Java Programming: Make AST Nodes: IfNode, WhileNode, RepeatNode. All will have BooleanCompare for a condition and a collection of StatementNode. ForNode will have a Node for from and a node for to. This will have to be of type Node because it could be any expression. For example: for a from 1+1 to c-6 Make parsing functions for each. Java won't let you create methods called if(), etc. parseIf() is an example of a way around that; use whatever you like but use good sense in your names. Next let's look at function calls. Each function has a name and a collection of parameters. A parameter can be a VAR variable or something that came from booleanCompare (IntegerNode, VariableReferenceNode, etc). It would be reasonable to make 2 objects - ParameterVariableNode and ParameterExpressionNode. But for this very simple and well-defined case, we can do something simple: ParameterNode has a VariableReferenceNode (for VAR IDENTIFIER) and a Node for the case where the parameter is not a VAR. One more thing - type limits. Remember that these are a way to put a range on your variables. variables numberOfCards : integer from 0 to 52 These apply to integer, real, string. Array already has this built in with the from and to. We can reuse that from/to range. We will have to make a mirror pair (realFrom, realTo - both float) for supporting ranges on real. Make the variable declaration parser changes and add the floating-point ranges to VariableNode. Below is what should AST claases and Parse.java must have. Make sure to give full working codes & output: IfNode: Has constructor(s), ToString, Conditions, Statements, NextIf WhileNode: Has constructor(s), ToString, Conditions, Statements ForNode: Has constructor(s), ToString, from, to, Statements RepeatNode: Has constructor(s), ToString, Conditions, Statements FunctionCallNode: Has constructor(s), ToString, name, collection of parameters ParameterNode: Has VariableReferenceNode, Node, constructor(s), ToString() ParseFor(): Parses complex for statements correctly ParseWhile(): Parses complex while statements correctly ParseIf(): Parses complex, chained if statements correctly ParseFunctionCalls(): Parses complex function calls with expressions and vars correctly Ranges: Implemented for integers, strings, reals
Java
Make AST Nodes: IfNode, WhileNode, RepeatNode. All will have BooleanCompare for a condition and a collection of StatementNode.
ForNode will have a Node for from and a node for to. This will have to be of type Node because it could be any expression. For example:
for a from 1+1 to c-6
Make parsing functions for each. Java won't let you create methods called if(), etc. parseIf() is an example of a way around that; use whatever you like but use good sense in your names.
Next let's look at function calls. Each function has a name and a collection of parameters. A parameter can be a VAR variable or something that came from booleanCompare (IntegerNode, VariableReferenceNode, etc). It would be reasonable to make 2 objects - ParameterVariableNode and ParameterExpressionNode. But for this very simple and well-defined case, we can do something simple:
ParameterNode has a VariableReferenceNode (for VAR IDENTIFIER) and a Node for the case where the parameter is not a VAR.
One more thing - type limits. Remember that these are a way to put a range on your variables.
variables numberOfCards : integer from 0 to 52
These apply to integer, real, string. Array already has this built in with the from and to. We can reuse that from/to range. We will have to make a mirror pair (realFrom, realTo - both float) for supporting ranges on real.
Make the variable declaration parser changes and add the floating-point ranges to VariableNode.
Below is what should AST claases and Parse.java must have. Make sure to give full working codes & output:
IfNode: Has constructor(s), ToString, Conditions, Statements, NextIf
WhileNode: Has constructor(s), ToString, Conditions, Statements
ForNode: Has constructor(s), ToString, from, to, Statements
RepeatNode: Has constructor(s), ToString, Conditions, Statements
FunctionCallNode: Has constructor(s), ToString, name, collection of parameters
ParameterNode: Has VariableReferenceNode, Node, constructor(s), ToString()
ParseFor(): Parses complex for statements correctly
ParseWhile(): Parses complex while statements correctly
ParseIf(): Parses complex, chained if statements correctly
ParseFunctionCalls(): Parses complex function calls with expressions and vars correctly
Ranges: Implemented for integers, strings, reals

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

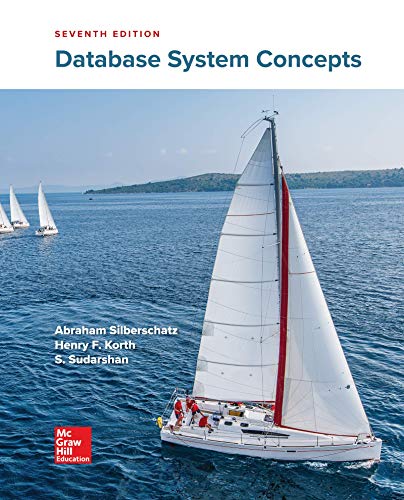
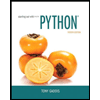
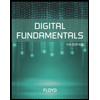
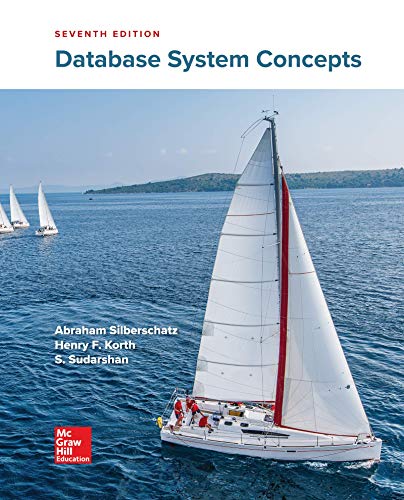
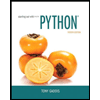
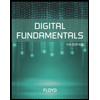
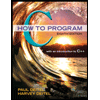
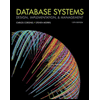
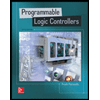