Make AST Nodes: IfNode, WhileNode, RepeatNode. All will have BooleanCompare for a condition and a collection of StatementNode. ForNode will have a Node for from and a node for to. This will have to be of type Node because it could be any expression. For example: for a from 1+1 to c-6 Make parsing functions for each. Java won't let you create methods called if(), etc. parseIf() is an example of a way around that; use whatever you like but use good sense in your names. Next let's look at function calls. Each function has a name and a collection of parameters. A parameter can be a VAR variable or something that came from booleanCompare (IntegerNode, VariableReferenceNode, etc). It would be reasonable to make 2 objects - ParameterVariableNode and ParameterExpressionNode. But for this very simple and well-defined case, we can do something simple: ParameterNode has a VariableReferenceNode (for VAR IDENTIFIER) and a Node for the case where the parameter is not a VAR. One more thing - type limits. Remember that these are a way to put a range on your variables. variables numberOfCards : integer from 0 to 52 These apply to integer, real, string. Array already has this built in with the from and to. We can reuse that from/to range. We will have to make a mirror pair (realFrom, realTo - both float) for supporting ranges on real. Make the variable declaration parser changes and add the floating-point ranges to VariableNode. Make sure to involve a parser.java to include all the functions for the parser as shown in the rubric. Below is the shank.txt that must be printed out as the output and attached is rubric. Make sure there are no errors in any of the files at all. shank.txt Fibonoacci (Iterative) define add (num1,num2:integer var sum : integer) variable counter : integer Finonacci(N) int N = 10; while counter < N define start () variables num1,num2,num3 : integer add num1,num2,var num3 {num1 and num2 are added together to get num3} num1 = num2; num2 = num3; counter = counter + 1; GCD (Recursive) define add (int a,int b : gcd) if b = 0 sum = a sum gcd(b, a % b) GCD (Iterative) define add (inta, intb : gcd) if a = 0 sum = b if b = 0 sum = a while counter a != b if a > b a = a - b; else b = b - a; sum = a; variables a,b : integer a = 60 b = 96 subtract a,b
Java
Make AST Nodes: IfNode, WhileNode, RepeatNode. All will have BooleanCompare for a condition and a collection of StatementNode.
ForNode will have a Node for from and a node for to. This will have to be of type Node because it could be any expression. For example:
for a from 1+1 to c-6
Make parsing functions for each. Java won't let you create methods called if(), etc. parseIf() is an example of a way around that; use whatever you like but use good sense in your names.
Next let's look at function calls. Each function has a name and a collection of parameters. A parameter can be a VAR variable or something that came from booleanCompare (IntegerNode, VariableReferenceNode, etc). It would be reasonable to make 2 objects - ParameterVariableNode and ParameterExpressionNode. But for this very simple and well-defined case, we can do something simple:
ParameterNode has a VariableReferenceNode (for VAR IDENTIFIER) and a Node for the case where the parameter is not a VAR.
One more thing - type limits. Remember that these are a way to put a range on your variables.
variables numberOfCards : integer from 0 to 52
These apply to integer, real, string. Array already has this built in with the from and to. We can reuse that from/to range. We will have to make a mirror pair (realFrom, realTo - both float) for supporting ranges on real.
Make the variable declaration parser changes and add the floating-point ranges to VariableNode.
Make sure to involve a parser.java to include all the functions for the parser as shown in the rubric.
Below is the shank.txt that must be printed out as the output and attached is rubric. Make sure there are no errors in any of the files at all.
shank.txt
Fibonoacci (Iterative)
define add (num1,num2:integer var sum : integer)
variable counter : integer
Finonacci(N)
int N = 10;
while counter < N
define start ()
variables num1,num2,num3 : integer
add num1,num2,var num3
{num1 and num2 are added together to get num3}
num1 = num2;
num2 = num3;
counter = counter + 1;
GCD (Recursive)
define add (int a,int b : gcd)
if b = 0
sum = a
sum gcd(b, a % b)
GCD (Iterative)
define add (inta, intb : gcd)
if a = 0
sum = b
if b = 0
sum = a
while counter a != b
if a > b
a = a - b;
else
b = b - a;
sum = a;
variables a,b : integer
a = 60
b = 96
subtract a,b


Step by step
Solved in 3 steps

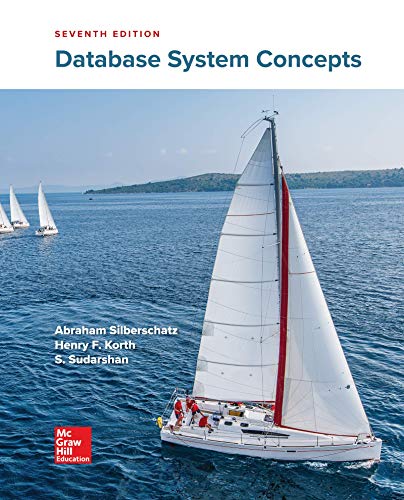
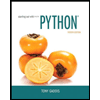
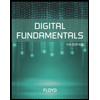
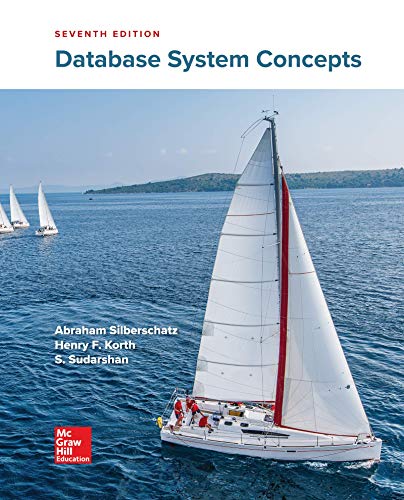
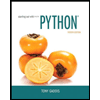
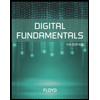
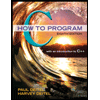
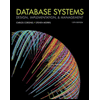
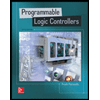