick for flipping the sign of a two's complement number: flip all the bits (0 becomes 1, or 1 becomes 0) and then add 1 to the least significant bit. • Addition is exactly the same as with an unsigned number. 2.2 Exercises For questions 1-3, answer each one for the case of a two's complement number and an unsigned number, indicating if it cannot be answered with a specific representation. 1. (15 pts) What is the largest integer…arrow_forwardcan u solve this questionarrow_forward1. Unsigned Integers If we have an n-digit unsigned numeral dn-1d n-2...do in radix (or base) r, then the value of that numeral is n−1 r² di Σi=0 which is basically saying that instead of a 10's or 100's place we have an r's or r²'s place. For binary, decimal, and hex r equals 2, 10, and 16, respectively. Just a reminder that in order to write down a large number, we typically use the IEC or SI prefixing system: IEC: Ki = 210, Mi = 220, Gi = 230, Ti = 240, Pi = 250, Ei = 260, Zi = 270, Yi = 280; SI: K=103, M = 106, G = 109, T = 10¹², P = 1015, E = 10¹8, Z = 1021, Y = 1024. 1.1 Conversions a. (15 pts) Write the following using IEC prefixes: 213, 223, 251, 272, 226, 244 21323 Ki8 Ki 223 23 Mi 8 Mi b. (15 pts) Write the following using SI prefixes: 107, 10¹7, 10¹¹, 1022, 1026, 1015 107 10¹ M = 10 M = 1017102 P = 100 P c. (10 pts) Write the following with powers of 10: 7 K, 100 E, 21 G 7 K = 7*10³arrow_forward
- answer shoul avoid using AI and should be basic and please explainarrow_forwardNode A is connected to node B by a 2000km fiber link having a bandwidth of 100Mbps. What is the total latency time (transmit + propagation) required to transmit a 4000 byte file using packets that include 1000 Bytes of data plus 40 Bytes of header.arrow_forwardanswer should avoid using AI and should be basic and explain pleasearrow_forward
- answer should avoid using AI (such as ChatGPT), do not any answer directly copied from AI would and explain codearrow_forwardanswer should avoid using AI (such as ChatGPT), do not any answer directly copied from AI would and explain codearrow_forwardWrite a c++ program that will count from 1 to 10 by 1. The default output should be: 1, 2, 3, 4, 5, 6 , 7, 8, 9, 10 There should be only a newline after the last number. Each number except the last should be followed by a comma and a space. To make your program more functional, you should parse command line arguments and change behavior based on their values. Argument Parameter Action -f, --first yes, an integer Change place you start counting -l, --last yes, an integer Change place you end counting -s, --skip optional, an integer, 1 if not specified Change the amount you add to the counter each iteration -h, —help none Print a help message including these instructions. -j, --joke none Tell a number based joke. So, if your program is called counter, counter -f 10 --last 4 --skip 2 should produce 10, 8, 6, 4 Please use the last supplied argument. If your code is called counter, counter -f 4 -f 5 -f 6 should count from 6. You should…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
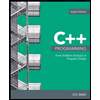
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
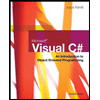
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
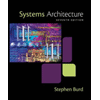
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
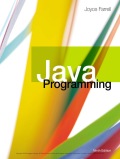
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
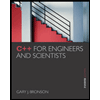
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY