Implement a recursive function void deleteMax() on the IntList class (provided). The function will delete from the IntList the IntNode containing the largest value. If there are multiple nodes containing this largest value, only delete the 1st one. Be careful not to cause any memory leaks or dangling pointers. You may NOT use any kind of loop (must use recursion). You may NOT use global or static variables. You may NOT use any s
Implement a recursive function void deleteMax() on the IntList class (provided). The function will delete from the IntList the IntNode containing the largest value. If there are multiple nodes containing this largest value, only delete the 1st one.
Be careful not to cause any memory leaks or dangling pointers.
You may NOT use any kind of loop (must use recursion).
You may NOT use global or static variables.
You may NOT use any standard library functions.
Ex:
list: 5->7->1->16->4->16->3
list.deleteMax();
list: 5->7->1->4->16->3
IntList.h
#ifndef __INTLIST_H__
#define __INTLIST_H__
#include <ostream>
using namespace std;
struct IntNode {
int value;
IntNode *next;
IntNode(int value) : value(value), next(nullptr) {}
};
class IntList {
private:
IntNode *head;
public:
/* Initializes an empty list.
*/
IntList() : head(nullptr) {}
/* Inserts a data value to the front of the list.
*/
void push_front(int val) {
if (!head) {
head = new IntNode(val);
} else {
IntNode *temp = new IntNode(val);
temp->next = head;
head = temp;
}
}
/* Outputs to a single line all of the int values stored in the list, each separated by a space.
This function does NOT output a newline or space at the end.
*/
friend ostream & operator<<(ostream &out, const IntList &rhs) {
if (rhs.head) {
IntNode *curr = rhs.head;
out << curr->value;
for (curr = curr->next ; curr ; curr = curr->next) {
out << ' ' << curr->value;
}
}
return out;
}
/* Deletes the 1st instance of the largest value from the list,
keeping all other values in the same relative order.
*/
void deleteMax();
private:
/* Recursive helper function that passes in a node (actually the address of a node)
and the largest value that exists from the head to curr (inclusive).
This function should delete the node after curr if that node contains the first instance
of the largest value within the entire list.
This function returns the largest integer that exists (or existed if deleted) after the curr node.
*/
int deleteMax(IntNode *curr, int leftMaxVal);
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

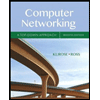
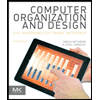
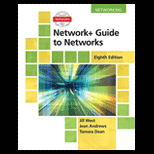
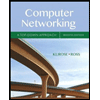
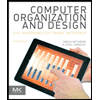
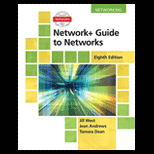
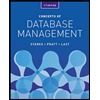
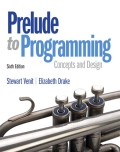
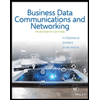