Can you fix the code please on the first picture shows the error output. // Corrected code #define _CRT_SECURE_NO_WARNINGS #include "LibraryManagement.h" #include "Books.h" #include "DigitalMedia.h" #include "LibraryConfig.h" #include #include #include #include // Include the necessary header for boolean data type // Comparison function for qsort to sort Digital Media by ID int compareDigitalMedia(const void* a, const void* b) { return ((struct DigitalMedia*)a)->id - ((struct DigitalMedia*)b)->id; } // initializing library struct Library initializeLibrary() { struct Library lib; lib.bookCount = 0; lib.ebookCount = 0; lib.digitalMediaCount = 0; // Initialize book array for (int i = 0; i < MAX_BOOK_COUNT; i++) { lib.books[i].commonAttributes.id = -1; // Set an invalid ID to mark empty slot } // Initialize ebook array for (int i = 0; i < MAX_EBOOK_COUNT; i++) { lib.ebooks[i].commonAttributes.id = -1; } // Initialize digital media array for (int i = 0; i < MAX_DIGITAL_MEDIA_COUNT; i++) { lib.digitalMedia[i].id = -1; } return lib; } void loadBooks(struct Library* lib, const char* filename) { FILE* file = fopen(filename, "r"); if (file == NULL) { perror("Error opening the file"); return; } char line[2048]; while (fgets(line, sizeof(line), file) != NULL) { if (lib->bookCount >= MAX_BOOK_COUNT) { printf("Reached the maximum book limit. Skipping the rest.\n"); break; } struct Book tempBook; int readCount = sscanf(line, "%d;%127[^;];%127[^;];%127[^;];%d;%127[^;];%127[^;];%d;%127[^;];%d;%9[^;]", &tempBook.commonAttributes.id, tempBook.commonAttributes.title, tempBook.commonAttributes.author, tempBook.commonAttributes.genre, &tempBook.commonAttributes.yearOfPublication, tempBook.commonAttributes.publisher, tempBook.commonAttributes.isbn, &tempBook.numberOfPages, tempBook.condition, &tempBook.numberOfCopies, tempBook.shelfLocation); if (readCount == 11) { strncpy(tempBook.commonAttributes.isbn, tempBook.commonAttributes.isbn, 13); tempBook.commonAttributes.isbn[13] = '\0'; lib->books[lib->bookCount] = tempBook; lib->bookCount++; } else { printf("Failed to parse line: %s", line); } } fclose(file); } // load digital media void loadDigitalMedia(struct Library* lib, const char* filename) { FILE* file = fopen(filename, "r"); if (file == NULL) { perror("Error opening the file"); return; } char line[2048]; int lineCount = 0; while (fgets(line, sizeof(line), file) != NULL) { lineCount++; if (lib->digitalMediaCount >= MAX_DIGITAL_MEDIA_COUNT) { printf("Reached the maximum digital media limit. Skipping the rest.\n"); break; } struct DigitalMedia tempDigitalMedia; int readCount = sscanf(line, "%d;%2047[^;];%2047[^;];%2047[^;];%d;%2047[^;];%2047[^;];%d;%lf;%2047[^;];%d", &tempDigitalMedia.id, tempDigitalMedia.title, tempDigitalMedia.creator, tempDigitalMedia.genre, &tempDigitalMedia.yearOfRelease, tempDigitalMedia.format, &tempDigitalMedia.fileSize, tempDigitalMedia.accessLink, &tempDigitalMedia.downloadCount); if (readCount == 10) { // Check for a valid year of release within the range (1800 - 2023) if (tempDigitalMedia.yearOfRelease < 1800 || tempDigitalMedia.yearOfRelease > 2023) { printf("Skipping digital media with an invalid year of release at line %d: %s\n", lineCount, tempDigitalMedia.title); continue; } lib->digitalMedia[lib->digitalMediaCount] = tempDigitalMedia; lib->digitalMediaCount++; } else { printf("Failed to parse line %d: %s", lineCount, line); } } fclose(file); } // load ebooks void loadEbooks(struct Library* lib, const char* filename) { FILE* file = fopen(filename, "r"); if (file == NULL) { perror("Error opening the file"); return; } char line[2048]; while (fgets(line, sizeof(line), file) != NULL) { if (lib->ebookCount >= MAX_EBOOK_COUNT) { printf("Reached the maximum eBook limit. Skipping the rest.