Part 3. Create a test class "TestPolymorphism.java" in the same package. Inside its main() method do the following steps. Some steps ask to provide explanantions for questions. Provide the answers in a separate PDF file. a) Create two Fruit objects "fruit1" and "fruit2" using the two constructors respectively. b) Display the total number of fruits using the getNumberOfFruits() method. c) Create a new Apple object "apple1" using the no-arg constructor and assign it to the object reference variable of type Fruit. (i.e., Apple as a subtype of Fruit)
Please complete and check my code in Java (eclipse). Complete the instructions (please see images). Please add comments.
package HW5;
enum Color {
YELLOW, ORANGE, RED, PURPLE;
}
public class Fruit{
private Color color;
private int calories;
private double pricePerPound;
protected static int numberOfFruits;
//Constructor a default fruit object
Fruit(){
numberOfFruits++;
}
Fruit(Color color, int calories, double pricePerPound){
this.color=color;
this.calories=calories;
this.pricePerPound= pricePerPound;
numberOfFruits++;
}
/////generate getters & setters
public Color getColor() {
return color;
}
public void setColor(Color color) {
this.color = color;
}
public int getCalories() {
return calories;
}
public void setCalories(int calories) {
this.calories = calories;
}
public double getPricePerPound() {
return pricePerPound;
}
public void setPricePerPound(double pricePerPound) {
this.pricePerPound = pricePerPound;
}
public static int getNumberOfFruits() {
return numberOfFruits;
}
public static void setNumberOfFruits(int numberOfFruits) {
Fruit.numberOfFruits = numberOfFruits;
}
@Override
public String toString() {
return "\ncolor: "+ color + "Calories: "+ calories + "pricePerPound: "+ pricePerPound;
}
}
package HW5;
//Child class #1
public class Apple extends Fruit {
private String variety;
private String texture;
//default constructor
Apple(){
super();
}
Apple(String variety, String texture, Color color, int calories, double pricePerPound){
super (color,calories, pricePerPound);
this.variety=variety;
this.texture=texture;
}
/////generate getters & setters
public String getVariety() {
return variety;
}
public void setVariety(String variety) {
this.variety = variety;
}
public String getTexture() {
return texture;
}
public void setTexture(String texture) {
this.texture = texture;
}
@Override
public String toString() {
return "\ncolor: "+ getColor() + "Calories: "+ getCalories() + "pricePerPound: "+ getPricePerPound()+
"\nvariety: "+ getVariety() +"\ntexture: "+ getTexture();
}
}
package HW5;
//Child class #2
public class Grapes extends Fruit {
private String taste;
private boolean hasSeed;
//default constructor
Grapes(){
super();
}
Grapes( String taste, boolean hasSeed, Color color, int calories, double pricePerPound){
super (color,calories, pricePerPound);
this.taste=taste;
this.hasSeed=hasSeed;
}
////////generate getters & setters
public String getTaste() {
return taste;
}
public void setTaste(String taste) {
this.taste = taste;
}
public boolean isHasSeed() {
return hasSeed;
}
public void setHasSeed(boolean hasSeed) {
this.hasSeed = hasSeed;
}
@Override
public String toString() {
return "\ntaste: "+ getTaste() +"\ncolor: "+ getColor() +
"Calories: "+ getCalories() + "pricePerPound: "+
getPricePerPound();
}
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

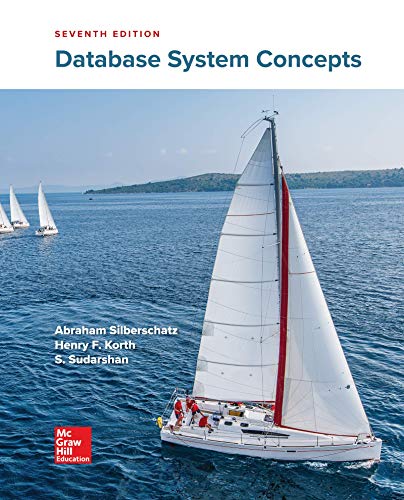
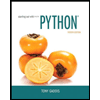
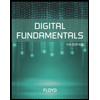
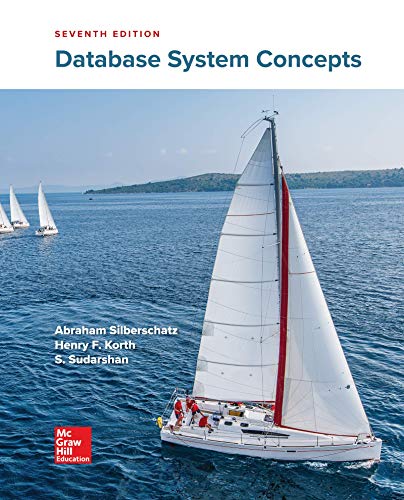
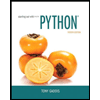
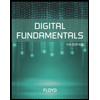
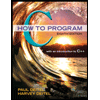
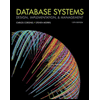
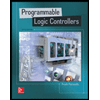