The template code must be incorporated into the following code after the template: // -- brief statement as to the file’s purpose //CSIS 111- ADD YOUR SECTION NUMBER // // //Include statements #include #include using namespace std; //Global declarations: Constants and type definitions only -- no variables //Function prototypes int main() { //In cout statement below SUBSTITUTE your name and lab number cout << "Your name -- Lab Number" << endl << endl; //Variable declarations //Program logic //Closing program statements system("pause"); return 0; } //Function definitions That template must be placed in with this code: #include //Program containing necessary header files #include // used for file read and write operation #include #include // used for mathtype calculations #include //used for precision using namespace std; // function is used to print the last name of the student in output void print_name(string lastName) { cout << lastName << " "; } // function prints remarks based on the percentage of student void print_remark(int per) { if (per > 90) { cout << "Excellent"; return; } if (per > 80 && per <= 90) { cout << "Well done"; return; } if (per > 70 && per <= 80) { cout << "Good"; return; } if (per >= 60 && per <= 70) { cout << "Need Improvement"; return; } if (per < 60) { cout << "Fail"; return; } } int main() { string filePath; cout << " Mention the filepath: "; cin >> filePath; // read data from the file ifstream inputFile(filePath); string lastName; int obtained, maximum; //every line of the file will have one string and two numbers and will be read token by token. while (inputFile >> lastName >> obtained >> maximum) { double per, per1; //calculate percentage per = (double)obtained / maximum; per1 = per * 100; print_name(lastName); // here instead of just using integer part of per1 'ceil' or 'floor' function can be used which gives you the 84(nearest upper integer) for .83333 and floor will get you 83(nearest lower integer) cout << (int)per1; cout << "% "; // std::fixed prints the trailing zeroes in decimal part eg:- 4.5000 // setprecision is the number of digits printed after decimal cout << std::fixed; cout << setprecision(5) << per << " "; // this function prints remarks based on percentage. print_remark((int)per1); cout << "\n"; } return 0; } I don't know how to incorporate both into one code without fixing the int main () error I get, as I am new to coding and don't know how to alleviate any issues.
The template code must be incorporated into the following code after the template:
// -- brief statement as to the file’s purpose
//CSIS 111- ADD YOUR SECTION NUMBER
//<Sources if necessary>
// //Include statements
#include <iostream>
#include <string>
using namespace std;
//Global declarations: Constants and type definitions only -- no variables
//Function prototypes
int main()
{
//In cout statement below SUBSTITUTE your name and lab number
cout << "Your name -- Lab Number" << endl << endl;
//Variable declarations
//
//Closing program statements
system("pause");
return 0;
}
//Function definitions
That template must be placed in with this code:
#include <iostream> //Program containing necessary header files
#include <fstream> // used for file read and write operation
#include <string>
#include <cmath> // used for mathtype calculations
#include <iomanip> //used for precision
using namespace std;
// function is used to print the last name of the student in output
void print_name(string lastName)
{
cout << lastName << " ";
}
// function prints remarks based on the percentage of student
void print_remark(int per)
{
if (per > 90)
{
cout << "Excellent";
return;
}
if (per > 80 && per <= 90)
{
cout << "Well done";
return;
}
if (per > 70 && per <= 80)
{
cout << "Good";
return;
}
if (per >= 60 && per <= 70)
{
cout << "Need Improvement";
return;
}
if (per < 60)
{
cout << "Fail";
return;
}
}
int main()
{
string filePath;
cout << " Mention the filepath: ";
cin >> filePath;
// read data from the file
ifstream inputFile(filePath);
string lastName;
int obtained, maximum;
//every line of the file will have one string and two numbers and will be read token by token.
while (inputFile >> lastName >> obtained >> maximum)
{
double per, per1;
//calculate percentage
per = (double)obtained / maximum;
per1 = per * 100;
print_name(lastName);
// here instead of just using integer part of per1 'ceil' or 'floor' function can be used which gives you the 84(nearest upper integer) for .83333 and floor will get you 83(nearest lower integer)
cout << (int)per1;
cout << "% ";
// std::fixed prints the trailing zeroes in decimal part eg:- 4.5000
// setprecision is the number of digits printed after decimal
cout << std::fixed;
cout << setprecision(5) << per << " ";
// this function prints remarks based on percentage.
print_remark((int)per1);
cout << "\n";
}
return 0;
}
I don't know how to incorporate both into one code without fixing the int main () error I get, as I am new to coding and don't know how to alleviate any issues.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

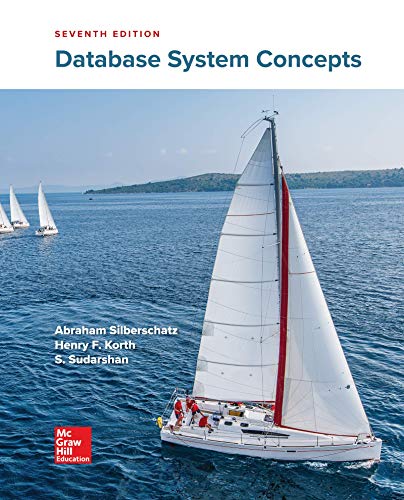
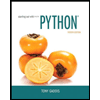
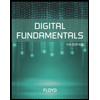
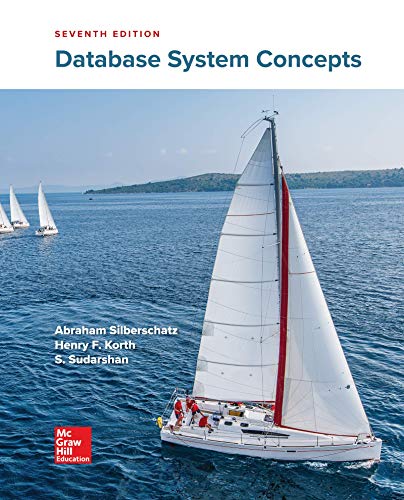
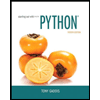
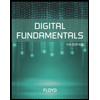
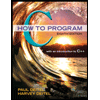
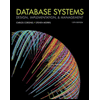
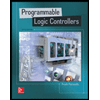