\n"); break; } struct EBook tempEBook; int readCount = sscanf(line, "%d;%50[^;];%50[^;];%50[^;];%d;%50[^;];%20[^;];%d", &tempEBook.commonAttributes.id, tempEBook.commonAttributes.title, tempEBook.commonAttributes.author, tempEBook.commonAttributes.genre, &tempEBook.commonAttributes.yearOfPublication, tempEBook.commonAttributes.publisher, tempEBook.commonAttributes.isbn); if (readCount == 7) { // Extract only the first 14 digits of ISBN strncpy(tempEBook.commonAttributes.isbn, tempEBook.commonAttributes.isbn, 13); tempEBook.commonAttributes.isbn[13] = '\0'; // Check for a valid publication year within the range (1800 - 2023) if (tempEBook.commonAttributes.yearOfPublication < 1800 || tempEBook.commonAttributes.yearOfPublication > 2023) { printf("Skipping eBook with an invalid publication year: %s\n", tempEBook.commonAttributes.title); continue; } lib->ebooks[lib->ebookCount] = tempEBook; lib->ebookCount++; } else { printf("Failed to parse line: %s", line); } } fclose(file); } // display books void displayBooks(const struct Library* lib) { // Check for valid library and book data if (lib == NULL || lib->books == NULL || lib->bookCount == 0) { printf("No books to display.\n"); return; } // Header printing printf("\n------- BOOKS -------\n"); printf("ID Title Author Genre Year Publisher ISBN Shelf\n\n"); printf("------------------------------------------------------------------------------------------------------------------------\n\n"); // Content display for (int i = 0; i < lib->bookCount; i++) { // Ensure that strings are null-terminated char title[MAX_TITLE_LEN + 1]; char author[MAX_AUTHOR_LEN + 1]; char genre[MAX_GENRE_LEN + 1]; char publisher[MAX_PUBLISHER_LEN + 1]; char isbn[MAX_ISBN_LEN + 1]; strncpy(title, lib->books[i].commonAttributes.title, MAX_TITLE_LEN); strncpy(author, lib->books[i].commonAttributes.author, MAX_AUTHOR_LEN); strncpy(genre, lib->books[i].commonAttributes.genre, MAX_GENRE_LEN); strncpy(publisher, lib->books[i].commonAttributes.publisher, MAX_PUBLISHER_LEN); strncpy(isbn, lib->books[i].commonAttributes.isbn, MAX_ISBN_LEN); title[MAX_TITLE_LEN] = '\0'; author[MAX_AUTHOR_LEN] = '\0'; genre[MAX_GENRE_LEN] = '\0'; publisher[MAX_PUBLISHER_LEN] = '\0'; isbn[MAX_ISBN_LEN] = '\0'; // Print book details printf("%-6d%-30.29s%-24.23s%-14.13s%-6d%-20.19s%-17.13s%-5s\n", lib->books[i].commonAttributes.id, title, author, genre, lib->books[i].commonAttributes.yearOfPublication, publisher, isbn, lib->books[i].shelfLocation); } } // display ebooks void displayEbooks(const struct Library* lib, int flag) { if (lib == NULL || lib->ebooks == NULL || lib->ebookCount == 0) { printf("No eBooks to display.\n"); return; } int start = (flag == 0) ? 0 : (lib->ebookCount < 5) ? 0 : lib->ebookCount - 5; int end = (flag == 0) ? ((lib->ebookCount < 5) ? lib->ebookCount : 5) : lib->ebookCount; printf("------- %s EBOOKS -------\n", (flag == 0) ? "First Five" : "Last Five"); printf("ID Title Author Genre Year Publisher ISBN Access Link Downloads\n"); printf("-----------------------------------------------------------------------------------------------------------------------------------------------------------\n"); for (int i = start; i < end; i++) { printf("%-6d%-30.29s%-24.19s%-14.11s%-6d%-20.19s%-20s%-7d\n", lib->ebooks[i].commonAttributes.id, lib->ebooks[i].commonAttributes.title, lib->ebooks[i].commonAttributes.author, lib->ebooks[i].commonAttributes.genre, lib->ebooks[i].commonAttributes.yearOfPublication, lib->ebooks[i].commonAttributes.publisher, lib->ebooks[i].commonAttributes.isbn, lib->ebooks[i].downloadCount); } } // display digital media void displayDigitalMedia(const struct Library* lib) { if (lib == NULL || lib->digitalMedia == NULL || lib->digitalMediaCount == 0) { printf("No digital media to display.