String inquiredItem and integer numData are read from input. Then, numData alphabetically sorted strings are read from input and each string is appended to a vector. Complete the FindMiddle() function: If inquiredItem is alphabetically before middleValue (the element at index middleIndex of the vector), output "Search lower half" and recursively call FindMiddle() to find inquiredItem in the lower half of the range. Otherwise, output "Search upper half" and recursively call FindMiddle() to find inquiredItem in the upper half of the range. End each output with a newline. Click here for example Ex: If the input is: ill 8 gif gum ill old pen pit pun ten then the output is: Search lower half Search upper half ill is found at index 2 Note: string1 < string2 returns true if string1 is alphabetically before string2, and returns false otherwise.You can only change the line "Your code goes here". '' #include <iostream>#include <vector>#include <string>using namespace std; void FindMiddle(vector<string> allWords, string inquiredItem, int startIndex, int endIndex) { int middleIndex; int rangeSize; string middleValue; rangeSize = (endIndex - startIndex) + 1; middleIndex = (startIndex + endIndex) / 2; middleValue = allWords.at(middleIndex); if (inquiredItem == middleValue) { cout << inquiredItem << " is found at index " << middleIndex << endl; } else if (rangeSize == 1) { cout << inquiredItem << " is not in the list" << endl; } else { /* Your code goes here */ }} int main() { string inquiredItem; vector<string> dataList; int numData; int i; string item; cin >> inquiredItem; cin >> numData; for (i = 0; i < numData; ++i) { cin >> item; dataList.push_back(item); } FindMiddle(dataList, inquiredItem, 0, dataList.size() - 1); return 0;}'''
String inquiredItem and integer numData are read from input. Then, numData alphabetically sorted strings are read from input and each string is appended to a vector. Complete the FindMiddle() function:
- If inquiredItem is alphabetically before middleValue (the element at index middleIndex of the vector), output "Search lower half" and recursively call FindMiddle() to find inquiredItem in the lower half of the range.
- Otherwise, output "Search upper half" and recursively call FindMiddle() to find inquiredItem in the upper half of the range.
End each output with a newline.
Click here for example Ex: If the input is:
ill 8 gif gum ill old pen pit pun ten
then the output is:
Search lower half Search upper half ill is found at index 2Note: string1 < string2 returns true if string1 is alphabetically before string2, and returns false otherwise.
You can only change the line "Your code goes here".
''
#include <iostream>
#include <vector>
#include <string>
using namespace std;
void FindMiddle(vector<string> allWords, string inquiredItem, int startIndex, int endIndex) {
int middleIndex;
int rangeSize;
string middleValue;
rangeSize = (endIndex - startIndex) + 1;
middleIndex = (startIndex + endIndex) / 2;
middleValue = allWords.at(middleIndex);
if (inquiredItem == middleValue) {
cout << inquiredItem << " is found at index " << middleIndex << endl;
}
else if (rangeSize == 1) {
cout << inquiredItem << " is not in the list" << endl;
}
else {
/* Your code goes here */
}
}
int main() {
string inquiredItem;
vector<string> dataList;
int numData;
int i;
string item;
cin >> inquiredItem;
cin >> numData;
for (i = 0; i < numData; ++i) {
cin >> item;
dataList.push_back(item);
}
FindMiddle(dataList, inquiredItem, 0, dataList.size() - 1);
return 0;
}
'''

Step by step
Solved in 2 steps

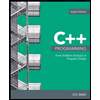
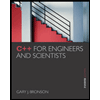
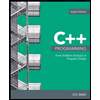
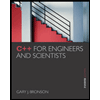