main.cc file #include #include #include "train.h" int main() { // Creates a train, with the locomotive at the front of the train. // LinkedList diagram: // Locomotive -> First Class -> Business Class -> Cafe Car -> Carriage 1 -> // Carriage 2 std::shared_ptr carriage2 = std::make_shared(100, 100, nullptr); std::shared_ptr carriage1 = std::make_shared(220, 220, carriage2); std::shared_ptr cafe_car = std::make_shared(250, 250, carriage1); std::shared_ptr business_class = std::make_shared(50, 50, cafe_car); std::shared_ptr first_class = std::make_shared(20, 20, business_class); std::shared_ptr locomotive = std::make_shared(1, 1, first_class); std::cout << "Total passengers in the train: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of passengers in the // train by invoking TotalTrainPassengers on the // first carriage in the train (the locomotive). // ====================================================== std::cout << std::endl; std::cout << "Max passengers in a carriage: "; // =================== YOUR CODE HERE =================== // 2. Print out the maximum number of passengers in a // single carriage in the train by invoking // MaxCarriagePassengers. // ====================================================== std::cout << std::endl; // =================== YOUR CODE HERE =================== // 3. Using IsTrainFull, check if the locomotive is full. // If IsTrainFull returns true, print // "The train is full." // If IsTrainFull returns false, print // "The train has available seats." // ====================================================== std::cout << std::endl; // =================== YOUR CODE HERE =================== // 4. Create a new std::shared_ptr. You may name // it the `caboose`, which refers to the carriage at // the end of a train. // Set its passenger count to 40, seat count to // 75, and next carriage to `nullptr`. // ====================================================== std::cout << "Adding a new carriage to the train!" << std::endl; // =================== YOUR CODE HERE =================== // 5. Using `AddCarriageToEnd`, add the new Train you've // created in #4 to the end of the `locomotive`. // ====================================================== // =================== YOUR CODE HERE =================== // 6. Using IsTrainFull, check if the locomotive is full. // If IsTrainFull returns true, print // "The train is now full." // If IsTrainFull returns false, print // "The train now has available seats." // ====================================================== std::cout << std::endl; return 0; } train.cc file #include "train.h" // ========================= YOUR CODE HERE ========================= // This implementation file (train.cc) is where you should implement // the member functions declared in the header (train.h), only // if you didn't implement them inline within train.h. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Train class. // =================================================================== train.h file #include #include class Train { public: Train(int passenger_count, int seat_count, std::shared_ptr next_carriage) : passenger_count_(passenger_count), seat_count_(seat_count), next_carriage_(next_carriage) {} int GetPassengerCount() const { return passenger_count_; } int GetSeatCount() const { return seat_count_; } std::shared_ptr GetNextCarriage() const { return next_carriage_; } // ====================== YOUR CODE HERE ====================== // Define the following recursive member functions, according // to the instructions in the README: // // 1. TotalTrainPassengers // 2. MaxCarriagePassengers // 3. IsTrainFull // 4. AddCarriageToEnd // ============================================================ private: int passenger_count_; int seat_count_; std::shared_ptr next_carriage_; }; Output: Total passengers in the train: 641 Max passengers in a carriage: 250 The train is full. Adding a new carriage to the train! The train now has available seats.
main.cc file
#include <iostream>
#include <memory>
#include "train.h"
int main() {
// Creates a train, with the locomotive at the front of the train.
// LinkedList diagram:
// Locomotive -> First Class -> Business Class -> Cafe Car -> Carriage 1 ->
// Carriage 2
std::shared_ptr<Train> carriage2 = std::make_shared<Train>(100, 100, nullptr);
std::shared_ptr<Train> carriage1 =
std::make_shared<Train>(220, 220, carriage2);
std::shared_ptr<Train> cafe_car =
std::make_shared<Train>(250, 250, carriage1);
std::shared_ptr<Train> business_class =
std::make_shared<Train>(50, 50, cafe_car);
std::shared_ptr<Train> first_class =
std::make_shared<Train>(20, 20, business_class);
std::shared_ptr<Train> locomotive =
std::make_shared<Train>(1, 1, first_class);
std::cout << "Total passengers in the train: ";
// =================== YOUR CODE HERE ===================
// 1. Print out the total number of passengers in the
// train by invoking TotalTrainPassengers on the
// first carriage in the train (the locomotive).
// ======================================================
std::cout << std::endl;
std::cout << "Max passengers in a carriage: ";
// =================== YOUR CODE HERE ===================
// 2. Print out the maximum number of passengers in a
// single carriage in the train by invoking
// MaxCarriagePassengers.
// ======================================================
std::cout << std::endl;
// =================== YOUR CODE HERE ===================
// 3. Using IsTrainFull, check if the locomotive is full.
// If IsTrainFull returns true, print
// "The train is full."
// If IsTrainFull returns false, print
// "The train has available seats."
// ======================================================
std::cout << std::endl;
// =================== YOUR CODE HERE ===================
// 4. Create a new std::shared_ptr<Train>. You may name
// it the `caboose`, which refers to the carriage at
// the end of a train.
// Set its passenger count to 40, seat count to
// 75, and next carriage to `nullptr`.
// ======================================================
std::cout << "Adding a new carriage to the train!" << std::endl;
// =================== YOUR CODE HERE ===================
// 5. Using `AddCarriageToEnd`, add the new Train you've
// created in #4 to the end of the `locomotive`.
// ======================================================
// =================== YOUR CODE HERE ===================
// 6. Using IsTrainFull, check if the locomotive is full.
// If IsTrainFull returns true, print
// "The train is now full."
// If IsTrainFull returns false, print
// "The train now has available seats."
// ======================================================
std::cout << std::endl;
return 0;
}
train.cc file
#include "train.h"
// ========================= YOUR CODE HERE =========================
// This implementation file (train.cc) is where you should implement
// the member functions declared in the header (train.h), only
// if you didn't implement them inline within train.h.
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the Train class.
// ===================================================================
train.h file
#include <memory>
#include <string>
class Train {
public:
Train(int passenger_count, int seat_count,
std::shared_ptr<Train> next_carriage)
: passenger_count_(passenger_count),
seat_count_(seat_count),
next_carriage_(next_carriage) {}
int GetPassengerCount() const {
return passenger_count_;
}
int GetSeatCount() const {
return seat_count_;
}
std::shared_ptr<Train> GetNextCarriage() const {
return next_carriage_;
}
// ====================== YOUR CODE HERE ======================
// Define the following recursive member functions, according
// to the instructions in the README:
//
// 1. TotalTrainPassengers
// 2. MaxCarriagePassengers
// 3. IsTrainFull
// 4. AddCarriageToEnd
// ============================================================
private:
int passenger_count_;
int seat_count_;
std::shared_ptr<Train> next_carriage_;
};
Output:
Total passengers in the train: 641
Max passengers in a carriage: 250
The train is full.
Adding a new carriage to the train!
The train now has available seats.


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

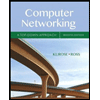
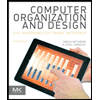
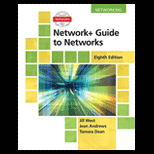
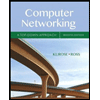
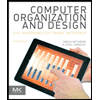
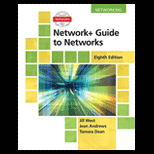
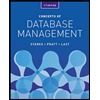
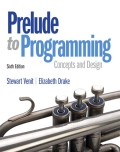
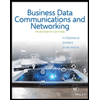