\n"); return; } printf("------- DIGITAL MEDIA -------\n"); printf("ID Title Creator Genre Year Format Size Access Link Downloads\n"); printf("---------------------------------------------------------------------------------------------------------------------------------------------------------------\n"); // Display all digital media items for (int i = 0; i < lib->digitalMediaCount; i++) { printf("%-6d%-30.29s%-21.20s%-16.15s%-6d%-20.19s%-20s%-22.21s%-10d\n", lib->digitalMedia[i].id, lib->digitalMedia[i].title, lib->digitalMedia[i].creator, lib->digitalMedia[i].genre, lib->digitalMedia[i].yearOfRelease, lib->digitalMedia[i].format, lib->digitalMedia[i].fileSize, lib->digitalMedia[i].accessLink, lib->digitalMedia[i].downloadCount); } } // print histogram void printBookPublicationHistogram(const struct Library* lib) { if (lib == NULL || lib->books == NULL || lib->bookCount == 0) { printf("No books to display.\n"); return; } // Initialize an array to store the counts of books per year int bookYearCounts[NUM_YEARS] = { 0 }; // Count the books per year for (int i = 0; i < lib->bookCount; i++) { int year = lib->books[i].commonAttributes.yearOfPublication; if (year >= START_YEAR && year 0) { printf("%d: ", year + START_YEAR); for (int i = 0; i < bookYearCounts[year]; i++) { printf("*"); } printf(" (%d)\n", bookYearCounts[year]); } } }
Can you fix the code please on the first picture shows the error output. // Corrected code #define _CRT_SECURE_NO_WARNINGS #include "LibraryManagement.h" #include "Books.h" #include "DigitalMedia.h" #include "LibraryConfig.h" #include #include #include #include // Include the necessary header for boolean data type // Comparison function for qsort to sort Digital Media by ID int compareDigitalMedia(const void* a, const void* b) { return ((struct DigitalMedia*)a)->id - ((struct DigitalMedia*)b)->id; } // initializing library struct Library initializeLibrary() { struct Library lib; lib.bookCount = 0; lib.ebookCount = 0; lib.digitalMediaCount = 0; // Initialize book array for (int i = 0; i < MAX_BOOK_COUNT; i++) { lib.books[i].commonAttributes.id = -1; // Set an invalid ID to mark empty slot } // Initialize ebook array for (int i = 0; i < MAX_EBOOK_COUNT; i++) { lib.ebooks[i].commonAttributes.id = -1; } // Initialize digital media array for (int i = 0; i < MAX_DIGITAL_MEDIA_COUNT; i++) { lib.digitalMedia[i].id = -1; } return lib; } void loadBooks(struct Library* lib, const char* filename) { FILE* file = fopen(filename, "r"); if (file == NULL) { perror("Error opening the file"); return; } char line[2048]; while (fgets(line, sizeof(line), file) != NULL) { if (lib->bookCount >= MAX_BOOK_COUNT) { printf("Reached the maximum book limit. Skipping the rest.\n"); break; } struct Book tempBook; int readCount = sscanf(line, "%d;%127[^;];%127[^;];%127[^;];%d;%127[^;];%127[^;];%d;%127[^;];%d;%9[^;]", &tempBook.commonAttributes.id, tempBook.commonAttributes.title, tempBook.commonAttributes.author, tempBook.commonAttributes.genre, &tempBook.commonAttributes.yearOfPublication, tempBook.commonAttributes.publisher, tempBook.commonAttributes.isbn, &tempBook.numberOfPages, tempBook.condition, &tempBook.numberOfCopies, tempBook.shelfLocation); if (readCount == 11) { strncpy(tempBook.commonAttributes.isbn, tempBook.commonAttributes.isbn, 13); tempBook.commonAttributes.isbn[13] = '\0'; lib->books[lib->bookCount] = tempBook; lib->bookCount++; } else { printf("Failed to parse line: %s", line); } } fclose(file); } // load digital media void loadDigitalMedia(struct Library* lib, const char* filename) { FILE* file = fopen(filename, "r"); if (file == NULL) { perror("Error opening the file"); return; } char line[2048]; int lineCount = 0; while (fgets(line, sizeof(line), file) != NULL) { lineCount++; if (lib->digitalMediaCount >= MAX_DIGITAL_MEDIA_COUNT) { printf("Reached the maximum digital media limit. Skipping the rest.