You may do this assignment using either Java or C++. Do *not use the JDK LinkedList class or any linked list library! A linked list consists of zero or more nodes, which each contain one item of data and a link (Java reference, C++ pointer or reference) to the next node, if there is one.
You may do this assignment using either Java or C++. Do *not use the JDK LinkedList class or any linked list library! A linked list consists of zero or more nodes, which each contain one item of data and a link (Java reference, C++ pointer or reference) to the next node, if there is one.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:You may do this assignment using either Java or C++. Do *not use the JDK LinkedList class
or any linked list library!
A linked list consists of zero or more nodes, which each contain one item of data and a link
(Java reference, C++ pointer or reference) to the next node, if there is one.
Linked List code is usually generic, so that you can use it to create a list of Strings, Students,
Doubles, etc. For this assignment, you will write a simplified version of linked list that only
can be used to create and use a list of ints and that has only a few of the functions typical of
linked lists. The data element can be just an int, and the data type of the link to the next
node can be just Node.
You will need a reference (Java) or either a reference or a pointer (C++) to the first node, as
well as one to the last node.
Write a method/function that takes an in, creates a node with that int as its data value, and
adds the node to the end of the list. This function will need to update the reference to the
last node, and if the new node is the first one, it will also need to update the reference to the
first node. Think about how to add the new node after the former last node, and remember
that the next reference for the last node in the list should be null.
Write another method/function that traverses the list, starting with the first node and
following the links until it reached the last node, and prints out the int data values.
Write another function/method that takes an int parameter and determines whether that int
is contained in the list.
Write either a main() method or JUnit tests to demonstrate that your list code works
correctly. Create a list, add some nodes, search the list for a value that is *not* in the list,
then for a value that is in the list. Also create a list with no nodes and search it for some
value.
Turn in your code and a screenshot of the output from running your main() or tests.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
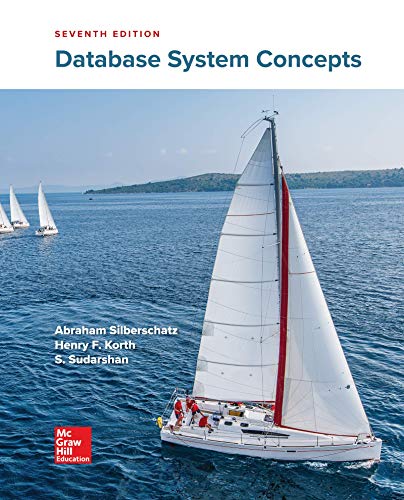
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
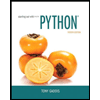
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
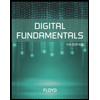
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
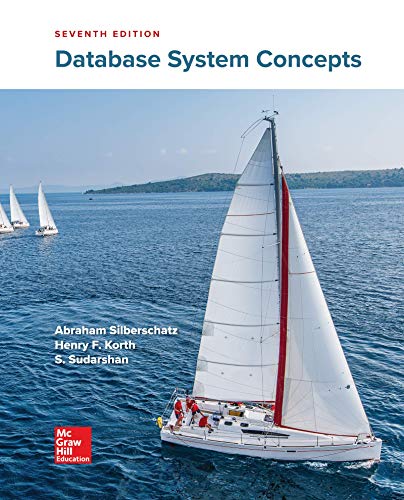
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
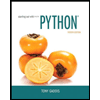
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
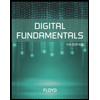
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
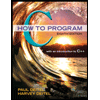
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
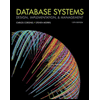
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
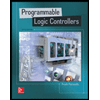
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education