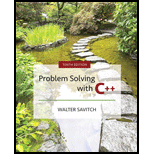
Structure in C++:
Structure is a user defined datatype that is used to combine data items of different data types.
Defining a Structure:
In order to define a structure, the “struct” statement is used. This statement defines a new data type with more than one member.
struct [structure tag] {
member definition;
member definition;
...
Member definition;
}[one or more structure variables];
Here, the “structure tag” is optional and each member definition is a normal variable definition. Specifying one or more structure variables at the end of the structure definition is also optional.
Given:
The following function declaration is given.
void readShoeRecord(ShoeType& newShoe);
//Fills newShoe with values read from the keyboard.

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Problem Solving with C++ (10th Edition)
- Write the definition of a void function that takes as input two integer values, say n and m. The function returns the sum and average of all the numbers between n and m (inclusive) .arrow_forwardINSTRUCTIONS: Write a C++ script/code to do the given problems. MOVIE PROBLEM: Write a function that checks whether a person can watch an R18+ rated movie. One of the following two conditions is required for admittance: The person is atleast 18 years old. • They have parental supervision. The function accepts two parameters, age and isSupervised. Return a boolean. Example: acceptIntoMovie(14, true) → true acceptIntoMovie(14, false) → falsearrow_forwardIn C++ Language (please use hint) : Write a function which will swap its arguments if the first argument is greater than its second argument, but will not interchange them if the first argument is samller than or equal to the second argument. The function should return 1 if a swap was made, and 0 otherwise. (Hint: Make sure to use call by reference.) Write also a short test driver (i.e. a main() invoking your function).arrow_forward
- Define Function printResult.arrow_forwardWrite the definition of a void function that takes as input two parameters of type int, say sum and testScore the function updates the value of sum by adding the value of testScore. The new value of sum is reflected in the calling environment.arrow_forwardUse Clojure to complete these questions: Please note that this is the beginning of the course so please use simple methods possible, thanks a. The built-in function type is useful for checking what kind of object an expression evaluates to. Write a function both-same-type? that takes two arguments, and returns true when they both have the same type, and false otherwise. b. Write a function list-longer-than? which takes two arguments: an integer n, and a list lst and returns true if lst has more than n elements, and false otherwise. For example, (list-longer-than? 3 '(1 2 3)) should return false, and (list-longer-than? 2 '(1 2 3)) should return true. c. See attached picture.arrow_forward
- 1. Consider the function definition: void Demo (int intVal, float& floatVal { intVal = intVal * 2; floatVal = float(intVal) + 3.5; } a. Suppose that the caller has variables int mylnt and float myEloat whose values are 20 and 4.8, respectively. What are the values of mylnt and myEloat after return from the following function call? Demo(mylnt, myEloat); mulnt: mvEloat: b. Also, describe what happens in MEMORY when the Demo function is called. Think about the by reference and by value parameters.arrow_forwardhello, how would I solve this and could you please explain each step and the reason for it? Thank you so much.arrow_forwardhello, how would I solve this and could you please explain each step and the reason for it? Thank you so much.arrow_forward
- 3. Write a function which will swap its arguments if the first argument is greater than its second argument, but will not interchange them if the first argument is smaller than or equal to the second argument. The function should return 1 if a swap was made, and 0 otherwise. (Hint: Make sure to use call by reference.) Write also a short test driver(i.e. a main() invoking your function).arrow_forwardExample 1: Define a function that takes an argument. Call the function. Identify what code is the argument and what code is the parameter.Example 2: Call your function from Example 1 three times with different kinds of arguments: a value, a variable, and an expression. Identify which kind of argument is which. Example 3: Construct a function with a local variable. Show what happens when you try to use that variable outside the function. Explain the results.Example 4: Construct a function that takes an argument. Give the function parameter a unique name. Show what happens when you try to use that parameter name outside the function. Explain the results.Example 5: Show what happens when a variable defined outside a function has the same name as a local variable inside a function. Explain what happens to the value of each variable as the program runs.The code and its output must be explained technically whenever asked. The explanation can be provided before or after the code, or in the…arrow_forwardhello, how would I solve this and could you please explain each step and the reason for it? Thank you so much.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
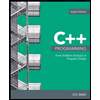