\n"); break; } struct DigitalMedia tempDigitalMedia; int readCount = sscanf(line, "%d;%2047[^;];%2047[^;];%2047[^;];%d;%2047[^;];%2047[^;];%d;%lf;%2047[^;];%d", &tempDigitalMedia.id, tempDigitalMedia.title, tempDigitalMedia.creator, tempDigitalMedia.genre, &tempDigitalMedia.yearOfRelease, tempDigitalMedia.format, &tempDigitalMedia.fileSize, tempDigitalMedia.accessLink, &tempDigitalMedia.downloadCount); if (readCount == 10) { // Check for a valid year of release within the range (1800 - 2023) if (tempDigitalMedia.yearOfRelease < 1800 || tempDigitalMedia.yearOfRelease > 2023) { printf("Skipping digital media with an invalid year of release at line %d: %s\n", lineCount, tempDigitalMedia.title); continue; } lib->digitalMedia[lib->digitalMediaCount] = tempDigitalMedia; lib->digitalMediaCount++; } else { printf("Failed to parse line %d: %s", lineCount, line); } } fclose(file); } // load ebooks void loadEbooks(struct Library* lib, const char* filename) { FILE* file = fopen(filename, "r"); if (file == NULL) { perror("Error opening the file"); return; } char line[2048]; while (fgets(line, sizeof(line), file) != NULL) { if (lib->ebookCount >= MAX_EBOOK_COUNT) { printf("Reached the maximum eBook limit. Skipping the rest.\n"); break; } struct EBook tempEBook; int readCount = sscanf(line, "%d;%50[^;];%50[^;];%50[^;];%d;%50[^;];%20[^;];%d", &tempEBook.commonAttributes.id, tempEBook.commonAttributes.title, tempEBook.commonAttributes.author, tempEBook.commonAttributes.genre, &tempEBook.commonAttributes.yearOfPublication, tempEBook.commonAttributes.publisher, tempEBook.commonAttributes.isbn); if (readCount == 7) { // Extract only the first 14 digits of ISBN strncpy(tempEBook.commonAttributes.isbn, tempEBook.commonAttributes.isbn, 13); tempEBook.commonAttributes.isbn[13] = '\0'; // Check for a valid publication year within the range (1800 - 2023) if (tempEBook.commonAttributes.yearOfPublication < 1800 || tempEBook.commonAttributes.yearOfPublication > 2023) { printf("Skipping eBook with an invalid publication year: %s\n", tempEBook.commonAttributes.title); continue; } lib->ebooks[lib->ebookCount] = tempEBook; lib->ebookCount++; } else { printf("Failed to parse line: %s", line); } } fclose(file); } // display books void displayBooks(const struct Library* lib) { // Check for valid library and book data if (lib == NULL || lib->books == NULL || lib->bookCount == 0) { printf("No books to display.\n"); return; } // Header printing printf("\n------- BOOKS -------\n"); printf("ID Title Author Genre Year Publisher ISBN Shelf\n\n"); printf("------------------------------------------------------------------------------------------------------------------------\n\n"); // Content display for (int i = 0; i < lib->bookCount; i++) { // Ensure that strings are null-terminated char title[MAX_TITLE_LEN + 1]; char author[MAX_AUTHOR_LEN + 1]; char genre[MAX_GENRE_LEN + 1]; char publisher[MAX_PUBLISHER_LEN + 1]; char isbn[MAX_ISBN_LEN + 1]; strncpy(title, lib->books[i].commonAttributes.title, MAX_TITLE_LEN); strncpy(author, lib->books[i].commonAttributes.author, MAX_AUTHOR_LEN); strncpy(genre, lib->books[i].commonAttributes.genre, MAX_GENRE_LEN); strncpy(publisher, lib->books[i].commonAttributes.publisher, MAX_PUBLISHER_LEN); strncpy(isbn, lib->books[i].commonAttributes.isbn, MAX_ISBN_LEN); title[MAX_TITLE_LEN] = '\0'; author[MAX_AUTHOR_LEN] = '\0'; genre[MAX_GENRE_LEN] = '\0'; publisher[MAX_PUBLISHER_LEN] = '\0'; isbn[MAX_ISBN_LEN] = '\0'; // Print book details printf("%-6d%-30.29s%-24.23s%-14.13s%-6d%-20.19s%-17.13s%-5s\n", lib->books[i].commonAttributes.id, title, author, genre, lib->books[i].commonAttributes.yearOfPublication, publisher, isbn, lib->books[i].shelfLocation); } } // display ebooks void displayEbooks(const struct Library* lib, int flag) { if (lib == NULL || lib->ebooks == NULL || lib->ebookCount == 0) { printf("No eBooks to display.\n"); return; } int start = (flag == 0) ? 0 : (lib->ebookCount < 5) ? 