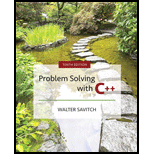
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 10.1, Problem 5STE
Here is an initialization of a structure type. Tell what happens with each initialization. Note any problems with these initializations.
struct Date { int month; int day; int year; }; |
a. Date dueDate = {12, 21};
b. Date dueDate = {12, 21, 20, 22};
c. Date dueDate = {12, 21, 20, 22};
d. Date dueDate = {12, 21, 22};
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
6. What is Race condition? How to prevent it? [2 marks]
7. How many synchronization methods do you know and compare the differences. [2 marks]
8. Explain what are the “mutual exclusion”, “deadlock”, “livelock”, and “eventual entry”, with
the traffic intersection as an example like dinning philosophy. [2 marks]
9. For memory allocation, what are the difference between internal fragmentation and external
fragmentation. Explain with an example. [2 marks]
10. How can the virtual memory map to the physical memory. Explain with an example. [2
marks]
Your answers normally have 50 words. Less than 50 words will not get marks.
1. What is context switch between multiple processes? [2 marks]
2. Draw the memory layout for a C program. [2 marks]
3. How many states does a process has? [2 marks]
4. Compare the non-preemptitve scheduling and preemptive scheduling. [2 marks]
5. Given 4 process and their arrival times and next CPU burst times, what are the average times
and average Turnaround time, for different scheduling algorithms including:
a. First Come, First-Served (FCFS) Scheduling [2 marks]
b. Shortest-Job-First (SJF) Scheduling [2 marks]
c. Shortest-remaining-time-first [2 marks]
d. Priority Scheduling [2 marks]
e. Round Robin (RR) [2 marks]
Process
Arrival Time
Burst Time
P1
0
8
P2
1
9
P3
3
2
P4
5
4
a database with multiple tables from attributes as shown above that are in 3NF,
showing PK, non-key attributes, and FK for each table? Assume the tables are already in
1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]
Chapter 10 Solutions
Problem Solving with C++ (10th Edition)
Ch. 10.1 - Given the following structure and structure...Ch. 10.1 - Consider the following type definition: struct...Ch. 10.1 - What is the error in the following structure...Ch. 10.1 - Given the following struct definition: struct A {...Ch. 10.1 - Here is an initialization of a structure type....Ch. 10.1 - Write a definition for a structure type for...Ch. 10.1 - Prob. 7STECh. 10.1 - Prob. 8STECh. 10.1 - Give the structure definition for a type named...Ch. 10.1 - Declare a variable of type StockRecord (given in...
Ch. 10.2 - Below we have redefined the class DayOfYear from...Ch. 10.2 - Given the following class definition, write an...Ch. 10.2 - Prob. 13STECh. 10.2 - The private member function DayOfYear::checkDate...Ch. 10.2 - Suppose your program contains the following class...Ch. 10.2 - Suppose you change Self-Test Exercise 15 so that...Ch. 10.2 - Explain what public: and private: do in a class...Ch. 10.2 - a. How many public: sections are required in a...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Suppose your program contains the following class...Ch. 10.2 - How would you change the definition of the class...Ch. 10.2 - Prob. 24STECh. 10.3 - When you define an ADT as a C++ class, should you...Ch. 10.3 - When you define an ADT as a C++ class, what items...Ch. 10.3 - Suppose your friend defines an ADT as a C++ class...Ch. 10.3 - Redo the three- and two-parameter constructors in...Ch. 10.4 - How does inheritance support code reuse and make...Ch. 10.4 - Can a derived class directly access by name a...Ch. 10.4 - Suppose the class SportsCar is a derived class of...Ch. 10 - Solution to Practice Program 10.1 Redefine...Ch. 10 - Redo your definition of the class CDAccount from...Ch. 10 - Define a class for a type called CounterType. An...Ch. 10 - Write a grading program for a class with the...Ch. 10 - Redo Programming Project 1 (or do it for the first...Ch. 10 - Define a class called Month that is an abstract...Ch. 10 - Redefine the implementation of the class Month...Ch. 10 - My mother always took a little red counter to the...Ch. 10 - Write a rational number class. This problem will...Ch. 10 - Define a class called Odometer that will be used...Ch. 10 - Redo Programming Project 7 from Chapter 5 (or do...Ch. 10 - The U.S. Postal Service printed a bar code on...Ch. 10 - Consider a class Movie that contains information...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
For the circuit shown, find (a) the voltage υ, (b) the power delivered to the circuit by the current source, an...
Electric Circuits. (11th Edition)
Demonstrate each of the anomaly types with an example.
Modern Database Management
What is pseudocode?
Starting Out With Visual Basic (8th Edition)
Modify the method you wrote for Checkpoint 9.7 so it performs a case-insensitive test. The method should return...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Code an SQL statement that creates a table with all columns from the parent and child tables in your answer to ...
Database Concepts (8th Edition)
The following pseudocode statement calls a function named half, which returns a value that is half that of the ...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forwardIf a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? Order, Product, store, customer.arrow_forward
- Inheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forwardSuppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forward
- Please answer the JAVA OOP Programming Assignment scenario below: Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise. Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise. Create an object-oriented solution with a menu that allows a user to select one of the following options: 1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message. 2. Search Cruise – This option allows to search a cruise by the user provided cruise ID. 3. Remove Cruise – This op…arrow_forwardI need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.arrow_forwardSouthern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forward
- Why is JAVA OOP is really difficult to study?arrow_forwardMy daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
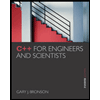
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
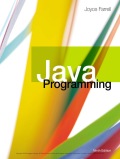
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
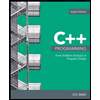
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
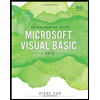
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
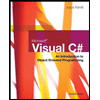
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Structured Chart; Author: Tutorials Point (India) Ltd.;https://www.youtube.com/watch?v=vdUO-sGA1DA;License: Standard YouTube License, CC-BY
Introduction to Structure Charts; Author: Christopher Kalodikis;https://www.youtube.com/watch?v=QN2bjNplGlQ;License: Standard Youtube License