OCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly without any errors and provide the code with the screenshots of the outputs. The code must have a function that takes a pair of strings (tuple) and returns a unit. Make sure to have the following methods below: -Push integers, strings, and names on the stack -Push booleans -Pushing an error literal or unit literal will push :error: or :unit: onto the stack, respectively -Command pop removes the top value from the stack -The command add refers to integer addition. Since this is a binary operator, it consumes the top two values in the stack, calculates the sum and pushes the result back to the stack - Command sub refers to integer subtraction where if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), subtract y from x, and push the result x-y back onto the stack -Command mul refers to integer multiplication where if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), multiply x by y, and push the result x*y back onto the stack -Command div refers to integer division. It is a binary operator and works in the following way: • if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), divide x by y, and push the result x y back onto the stack • if top two elements in the stack are integer numbers but y equals to 0, push them back in the same order and push :error: onto the stack • if the top two elements in the stack are not both integer numbers, push them back in the same order and push :error: onto the stack • if there is only one element in the stack, push it back and push :error: onto the stack • if the stack is empty, push :error: onto the stack -Command rem refers to the remainder of integer division. It is a binary operator and works in the following way: • if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), calculate the remainder of x y , and push the result back onto the stack • if top two elements in the stack are integer numbers but y equals to 0, push them back in the same order and push :error: onto the stack • if the top two elements in the stack are not all integer numbers, push them back and push :error: onto the stack • if there is only one element in the stack, push it back and push :error: onto the stack • if the stack is empty, push :error: onto the stack -Command neg is to calculate the negation of an integer. It is unary therefore consumes only the top element from the stack, calculate its negation and push the result back. A value :error: will be pushed onto the stack if: • the top element is not an integer, push the top element back and push :error: • the stack is empty, push :error: onto the stack -Command swap interchanges the top two elements in the stack, meaning that the first element becomes the second and the second becomes the first. A value :error: will be pushed onto the stack if: • there is only one element in the stack, push the element back and push :error: • the stack is empty, push :error: onto the stack -Command toString removes the top element of the stack and converts it to a string. If the stack is empty, the error value is pushed instead. Conversions of values to strings should be done as follows: • Integers shall be rendered as decimal values with no leading zeros. Negative integers should be prefixed with a ‘-’. • Booleans shall be rendered as the values “:true” and “:false:”. • Error values shall be rendered as “:error:” • Unit values shall be rendered as “:unit:” • String values should be left unchanged (i.e. surrounding punctuation may not be added) • Names shall be converted to the corresponding string, contents unchanged. • Closures shall be rendered as “:fun:” -println command pops a string off the top of the stack and writes it, followed by a newline, to the output file that is specified as the second argument to the interpreter function. In the case that the top element on the stack is not a string, it should be returned to the stack and an :error: pushed. If the stack is empty, an :error: shall be pushed. -Command quit causes the interpreter to stop Below are the test cases: input1.txt: push 1 toString println quit input2.txt: push 5 push :true: push :false: push "str1" push str1 push :error: push :unit: toString println toString println toString println toString println toString println toString println toString println quit input3.txt: push 10 push 2 push 8 pop add toString println quit input4.txt: push 6 push 2 push 3 sub add toString println quit input5.txt: push :true: push 7 push 8 push :false: pop mul toString println toString println quit output1.txt: 1 output2.txt: :unit: :error: str1 str1 :false: :true: 5 output3.txt: 12 output4.txt: 5 output5.txt: 56 :true:
OCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly without any errors and provide the code with the screenshots of the outputs. The code must have a function that takes a pair of strings (tuple) and returns a unit. Make sure to have the following methods below:
-Push integers, strings, and names on the stack
-Push booleans
-Pushing an error literal or unit literal will push :error: or :unit: onto the stack, respectively
-Command pop removes the top value from the stack
-The command add refers to integer addition. Since this is a binary operator, it consumes the top two values in the stack, calculates the sum and pushes the result back to the stack
- Command sub refers to integer subtraction where if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), subtract y from x, and push the result x-y back onto the stack
-Command mul refers to integer multiplication where if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), multiply x by y, and push the result x*y back onto the stack
-Command div refers to integer division. It is a binary operator and works in the following way:
• if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), divide x by y, and push the result x y back onto the stack
• if top two elements in the stack are integer numbers but y equals to 0, push them back in the same order and push :error: onto the stack
• if the top two elements in the stack are not both integer numbers, push them back in the same order and push :error: onto the stack
• if there is only one element in the stack, push it back and push :error: onto the stack
• if the stack is empty, push :error: onto the stack
-Command rem refers to the remainder of integer division. It is a binary operator and works in the following way:
• if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), calculate the remainder of x y , and push the result back onto the stack
• if top two elements in the stack are integer numbers but y equals to 0, push them back in the same order and push :error: onto the stack
• if the top two elements in the stack are not all integer numbers, push them back and push :error: onto the stack
• if there is only one element in the stack, push it back and push :error: onto the stack
• if the stack is empty, push :error: onto the stack
-Command neg is to calculate the negation of an integer. It is unary therefore consumes only the top element from the stack, calculate its negation and push the result back. A value :error: will be pushed onto the stack if:
• the top element is not an integer, push the top element back and push :error:
• the stack is empty, push :error: onto the stack
-Command swap interchanges the top two elements in the stack, meaning that the first element becomes the second and the second becomes the first. A value :error: will be pushed onto the stack if:
• there is only one element in the stack, push the element back and push :error:
• the stack is empty, push :error: onto the stack
-Command toString removes the top element of the stack and converts it to a string. If the stack is empty, the error value is pushed instead. Conversions of values to strings should be done as follows:
• Integers shall be rendered as decimal values with no leading zeros. Negative integers should be prefixed with a ‘-’.
• Booleans shall be rendered as the values “:true” and “:false:”.
• Error values shall be rendered as “:error:”
• Unit values shall be rendered as “:unit:”
• String values should be left unchanged (i.e. surrounding punctuation may not be added)
• Names shall be converted to the corresponding string, contents unchanged.
• Closures shall be rendered as “:fun:”
-println command pops a string off the top of the stack and writes it, followed by a newline, to the output file that is specified as the second argument to the interpreter function. In the case that the top element on the stack is not a string, it should be returned to the stack and an :error: pushed. If the stack is empty, an :error: shall be pushed.
-Command quit causes the interpreter to stop
Below are the test cases:
input1.txt:
push 1
toString
println
quit
input2.txt:
push 5
push :true:
push :false:
push "str1"
push str1
push :error:
push :unit:
toString
println
toString
println
toString
println
toString
println
toString
println
toString
println
toString
println
quit
input3.txt:
push 10
push 2
push 8
pop
add
toString
println
quit
input4.txt:
push 6
push 2
push 3
sub
add
toString
println
quit
input5.txt:
push :true:
push 7
push 8
push :false:
pop
mul
toString
println
toString
println
quit
output1.txt:
1
output2.txt:
:unit:
:error:
str1
str1
:false:
:true:
5
output3.txt:
12
output4.txt:
5
output5.txt:
56
:true:

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 12 images

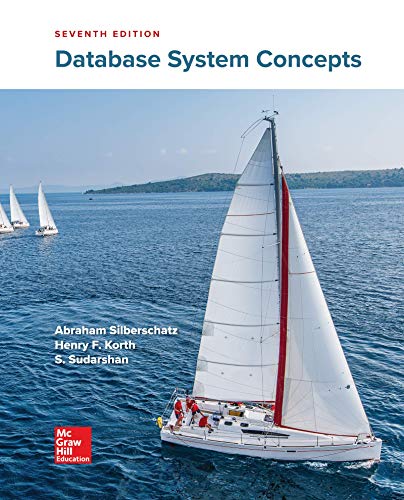
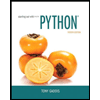
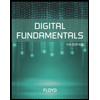
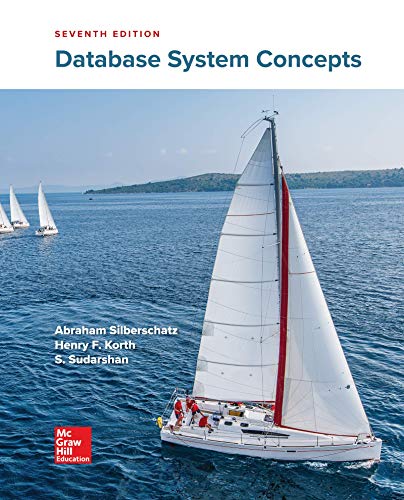
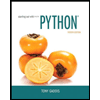
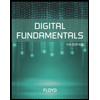
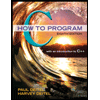
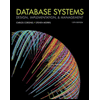
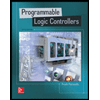