0 : lib->ebookCount - 5; int end = (flag == 0) ? ((lib->ebookCount < 5) ? lib->ebookCount : 5) : lib->ebookCount; printf("------- %s EBOOKS -------\n", (flag == 0) ? "First Five" : "Last Five"); printf("ID Title Author Genre Year Publisher ISBN Access Link Downloads\n"); printf("-----------------------------------------------------------------------------------------------------------------------------------------------------------\n"); for (int i = start; i < end; i++) { printf("%-6d%-30.29s%-24.19s%-14.11s%-6d%-20.19s%-20s%-7d\n", lib->ebooks[i].commonAttributes.id, lib->ebooks[i].commonAttributes.title, lib->ebooks[i].commonAttributes.author, lib->ebooks[i].commonAttributes.genre, lib->ebooks[i].commonAttributes.yearOfPublication, lib->ebooks[i].commonAttributes.publisher, lib->ebooks[i].commonAttributes.isbn, lib->ebooks[i].downloadCount); } } // display digital media void displayDigitalMedia(const struct Library* lib) { if (lib == NULL || lib->digitalMedia == NULL || lib->digitalMediaCount == 0) { printf("No digital media to display.\n"); return; } printf("------- DIGITAL MEDIA -------\n"); printf("ID Title Creator Genre Year Format Size Access Link Downloads\n"); printf("---------------------------------------------------------------------------------------------------------------------------------------------------------------\n"); // Display all digital media items for (int i = 0; i < lib->digitalMediaCount; i++) { printf("%-6d%-30.29s%-21.20s%-16.15s%-6d%-20.19s%-20s%-22.21s%-10d\n", lib->digitalMedia[i].id, lib->digitalMedia[i].title, lib->digitalMedia[i].creator, lib->digitalMedia[i].genre, lib->digitalMedia[i].yearOfRelease, lib->digitalMedia[i].format, lib->digitalMedia[i].fileSize, lib->digitalMedia[i].accessLink, lib->digitalMedia[i].downloadCount); } } // print histogram void printBookPublicationHistogram(const struct Library* lib) { if (lib == NULL || lib->books == NULL || lib->bookCount == 0) { printf("No books to display.\n"); return; } // Initialize an array to store the counts of books per year int bookYearCounts[NUM_YEARS] = { 0 }; // Count the books per year for (int i = 0; i < lib->bookCount; i++) { int year = lib->books[i].commonAttributes.yearOfPublication; if (year >= START_YEAR && year 0) { printf("%d: ", year + START_YEAR); for (int i = 0; i < bookYearCounts[year]; i++) { printf("*"); } printf(" (%d)\n", bookYearCounts[year]); } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can you fix the code please on the first picture shows the error output.
// Corrected code
#define _CRT_SECURE_NO_WARNINGS
#include "LibraryManagement.h"
#include "Books.h"
#include "DigitalMedia.h"
#include "LibraryConfig.h"
#include
#include
#include
#include // Include the necessary header for boolean data type
// Comparison function for qsort to sort Digital Media by ID
int compareDigitalMedia(const void* a, const void* b) {
return ((struct DigitalMedia*)a)->id - ((struct DigitalMedia*)b)->id;
}
// initializing library
struct Library initializeLibrary() {
struct Library lib;
lib.bookCount = 0;
lib.ebookCount = 0;
lib.digitalMediaCount = 0;
// Initialize book array
for (int i = 0; i < MAX_BOOK_COUNT; i++) {
lib.books[i].commonAttributes.id = -1; // Set an invalid ID to mark empty slot
}
// Initialize ebook array
for (int i = 0; i < MAX_EBOOK_COUNT; i++) {
lib.ebooks[i].commonAttributes.id = -1;
}
// Initialize digital media array
for (int i = 0; i < MAX_DIGITAL_MEDIA_COUNT; i++) {
lib.digitalMedia[i].id = -1;
}
return lib;
}
void loadBooks(struct Library* lib, const char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
perror("Error opening the file");
return;
}
char line[2048];
while (fgets(line, sizeof(line), file) != NULL) {
if (lib->bookCount >= MAX_BOOK_COUNT) {
printf("Reached the maximum book limit. Skipping the rest.\n");
break;
}
struct Book tempBook;
int readCount = sscanf(line, "%d;%127[^;];%127[^;];%127[^;];%d;%127[^;];%127[^;];%d;%127[^;];%d;%9[^;]",
&tempBook.commonAttributes.id, tempBook.commonAttributes.title,
tempBook.commonAttributes.author, tempBook.commonAttributes.genre,
&tempBook.commonAttributes.yearOfPublication, tempBook.commonAttributes.publisher,
tempBook.commonAttributes.isbn, &tempBook.numberOfPages, tempBook.condition,
&tempBook.numberOfCopies, tempBook.shelfLocation);
if (readCount == 11) {
strncpy(tempBook.commonAttributes.isbn, tempBook.commonAttributes.isbn, 13);
