Overview In this assignment you will define a class called NumCouple that will store two integers (num1 and num2) and have a variety of functions for working with them. You will then define a class called NumThruple which inherits from NumCouple and has an additional integer num3. NumThruple will support all the same functions as NumCouple and it will reuse the NumCouple code to supply answers. Cut and paste my hard-coded calls that will create several instances of User and call their functions. There is no user input on this assignment. Expected Output Example 1 The couples minimum is 4. The couples maximum is 7 The couples product is 28 The thruples minimum is 2 The thruples maximum is 5 The thruples product is 30 Specifications • You should submit a single file called M7A4.py • It should follow the submission standards outlined here: Submission Standards • Define a class call NumCouple with Integer attributes num1 and num2 • A function minimum()) that will return the minimum of num1 and num2 • A function maximum ()) that will return the maximum of num1 and num2 • A function product() that will return num1* num2 • Define a class called NumThruple that inherits from NumCouple and • Calls the __init___ method of NumCouple • Has an aditional integer named num3 • A function minimum()) that will return the minimum of num1, • A function maximum()) that will return the maximum of num1, • A function product()) that will return the product of num1, num2) and num3 and uses a call to NumCouples (minimum () function to do it. num2 and num3 and uses a call to NumCouples maximum() function to do it. num2 and num3 and uses a call to NumCouples product() function to do it.
Overview In this assignment you will define a class called NumCouple that will store two integers (num1 and num2) and have a variety of functions for working with them. You will then define a class called NumThruple which inherits from NumCouple and has an additional integer num3. NumThruple will support all the same functions as NumCouple and it will reuse the NumCouple code to supply answers. Cut and paste my hard-coded calls that will create several instances of User and call their functions. There is no user input on this assignment. Expected Output Example 1 The couples minimum is 4. The couples maximum is 7 The couples product is 28 The thruples minimum is 2 The thruples maximum is 5 The thruples product is 30 Specifications • You should submit a single file called M7A4.py • It should follow the submission standards outlined here: Submission Standards • Define a class call NumCouple with Integer attributes num1 and num2 • A function minimum()) that will return the minimum of num1 and num2 • A function maximum ()) that will return the maximum of num1 and num2 • A function product() that will return num1* num2 • Define a class called NumThruple that inherits from NumCouple and • Calls the __init___ method of NumCouple • Has an aditional integer named num3 • A function minimum()) that will return the minimum of num1, • A function maximum()) that will return the maximum of num1, • A function product()) that will return the product of num1, num2) and num3 and uses a call to NumCouples (minimum () function to do it. num2 and num3 and uses a call to NumCouples maximum() function to do it. num2 and num3 and uses a call to NumCouples product() function to do it.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
# hard-coded calls
# hard-coded lines
couple = NumCouple(4, 7)
print(f"The couples minimum is {couple.minimum()}")
print(f"The couples maximum is {couple.maximum()}")
print(f"The couples product is {couple.product()}")
thruple = NumThruple(3, 5, 2)
print(f"The thruples minimum is {thruple.minimum()}")
print(f"The thruples maximum is {thruple.maximum()}")
print(f"The thruples product is {thruple.product()}")

Transcribed Image Text:Overview
In this assignment you will define a class called NumCouple that will store two integers (num1 and num2) and have a variety of functions for working with them. You will then define a class called NumThruple which inherits
from NumCouple and has an additional integer num3. NumThruple will support all the same functions as NumCouple and it will reuse the NumCouple code to supply answers.
Cut and paste my hard-coded calls that will create several instances of User and call their functions. There is no user input on this assignment.
Expected Output
Example 1
The couples minimum is 4.
The couples maximum is 7
The couples product is 28
The thruples minimum is 2
The thruples maximum is 5
The thruples product is 30
Specifications
• You should submit a single file called M7A4.py
• It should follow the submission standards outlined here: Submission Standards
• Define a class call NumCouple with
• Integer attributes num1 and num2
• A function minimum()) that will return the minimum of (num1 and num2
• A function maximum ()) that will return the maximum of num1 and num2
• A function product() that will return num1* num2
• Define a class called NumThruple that inherits from NumCouple and
• Calls the __init___ method of NumCouple
• Has an aditional integer named num3
• A function minimum()) that will return the minimum of num1,
• A function maximum()) that will return the maximum of num1,
• A function product() that will return the product of num1,
• Your program must end with the hard-coded code below
num2 and num3 and uses a call to NumCouples minimum() function to do it.
num2 and num3 and uses a call to NumCouples maximum() function to do it.
num2 and num3 and uses a call to NumCouples product() function to do it.

Transcribed Image Text:Tips and Tricks
Be mindful of PyCharms autocomplete, there is a defined__int__ function that is not the same as your__init__
Hard-Coded
Copy/paste the code below into your M7A4.py document, after your class definition.
# hard-coded calls
#hard-coded lines.
couple = NumCouple(4, 7)
print (f"The couples minimum is {couple.minimum()}")
print (f"The couples maximum is {couple.maximum()}")
print (f"The couples product is {couple.product()}")
thruple = NumThruple(3, 5, 2)
print (f"The thruples minimum is {thruple.minimum()}")
print (f"The thruples maximum is (thruple.maximum()}")
print (f"The thruples product is {thruple.product()}")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
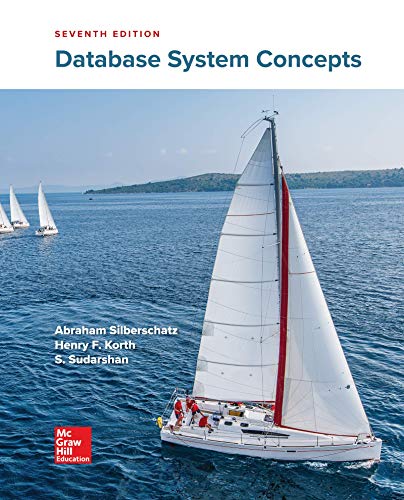
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
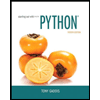
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
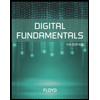
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
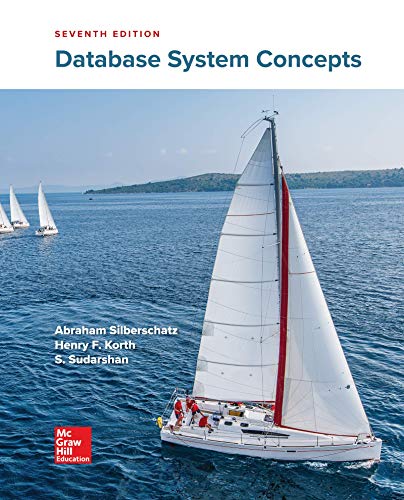
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
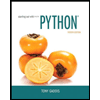
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
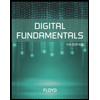
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
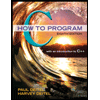
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
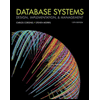
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
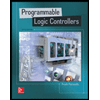
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education