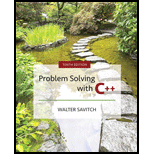
Concept explainers
Define a class for a type called CounterType. An object of this type is used to count things, so it records a count that is a nonnegative whole number. Include a default constructor that sets the counter to zero and a constructor with one argument that sets the counter to the value specified by its argument. Include member functions to increase the count by 1 and to decrease the count by 1. Be sure that no member function allows the value of the counter to become negative. Also, include a member function that returns the current count value and one that outputs the count to a stream. The member function for doing output will have one formal parameter of type ostream for the output stream that receives the output. Embed your class definition in a test

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
C How to Program (8th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Starting Out with Programming Logic and Design (4th Edition)
Software Engineering (10th Edition)
Modern Database Management
Starting Out with Python (3rd Edition)
- Write a full class definition for a class named Counter , and containing the following members: A data member counter of type int . A constructor that takes one int argument and assigns its value to counter . A function called increment that accepts no parameters and returns no value. increment adds one to the counter data member. A function called decrement that accepts no parameters and returns no value. decrement subtracts one to the counter data member. A function called getValue that accepts no parameters. It returns the value of the instance variable counter .arrow_forwardAssigrment Question: Create a Circle class with a centre (x, y) and radius r. Use parameterized constructor to initialize the radius and centre of a circle object. Write a copy constructor that copies the values of one circle object to another one. Use a member function to display the values x, y, r of a circle object. Allow a friend function to update the centre and radius of the circle. There should a static variable 'number_of_circles' and a static member function 'update_number_of_circles' which will update the 'umber_of_circles' whenever an instance of a circle is created.arrow_forwardWrite a Rectangle class that has the following fields: Length (L): a double - Width (W): a double The class should have the following functions: Constructor: accepts the length (L) and the width (W) of a rectangle as arguments. Constructor: does not take any parameters and initializes the length(L) and width(W) to zero. - A function called getArea which returns the area of the rectangle. A function called getPerimeter which returns the perimeter of the rectangle. {note: The area of a rectangle =L*W, and its perimeter = 2*(L+W) } Then, write a program to ask the user to enter the length and width of a rectangle, and then use the above class to calculate and print the area and perimeter of the rectangle.arrow_forward
- C# languageWrite a program to create a class employee, it consist of ID, name, department and address. All employees belongs to “Computer Science” department and it can never be changed by any means. Employee ID is initialized only once when Employee object is created, any further attempt to change ID should be failed. Class must have a 3 parameterized constructor to set values and two methods: print(): to display all the data of a particular employee totalObjects(): to count and print total number of objects that has been created In Main(), create atleast two objects of employee class, display their records by calling print() function and also print the total number of objects that has been created. [this question is continued on next page] Sample Main Method: static void Main(string[] args) { Employee obj1 = new Employee(1, "Zubair", "Karachi"); obj1.print(); Employee obj2 = new Employee(2, "Nabeel", "Islamabad"); obj2.print();…arrow_forwardT/F: Instance variables are shared by all the instances of the class. T/F: The scope of instance and static variables is the entire class. They can be declared anywhere inside a class. T/F: To declare static variables, constants, and methods, use the static modifier.arrow_forwardWhen a class can be derived from another class and use its methods, this is called Polymorphism. A. True B. Falsearrow_forward
- In a class with overloaded constructors, each version of the constructor must have a differentarrow_forwardUser-defined Class: You will design and implement your own data class. The class will store data that has been read as user input from the keyboard (see Getting Input below), and provide necessary operations. As the data stored relates to monetary change, the class should be named MoneyChange. The class requires at least 2 instance variables for the name of a person and the coin change amount to be given to that person. You may also wish to use 6 instance variables to represent amounts for each of the 6 coin denominations (see Client Class below). There should be no need for more than these instance variables. However, if you wish to use more instance variables, you must provide a legitimate justification for their usage in the internal and external documentation.Your class will need to have at least a default constructor, and a constructor with two parameters:one parameter being a name and the other a coin amount. Your class should also provide appropriate get and set methods for…arrow_forwardC++ Language Please Write a program that implements four classes: NPC, Flying, Walking, and Generic for a fantasy roleplaying game. Each class should have the following attributes and methods: NPC -a parent class that defines methods and an attribute common to all non-player characters (npc) in the game. a private string variable named name, for storing the name of the npc. a default constructor for setting name to "placeholder". an overloaded constructor that sets name to a string argument passed to it. setName - a mutator for updating the name attribute getName - an accessor for returning the npc name printStats - a pure virtual function that will be overridden by each NPC subclass. Flying - a subclass of NPC that defines a flying npc in the game a private int variable named flightSpeed for tracking the speed of the npc. a default constructor for setting flightSpeed to 0 and name to "Flying" using setName. setFlightSpeed - a mutator that accepts an integer as it's only argument…arrow_forward
- There are two types of data members in a class: static and non-static. Provide an example of when it might be useful to have a static data member in the actual world.arrow_forward2019 AP® COMPUTER SCIENCE A FREE-RESPONSE QUESTIONS 2. This question involves the implementation of a fitness tracking system that is represented by the StepTracker class. A StepTracker object is created with a parameter that defines the minimum number of steps that must be taken for a day to be considered active. The StepTracker class provides a constructor and the following methods. addDailySteps, which accumulates information about steps, in readings taken once per day activeDays, which returns the number of active days averageSteps, which returns the average number of steps per day, calculated by dividing the total number of steps taken by the number of days tracked The following table contains a sample code execution sequence and the corresponding results. Statements and Expressions Value Returned Comment (blank if no value) StepTracker tr StepTracker(10000); Days with at least 10,000 steps are considered active. Assume that the parameter is positive. new tr.activeDays () ; No…arrow_forwardColorful bubbles In this problem, you will use the Bubble class to make images of colorful bubbles. You will use your knowledge of classes and objects to make an instance of the Bubble class (aka instantiate a Bubble), set its member variables, and use its member functions to draw a Bubble into an image. Every Bubble object has the following member variables: X coordinate Y coordinate Size (i.e. its radius) Color Complete main.cc Your task is to complete main.cc to build and draw Bubble objects based on user input. main.cc already does the work to draw the Bubble as an image saved in bubble.bmp. You should follow these steps: First, you will need to create a Bubble object from the Bubble class. Next, you must prompt the user to provide the following: an int for the X coordinate, an int for the Y coordinate, an int for the Bubble's size, and a std::string for the Bubble's color. Next, you must use the user's input to set the new Bubble object's x and y coordinates, the size, and the…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
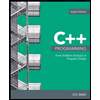
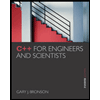
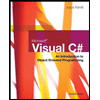
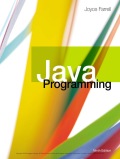