Write all the code within the main method in the Test Truck class below. Implement the following functionality. a) Constructs two truck objects: one with any make and model you choose and the second object with gas tank capacity 10. b) If an exception occurs, print the stack trace. c) Prints both truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main ( String [] args ) { heres the truck class information A Truck can be described as having a make (string), model (string), gas tank capacity (double), and whether it has a manual transmission (or not). Include the following methods in your class definition. . An overloaded constructor which takes the make and model. This method throws an IllegalArgumentException if the make is "Jeep". An overloaded constructor which takes the gas tank capacity. This method throws an IllegalArgumentException if the capacity of the gas tank is not between 15 and 20 gallons (inclusive). . That's 3 constructors in total. Each constructor, once done executing, should have fully initialized all of the object's properties .a default constructor Do not implement a toString method; assume one is properly defined for you. . Do not implement any getters or setters. In java class Truck { private String make, model; private double capacity; boolean manualTransmission; //Default constructor public Truck() { make = model = ""; capacity = 0.0; manualTransmission = false; } //Overloaded constructor public Truck(String ma, String mo) { if(ma.equals("Jeep")) throw new IllegalArgumentException(Integer.toString(ma)); else { make = ma; model = mo; capacity = 0.0; manualTransmission = false; } } //Overloaded constructor public Truck(double cap) { if(cap >= 15 && cap <= 20) throw new IllegalArgumentException(Integer.toString(cap)); else { make = ""; model = ""; capacity = cap; manualTransmission = false; } } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Write all the code within the main method in the Test Truck class below. Implement the following functionality.
a) Constructs two truck objects: one with any make and model you
choose and the second object with gas tank capacity 10.
b) If an exception occurs, print the stack trace.
c) Prints both truck objects that were constructed.
import java.lang.IllegalArgumentException ;
public class TestTruck
{
public static void main ( String [] args )
{
heres the truck class information
A Truck can be described as having a make (string), model (string), gas tank capacity (double), and whether it has a manual transmission (or not). Include the following methods in your class definition. . An overloaded constructor which takes the make and model. This method throws an IllegalArgumentException if the make is "Jeep". An overloaded constructor which takes the gas tank capacity. This method throws an IllegalArgumentException if the capacity of the gas tank is not between 15 and 20 gallons (inclusive). . That's 3 constructors in total. Each constructor, once done executing, should have fully initialized all of the object's properties .a default constructor Do not implement a toString method; assume one is properly defined for you. . Do not implement any getters or setters. In java
class Truck
{
private String make, model;
private double capacity;
boolean manualTransmission;
//Default constructor
public Truck()
{
make = model = "";
capacity = 0.0;
manualTransmission = false;
}
//Overloaded constructor
public Truck(String ma, String mo)
{
if(ma.equals("Jeep"))
throw new IllegalArgumentException(Integer.toString(ma));
else
{
make = ma;
model = mo;
capacity = 0.0;
manualTransmission = false;
}
}
//Overloaded constructor
public Truck(double cap)
{
if(cap >= 15 && cap <= 20)
throw new IllegalArgumentException(Integer.toString(cap));
else
{
make = "";
model = "";
capacity = cap;
manualTransmission = false;
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

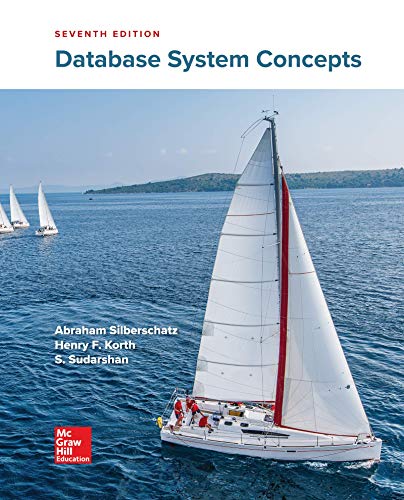
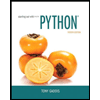
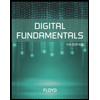
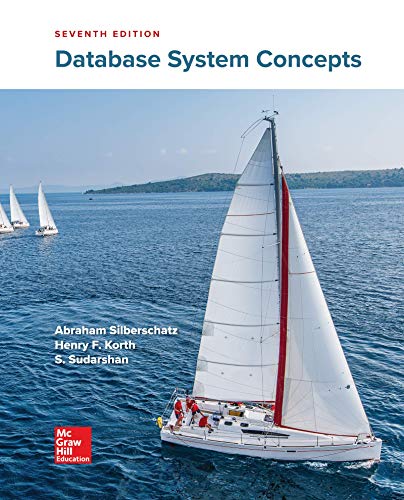
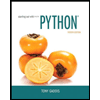
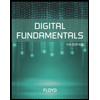
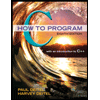
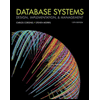
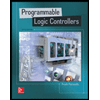