Java Code: How to implement logic for ParseDoWhile, ParseDelete, and ParseReturn where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.
Java Code: How to implement logic for ParseDoWhile, ParseDelete, and ParseReturn where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.

1. Start
2. Define the 'Token' class to represent lexical tokens with 'type' and 'lexeme' attributes.
3. Define an enumeration 'TokenType' to represent different token types.
4. Define classes for different AST nodes, including 'Node', 'StatementNode', 'DoWhileNode', 'DeleteNode', 'ReturnNode', and 'IdentifierNode'.
5. Create a 'Parser' class to handle parsing logic for the input tokens.
6. In the 'Parser' class:
a. Initialize the parser with a list of tokens.
b. Implement methods to parse 'do-while' statements, 'delete' statements, and 'return' statements, including error handling.
c. Create simplified methods for parsing expressions and blocks (not shown in detail).
d. Implement 'check', 'match', 'advance', and 'isAtEnd' helper methods to manage token consumption.
7. In the 'Main' class:
a. Define a list of sample tokens representing input code.
b. Initialize a 'Parser' with the sample tokens.
c. Parse 'do-while', 'delete', and 'return' statements using the parser, handling potential parse errors.
d. You can further process and execute the parsed statements as needed.
8. End.
Step by step
Solved in 4 steps with 7 images

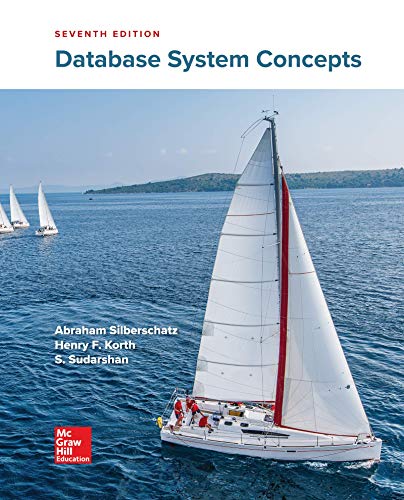
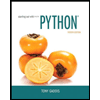
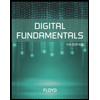
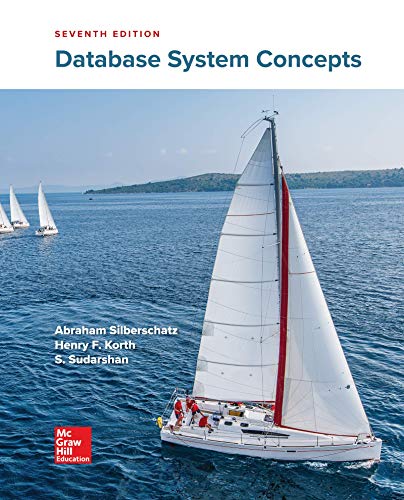
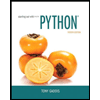
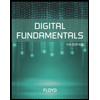
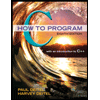
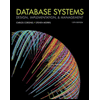
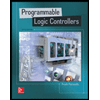