Suppose you are trapped on a desert island with nothing but a priority queue, and you need to implement a stack. Complete the following class that stores pairs (count, element) where the count is incremented with each insertion. Recall that make_pair(count, element) yields a pair object, and that p.second yields the second component of a pair p. The pair class defines an operator< that compares pairs by their first component, and uses the second component only to break ties. Code: #include #include #include #include using namespace std; class Stack { public: Stack(); string top(); void pop(); void push(string element); private: int count; priority_queue> pqueue; }; Stack::Stack() { /* Your code goes here */ } string Stack::top() { /* Your code goes here */ } void Stack::pop() { /* Your code goes here */ } void Stack::push(string element) { /* Your code goes here */ } int main() { Stack stk; stk.push("Mary"); stk.push("had"); stk.push("a"); cout << stk.top() << endl; stk.pop(); cout << "Expected: a\n" << endl; stk.push("little"); cout << stk.top() << endl; stk.pop(); cout << "Expected: little\n" << endl; cout << stk.top() << endl; stk.pop(); cout << "Expected: had\n" << endl; stk.push("lamb"); cout << stk.top() << endl; stk.pop(); cout << "Expected: lamb\n" << endl; cout << stk.top() << endl; stk.pop(); cout << "Expected: Mary" << endl;
Suppose you are trapped on a desert island with nothing but a priority queue, and you need to implement a stack. Complete the following class that stores pairs (count, element) where the count is incremented with each insertion.
Recall that make_pair(count, element) yields a pair object, and that p.second yields the second component of a pair p.
The pair class defines an operator< that compares pairs by their first component, and uses the second component only to break ties.
Code:
#include <iostream>
#include <queue>
#include <string>
#include <utility>
using namespace std;
class Stack
{
public:
Stack();
string top();
void pop();
void push(string element);
private:
int count;
priority_queue<pair<int, string>> pqueue;
};
Stack::Stack()
{
/* Your code goes here */
}
string Stack::top()
{
/* Your code goes here */
}
void Stack::pop()
{
/* Your code goes here */
}
void Stack::push(string element)
{
/* Your code goes here */
}
int main()
{
Stack stk;
stk.push("Mary");
stk.push("had");
stk.push("a");
cout << stk.top() << endl; stk.pop();
cout << "Expected: a\n" << endl;
stk.push("little");
cout << stk.top() << endl; stk.pop();
cout << "Expected: little\n" << endl;
cout << stk.top() << endl; stk.pop();
cout << "Expected: had\n" << endl;
stk.push("lamb");
cout << stk.top() << endl; stk.pop();
cout << "Expected: lamb\n" << endl;
cout << stk.top() << endl; stk.pop();
cout << "Expected: Mary" << endl;
}

Step by step
Solved in 4 steps with 2 images

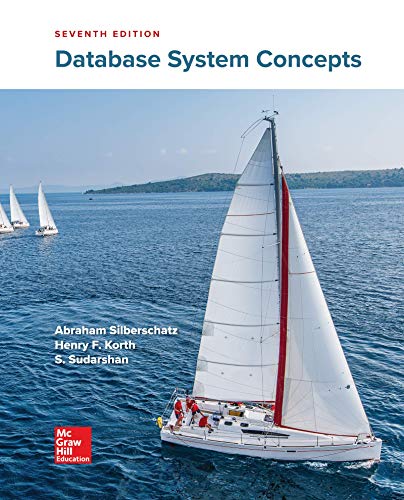
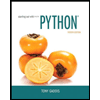
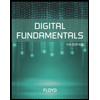
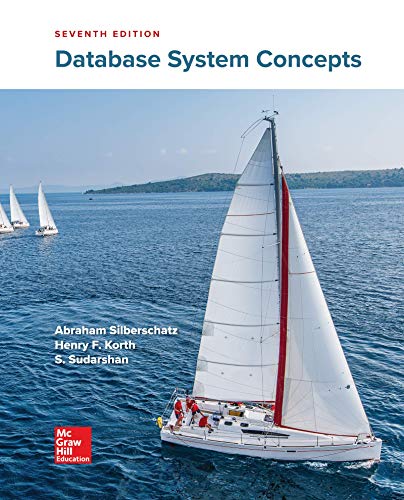
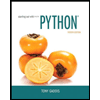
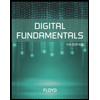
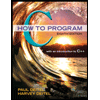
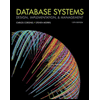
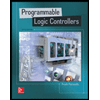