this is my code and the question need to be continued. #include using namespace std; class Course{ private: string name; string *studentList; int size, capacity=10; public: Course(){ name = "TBD"; size = 0; studentList = new string[capacity]; }Course(string s){ name = s; size = 0; studentList = new string[capacity]; }void setName(string s){ name = s; }getter/accessor for variable name string getName(){ return name; } }; int main(){ Course a, b("Yankee"); cout << a.getName() << endl; a.setName("Mets"); cout << a.getName() << endl; cout << b.getName() << endl; return 0; } this is the Question:: Continue with Team class: a) Copy the previous program to a new file. b) Implement the addMember, removeMember and display member functions to Team class. addMember(string) - add a new student with given name to the members. removeMember() - delete the last added member from the members, no deletion if there is no member in the members. display() - display Team information. Use following main() to test your class. int main() { Team a("Mets"); a.removeMember(); // a.display(); a.addMember("David"); a.addMember("Steven"); a.addMember("Sara"); a.addMember("Tom"); a.display(); a.removeMember(); a.display(); a.removeMember(); a.display(); return 0; } Output from given main function: No member to remove Team name:Mets Member List: Team name:Mets Member List: David Steven Sara Tom Team name:Mets Member List: David Steven Sara Team name:Mets Member List: David Steven
this is my code and the question need to be continued.
#include <iostream>
using namespace std;
class Course{
private:
string name;
string *studentList;
int size, capacity=10;
public:
Course(){
name = "TBD";
size = 0;
studentList = new string[capacity];
}Course(string s){
name = s;
size = 0;
studentList = new string[capacity];
}void setName(string s){
name = s;
}getter/accessor for variable name
string getName(){
return name;
}
};
int main(){
Course a, b("Yankee");
cout << a.getName() << endl;
a.setName("Mets");
cout << a.getName() << endl;
cout << b.getName() << endl;
return 0;
}
this is the Question:: Continue with Team class:
a) Copy the previous program to a new file.
b) Implement the addMember, removeMember and display member functions to
Team class.
addMember(string) - add a new student with given name to the members.
removeMember() - delete the last added member from the members, no
deletion if there is no member in the members.
display() - display Team information.
Use following main() to test your class.
int main() {
Team a("Mets");
a.removeMember(); //
a.display();
a.addMember("David");
a.addMember("Steven");
a.addMember("Sara");
a.addMember("Tom");
a.display();
a.removeMember();
a.display();
a.removeMember();
a.display();
return 0;
}
Output from given main function:
No member to remove
Team name:Mets
Member List:
Team name:Mets
Member List: David Steven Sara Tom
Team name:Mets
Member List: David Steven Sara
Team name:Mets
Member List: David Steven

Step by step
Solved in 3 steps with 1 images

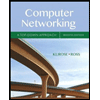
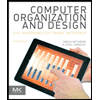
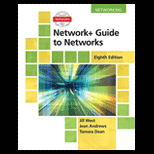
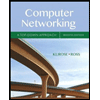
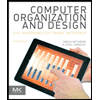
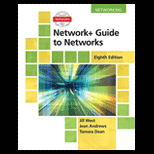
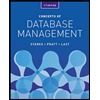
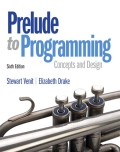
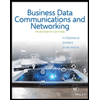