Modify to do the following: Add data integrity handing using cin.ignore, etc. for the initial inputs #include #include #include #include #include using namespace std; class StudentRec { private: string last_name = ""; // Last name string first_name = ""; // First name int year_grad = 0; // Year expected to graduate float gpa = 0.0; // Current gpa public: void set_last_name(string last_name_param); void set_first_name(string first_name_param); void set_gpa(float gpa = 0.0); string get_last_name() const; string get_first_name()const; string get_last_name_upper() const; string get_first_name_upper() const; void set_year_grad(int year_grad_param); int get_year_grad() const; int getgpa()const; bool equals(const StudentRec&); string to_str() const; // the rest of the "setter" and "getter" functions for each variable above go here }; void StudentRec::set_last_name(string last_name_param) { last_name = last_name_param; } void StudentRec::set_first_name(string first_name_param) { first_name = first_name_param; } void StudentRec::set_gpa(float gpa_param) { gpa = gpa_param; } string StudentRec::get_last_name() const { return last_name; } string StudentRec::get_first_name() const { return first_name; } string StudentRec::get_last_name_upper() const { string last_name_upper; for (char c : last_name) { last_name_upper.push_back(toupper(c)); } return last_name_upper; } string StudentRec::get_first_name_upper() const { string first_name_upper; for (char c : first_name) { first_name_upper.push_back(toupper(c)); } return first_name_upper; } void StudentRec::set_year_grad(int year_param) { if (year_param < 1888) { throw invalid_argument("Year must be 1888 or later."); } year_grad = year_param; } int StudentRec::get_year_grad() const { return year_grad; } bool StudentRec::equals(const StudentRec& to_compare) { return (last_name == to_compare.last_name && year_grad == to_compare.year_grad); } string StudentRec::to_str()const { return last_name + " (" + to_string(year_grad) + ')'; } int StudentRec::getgpa() const { return gpa; } int main() { float avggpa = 0; cout << "The Student List Program\n\n"; cout << "Enter a Student Record \n\n"; // Get vector of StudentRec objects vector student_list; char another = 'y'; while (tolower(another) == 'y') { StudentRec StudentRec; // make temporary new (initialized) StudentRec object string first_name; cout << "First Name: "; getline(cin, first_name); StudentRec.set_first_name(first_name); string last_name; cout << "Last name: "; getline(cin, last_name); StudentRec.set_last_name(last_name); int year_grad; cout << "Grad Year: "; cin >> year_grad; try { StudentRec.set_year_grad(year_grad); } catch (const invalid_argument& e) { cout << e.what() << "\n"; cout << "Exiting Program...\n\n"; return 0; } StudentRec.set_year_grad(year_grad); float gpa; cout << "GPA: "; cin >> gpa; StudentRec.set_gpa(gpa); student_list.push_back(StudentRec); cout << "\nEnter another Student Record? (y/n): "; cin >> another; cin.ignore(); // Only one character should be extracted; the others should be ignored (flush the buffer) cout << endl; } // display the list vector avg; for (StudentRec temp : student_list) { avg.push_back(temp.getgpa()); } for (int x = 0; x < avg.size(); x++) { avggpa += avg.at(x); } avggpa = (avggpa) / (avg.size()); // Use to find the average gpa of the students const int w = 5; cout << left << setw(w * 3) << "First Name" << setw(w * 3) << "Last Name" << setw(w * 3) << "Grad Year" << setw(w * 3) << "GPA" << endl; cout << endl; for (StudentRec StudentRec : student_list) { cout << setw(w * 3) << StudentRec.get_first_name() << setw(w * 3) << StudentRec.get_last_name() << setw(w * 3) << StudentRec.get_year_grad() << setw(w * 3) << StudentRec.getgpa() << endl; } cout << endl; cout << endl; // Output with titles in ALL CAPS for (StudentRec StudentRec : student_list) { cout << setw(w * 3) << StudentRec.get_first_name_upper() << setw(w * 3) << StudentRec.get_last_name_upper() << setw(w * 3) << StudentRec.get_year_grad() << setw(w * 3) << StudentRec.getgpa() << endl; } cout << endl; cout << "Average gpa for the students is " << fixed << setprecision(2) << avggpa << endl; return 0; }
Modify to do the following:
Add data integrity handing using cin.ignore, etc. for the initial inputs
#include <iostream>
#include <iomanip>
#include <string>
#include <
#include <cmath>
using namespace std;
class StudentRec
{
