Assume you have a class called Deck which represents a deck of cards using a singly linked lists. Each node is an object of type Card with a field called next where a reference to the next Card is stored. A field from the Deck class called head stores a reference to the card on the top of the deck, and a field called tail stores a reference to the last card, at the bottom of the deck. We can cut a deck of cards by performing what is called a triple cut: the deck is partitioned into three parts and the bottom part is positioned between the first two. You would like to write a method that implements such a cut on your deck data structure. The method will take as input two cards, the first one being the last card of the first part of the deck, and the second being the first one of the third part of the deck. For example, assume a deck contains cards in the following order AC 2C 3C 4C 5C AD 2D 3D 4D 5D After calling tripleCut() on the deck the card, with input a reference to 3C and a reference to 2D in that order, then the deck should appear in the following order: AC 2C 3C 2D 3D 4D 5D 4C 5C AD Here is the code for the method tripleCut() : public void tripleCut(Card first, Card second) { Card c = first.next; ***BLANK_1*** ***BLANK_2*** while (c.next != second) { ***BLANK_3*** } ***BLANK_4*** ***BLANK_5*** } Place the following instructions in the correct order to complete the implementation of the method. Note: you can assume that the inputs are not null, and that first references a card that appears appears in the deck before the one referenced by second. Question 1 options: tail.next = c; first.next = second; c.next = null; c = c.next; tail = c;
Assume you have a class called Deck which represents a deck of cards using a singly linked lists. Each node is an object of type Card with a field called next where a reference to the next Card is stored. A field from the Deck class called head stores a reference to the card on the top of the deck, and a field called tail stores a reference to the last card, at the bottom of the deck.
We can cut a deck of cards by performing what is called a triple cut: the deck is partitioned into three parts and the bottom part is positioned between the first two. You would like to write a method that implements such a cut on your deck data structure. The method will take as input two cards, the first one being the last card of the first part of the deck, and the second being the first one of the third part of the deck.
For example, assume a deck contains cards in the following order
AC 2C 3C 4C 5C AD 2D 3D 4D 5D
After calling tripleCut() on the deck the card, with input a reference to 3C and a reference to 2D in that order, then the deck should appear in the following order:
AC 2C 3C 2D 3D 4D 5D 4C 5C AD
Here is the code for the method tripleCut() :
public void tripleCut(Card first, Card second) {
Card c = first.next;
***BLANK_1***
***BLANK_2***
while (c.next != second) {
***BLANK_3***
}
***BLANK_4***
***BLANK_5***
}
Place the following instructions in the correct order to complete the implementation of the method. Note: you can assume that the inputs are not null, and that first references a card that appears appears in the deck before the one referenced by second.
Question 1 options:
|
tail.next = c;
|
|
first.next = second;
|
|
c.next = null;
|
|
c = c.next;
|
|
tail = c;
|

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

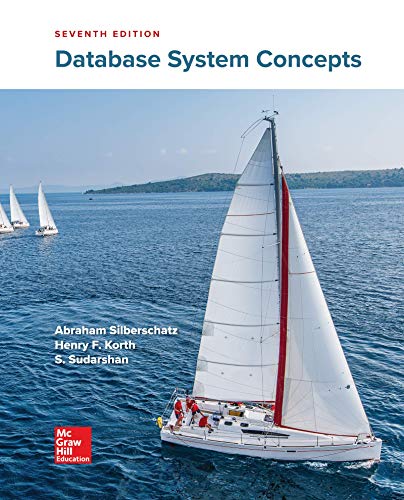
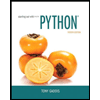
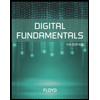
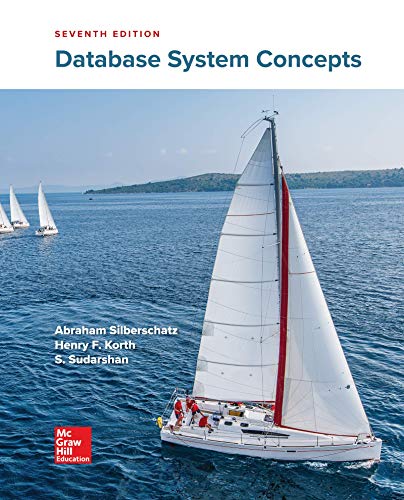
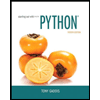
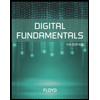
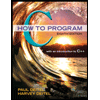
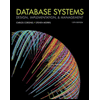
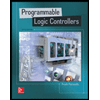