tempBook.commonAttributes.isbn[13] = '\0';
lib->books[lib->bookCount] = tempBook;
lib->bookCount++;
}
else {
printf("Failed to parse line: %s", line);
}
}
fclose(file);
}
// load digital media
void loadDigitalMedia(struct Library* lib, const char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
perror("Error opening the file");
return;
}
char line[2048];
int lineCount = 0;
while (fgets(line, sizeof(line), file) != NULL) {
lineCount++;
if (lib->digitalMediaCount >= MAX_DIGITAL_MEDIA_COUNT) {
printf("Reached the maximum digital media limit. Skipping the rest.\n");
break;
}
struct DigitalMedia tempDigitalMedia;
int readCount = sscanf(line, "%d;%2047[^;];%2047[^;];%2047[^;];%d;%2047[^;];%2047[^;];%d;%lf;%2047[^;];%d",
&tempDigitalMedia.id, tempDigitalMedia.title, tempDigitalMedia.creator,
tempDigitalMedia.genre, &tempDigitalMedia.yearOfRelease, tempDigitalMedia.format,
&tempDigitalMedia.fileSize, tempDigitalMedia.accessLink, &tempDigitalMedia.downloadCount);
if (readCount == 10) {
// Check for a valid year of release within the range (1800 - 2023)
if (tempDigitalMedia.yearOfRelease < 1800 || tempDigitalMedia.yearOfRelease > 2023) {
printf("Skipping digital media with an invalid year of release at line %d: %s\n", lineCount, tempDigitalMedia.title);
continue;
}
lib->digitalMedia[lib->digitalMediaCount] = tempDigitalMedia;
lib->digitalMediaCount++;
}
else {
printf("Failed to parse line %d: %s", lineCount, line);
}
}
fclose(file);
}
// load ebooks
void loadEbooks(struct Library* lib, const char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
perror("Error opening the file");
return;
}
char line[2048];
while (fgets(line, sizeof(line), file) != NULL) {
if (lib->ebookCount >= MAX_EBOOK_COUNT) {
printf("Reached the maximum eBook limit. Skipping the rest.\n");
break;
}
struct EBook tempEBook;
int readCount = sscanf(line, "%d;%50[^;];%50[^;];%50[^;];%d;%50[^;];%20[^;];%d",
&tempEBook.commonAttributes.id, tempEBook.commonAttributes.title,
tempEBook.commonAttributes.author, tempEBook.commonAttributes.genre,
&tempEBook.commonAttributes.yearOfPublication, tempEBook.commonAttributes.publisher,
tempEBook.commonAttributes.isbn);
if (readCount == 7) {
// Extract only the first 14 digits of ISBN
strncpy(tempEBook.commonAttributes.isbn, tempEBook.commonAttributes.isbn, 13);
tempEBook.commonAttributes.isbn[13] = '\0';
// Check for a valid publication year within the range (1800 - 2023)
if (tempEBook.commonAttributes.yearOfPublication < 1800 || tempEBook.commonAttributes.yearOfPublication > 2023) {
printf("Skipping eBook with an invalid publication year: %s\n", tempEBook.commonAttributes.title);
continue;
}
lib->ebooks[lib->ebookCount] = tempEBook;
lib->ebookCount++;
}
else {
printf("Failed to parse line: %s", line);
}
}
fclose(file);
}
// display books
void displayBooks(const struct Library* lib) {
// Check for valid library and book data
if (lib == NULL || lib->books == NULL || lib->bookCount == 0) {
printf("No books to display.\n");
return;
}
// Header printing
printf("\n------- BOOKS -------\n");
printf("ID Title Author Genre Year Publisher ISBN Shelf\n\n");
printf("------------------------------------------------------------------------------------------------------------------------\n\n");
// Content display
for (int i = 0; i < lib->bookCount; i++) {
// Ensure that strings are null-terminated
char title[MAX_TITLE_LEN + 1];
char author[MAX_AUTHOR_LEN + 1];
char genre[MAX_GENRE_LEN + 1];
char publisher[MAX_PUBLISHER_LEN + 1];
char isbn[MAX_ISBN_LEN + 1];
strncpy(title, lib->books[i].commonAttributes.title, MAX_TITLE_LEN);
strncpy(author, lib->books[i].commonAttributes.author, MAX_AUTHOR_LEN);
strncpy(genre, lib->books[i].commonAttributes.genre, MAX_GENRE_LEN);
strncpy(publisher, lib->books[i].commonAttributes.publisher, MAX_PUBLISHER_LEN);
strncpy(isbn, lib->books[i].commonAttributes.isbn, MAX_ISBN_LEN);
title[MAX_TITLE_LEN] = '\0';
author[MAX_AUTHOR_LEN] = '\0';