private:
string last_name = ""; // Last name
string first_name = ""; // First name
int year_grad = 0; // Year expected to graduate
float gpa = 0.0; // Current gpa
public:
void set_last_name(string last_name_param);
void set_first_name(string first_name_param);
void set_gpa(float gpa = 0.0);
string get_last_name() const;
string get_first_name()const;
string get_last_name_upper() const;
string get_first_name_upper() const;
void set_year_grad(int year_grad_param);
int get_year_grad() const;
int getgpa()const;
bool equals(const StudentRec&);
string to_str() const;
// the rest of the "setter" and "getter" functions for each variable above go here
};
void StudentRec::set_last_name(string last_name_param)
{
last_name = last_name_param;
}
void StudentRec::set_first_name(string first_name_param)
{
first_name = first_name_param;
}
void StudentRec::set_gpa(float gpa_param)
{
gpa = gpa_param;
}
string StudentRec::get_last_name() const
{
return last_name;
}
string StudentRec::get_first_name() const
{
return first_name;
}
string StudentRec::get_last_name_upper() const
{
string last_name_upper;
for (char c : last_name) {
last_name_upper.push_back(toupper(c));
}
return last_name_upper;
}
string StudentRec::get_first_name_upper() const
{
string first_name_upper;
for (char c : first_name) {
first_name_upper.push_back(toupper(c));
}
return first_name_upper;
}
void StudentRec::set_year_grad(int year_param)
{
if (year_param < 1888)
{
throw invalid_argument("Year must be 1888 or later.");
}
year_grad = year_param;
}
int StudentRec::get_year_grad() const
{
return year_grad;
}
bool StudentRec::equals(const StudentRec& to_compare)
{
return (last_name == to_compare.last_name && year_grad == to_compare.year_grad);
}
string StudentRec::to_str()const
{
return last_name + " (" + to_string(year_grad) + ')';
}
int StudentRec::getgpa() const
{
return gpa;
}
int main()
{
float avggpa = 0;
cout << "The Student List Program\n\n";
cout << "Enter a Student Record \n\n"; // Get vector of StudentRec objects
vector<StudentRec> student_list;
char another = 'y';
while (tolower(another) == 'y')
{
StudentRec StudentRec; // make temporary new (initialized) StudentRec object
string first_name;
cout << "First Name: ";
getline(cin, first_name);
StudentRec.set_first_name(first_name);
string last_name;
cout << "Last name: ";
getline(cin, last_name);
StudentRec.set_last_name(last_name);
int year_grad;
cout << "Grad Year: ";
cin >> year_grad;
try {
StudentRec.set_year_grad(year_grad);
}
catch (const invalid_argument& e)
{
cout << e.what() << "\n";
cout << "Exiting Program...\n\n";
return 0;
}
StudentRec.set_year_grad(year_grad);
float gpa;
cout << "GPA: ";
cin >> gpa;
StudentRec.set_gpa(gpa);
student_list.push_back(StudentRec);
cout << "\nEnter another Student Record? (y/n): ";
cin >> another;
cin.ignore(); // Only one character should be extracted; the others should be ignored (flush the buffer)
cout << endl;
}
// display the list
vector<double> avg;
for (StudentRec temp : student_list)
{
avg.push_back(temp.getgpa());
}
for (int x = 0; x < avg.size(); x++)
{
avggpa += avg.at(x);
}
avggpa = (avggpa) / (avg.size()); // Use to find the average gpa of the students
const int w = 5;
cout << left << setw(w * 3) << "First Name" << setw(w * 3) << "Last Name" << setw(w * 3) << "Grad Year" << setw(w * 3) << "GPA" << endl;
cout << endl;
for (StudentRec StudentRec : student_list)
{
cout << setw(w * 3) << StudentRec.get_first_name()
<< setw(w * 3) << StudentRec.get_last_name() << setw(w * 3) << StudentRec.get_year_grad() << setw(w * 3) << StudentRec.getgpa() << endl;
}
cout << endl;
cout << endl;
// Output with titles in ALL CAPS
for (StudentRec StudentRec : student_list)
{
cout << setw(w * 3) << StudentRec.get_first_name_upper()
<< setw(w * 3) << StudentRec.get_last_name_upper() << setw(w * 3) << StudentRec.get_year_grad() << setw(w * 3) << StudentRec.getgpa() << endl;
}
cout << endl;
cout << "Average gpa for the students is " << fixed << setprecision(2) << avggpa << endl;
return 0;
}

Step by step
Solved in 2 steps

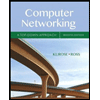
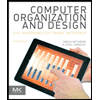
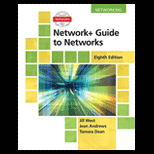
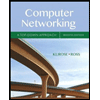
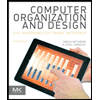
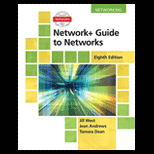
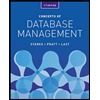
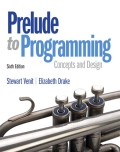
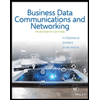