genre[MAX_GENRE_LEN] = '\0';
publisher[MAX_PUBLISHER_LEN] = '\0';
isbn[MAX_ISBN_LEN] = '\0';
// Print book details
printf("%-6d%-30.29s%-24.23s%-14.13s%-6d%-20.19s%-17.13s%-5s\n", lib->books[i].commonAttributes.id, title,
author, genre, lib->books[i].commonAttributes.yearOfPublication, publisher, isbn, lib->books[i].shelfLocation);
}
}
// display ebooks
void displayEbooks(const struct Library* lib, int flag) {
if (lib == NULL || lib->ebooks == NULL || lib->ebookCount == 0) {
printf("No eBooks to display.\n");
return;
}
int start = (flag == 0) ? 0 : (lib->ebookCount < 5) ? 0 : lib->ebookCount - 5;
int end = (flag == 0) ? ((lib->ebookCount < 5) ? lib->ebookCount : 5) : lib->ebookCount;
printf("------- %s EBOOKS -------\n", (flag == 0) ? "First Five" : "Last Five");
printf("ID Title Author Genre Year Publisher ISBN Access Link Downloads\n");
printf("-----------------------------------------------------------------------------------------------------------------------------------------------------------\n");
for (int i = start; i < end; i++) {
printf("%-6d%-30.29s%-24.19s%-14.11s%-6d%-20.19s%-20s%-7d\n", lib->ebooks[i].commonAttributes.id,
lib->ebooks[i].commonAttributes.title, lib->ebooks[i].commonAttributes.author,
lib->ebooks[i].commonAttributes.genre, lib->ebooks[i].commonAttributes.yearOfPublication,
lib->ebooks[i].commonAttributes.publisher, lib->ebooks[i].commonAttributes.isbn,
lib->ebooks[i].downloadCount);
}
}
// display digital media
void displayDigitalMedia(const struct Library* lib) {
if (lib == NULL || lib->digitalMedia == NULL || lib->digitalMediaCount == 0) {
printf("No digital media to display.\n");
return;
}
printf("------- DIGITAL MEDIA -------\n");
printf("ID Title Creator Genre Year Format Size Access Link Downloads\n");
printf("---------------------------------------------------------------------------------------------------------------------------------------------------------------\n");
// Display all digital media items
for (int i = 0; i < lib->digitalMediaCount; i++) {
printf("%-6d%-30.29s%-21.20s%-16.15s%-6d%-20.19s%-20s%-22.21s%-10d\n", lib->digitalMedia[i].id,
lib->digitalMedia[i].title, lib->digitalMedia[i].creator,
lib->digitalMedia[i].genre, lib->digitalMedia[i].yearOfRelease,
lib->digitalMedia[i].format, lib->digitalMedia[i].fileSize,
lib->digitalMedia[i].accessLink, lib->digitalMedia[i].downloadCount);
}
}
// print histogram
void printBookPublicationHistogram(const struct Library* lib) {
if (lib == NULL || lib->books == NULL || lib->bookCount == 0) {
printf("No books to display.\n");
return;
}
// Initialize an array to store the counts of books per year
int bookYearCounts[NUM_YEARS] = { 0 };
// Count the books per year
for (int i = 0; i < lib->bookCount; i++) {
int year = lib->books[i].commonAttributes.yearOfPublication;
if (year >= START_YEAR && year 0) {
printf("%d: ", year + START_YEAR);
for (int i = 0; i < bookYearCounts[year]; i++) {
printf("*");
}
printf(" (%d)\n", bookYearCounts[year]);
}
}
}
![Error List - Current Project (LibraryManagement System) ******
Current Project
X 2 Errors
15 Warnings
C...
10 of 9 Messages 97 Build+ IntelliSense
Description
declared formal parameter list different from definition
'sscanf': not enough arguments passed for format string
'sscanf': not enough arguments passed for format string
'sscanf': format string '%2047[^]' requires an argument of type 'char *', but variadic argument 7 has type 'double*
'sscanf': format string '%d' requires an argument of type 'int **, but variadic argument 8 has type 'char *¹
'sscanf': format string '%lf' requires an argument of type 'double *, but variadic argument 9 has type 'int **
C4477 'printf': format string '%-20s' requires an argument of type 'char *, but variadic argument 7 has type 'const double'
C6063 Missing string argument to 'sscanf' that corresponds to conversion specifier '10'.
C6064 Missing integer argument to 'sscanf' that corresponds to conversion specifier '11'.
C6064 Missing integer argument to 'sscanf' that corresponds to conversion specifier '8'.
C6067 _Param_(9) in call to 'sscanf' must be the address of a string. Actual type: 'double *.
C6067 _Param_(8) in call to 'printf' must be the address of a string. Actual type: 'const double'.
C6262 Function uses '224028' bytes of stack. Consider moving some data to heap.
A C6272
Non-float passed as argument '11' when float is required in call to 'sscanf' Actual type: 'int'.
C6328
Size mismatch: 'char' passed as_Param_(10) when '32 bit operand' is required in call to 'sscanf'. This indicates a potential
functions.
LNK1120 1 unresolved externals
XLNK2019 unresolved external symbol main referenced in function "int_cdecl invoke_main(void)" (?invoke_main@@YAHXZ)
C4029
A C4473
A C4473
A C4477
A C4477
C4477](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb264107d-fe28-4156-b6f7-f464b838b63d%2Fa2516883-441a-4085-ae02-8bb6f72c1236%2Fh94vs8_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Error List - Current Project (LibraryManagement System) ******
Current Project
X 2 Errors
15 Warnings
C...
10 of 9 Messages 97 Build+ IntelliSense
Description
declared formal parameter list different from definition
'sscanf': not enough arguments passed for format string
'sscanf': not enough arguments passed for format string
'sscanf': format string '%2047[^]' requires an argument of type 'char *', but variadic argument 7 has type 'double*
'sscanf': format string '%d' requires an argument of type 'int **, but variadic argument 8 has type 'char *¹
'sscanf': format string '%lf' requires an argument of type 'double *, but variadic argument 9 has type 'int **
C4477 'printf': format string '%-20s' requires an argument of type 'char *, but variadic argument 7 has type 'const double'
C6063 Missing string argument to 'sscanf' that corresponds to conversion specifier '10'.
C6064 Missing integer argument to 'sscanf' that corresponds to conversion specifier '11'.
C6064 Missing integer argument to 'sscanf' that corresponds to conversion specifier '8'.
C6067 _Param_(9) in call to 'sscanf' must be the address of a string. Actual type: 'double *.
C6067 _Param_(8) in call to 'printf' must be the address of a string. Actual type: 'const double'.
C6262 Function uses '224028' bytes of stack. Consider moving some data to heap.
A C6272
Non-float passed as argument '11' when float is required in call to 'sscanf' Actual type: 'int'.
C6328
Size mismatch: 'char' passed as_Param_(10) when '32 bit operand' is required in call to 'sscanf'. This indicates a potential
functions.
LNK1120 1 unresolved externals
XLNK2019 unresolved external symbol main referenced in function "int_cdecl invoke_main(void)" (?invoke_main@@YAHXZ)
C4029
A C4473
A C4473
A C4477
A C4477
C4477
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
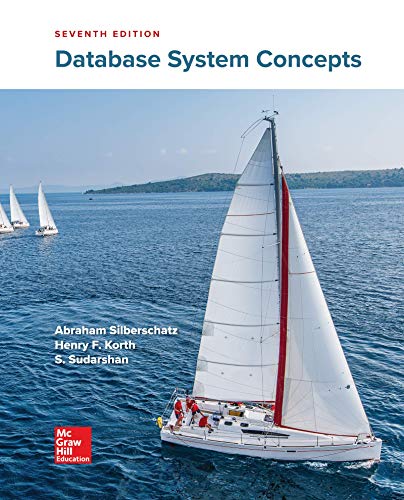
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
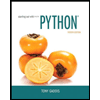
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
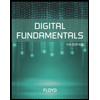
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
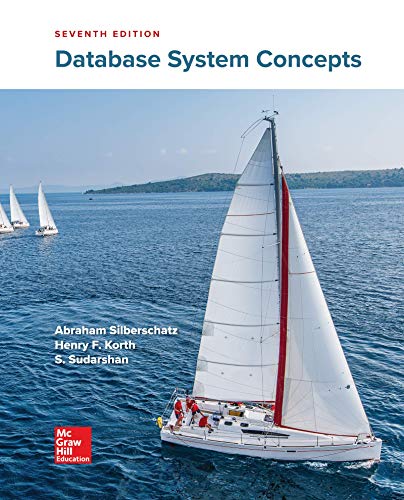
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
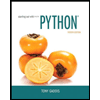
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
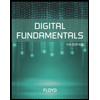
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
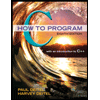
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
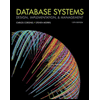
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
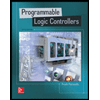
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education