Java Code: How to implement logic for {ParseFunctionCall where all of the Node data structure correct, parses correctly, throws exceptions with good error messages? Make sure to write block of codes for these three methods.
Java Code: How to implement logic for {ParseFunctionCall where all of the Node data structure correct, parses correctly, throws exceptions with good error messages? Make sure to write block of codes for these three methods.

To implement a ParseFuctionCall method in Java that correctly parses function calls and handles exceptions with informative error messages, you can extend the existing Parser class. Below is the modified code for the Parser class that includes the ParseFuctionCall method:
1class Parser {
2 // ... Existing code ...
3
4 public Node parseFunctionCall(String code) throws ParseException {
5 if (code.matches("^\\s*(\\w+)\\s*\\(.*\\)\\s*\\{.*\\}\\s*$")) {
6 // Extract the function name and parameters
7 String functionName = code.replaceAll("^\\s*(\\w+)\\s*\\(.*\\)\\s*\\{.*\\}\\s*$", "$1");
8 String parameterList = code.replaceAll("^\\s*\\w+\\s*\\((.*)\\)\\s*\\{.*\\}\\s*$", "$1");
9 String codeBlock = code.replaceAll("^\\s*\\w+\\s*\\(.*\\)\\s*\\{(.*?)\\}\\s*$", "$1");
10
11 // Implement logic to create a 'FunctionCall' node with function name, parameters, and code block
12 Node functionCallNode = new Node("FunctionCall");
13 functionCallNode.addProperty("FunctionName", functionName);
14 functionCallNode.addProperty("Parameters", parameterList);
15 functionCallNode.addProperty("CodeBlock", codeBlock);
16 return functionCallNode;
17 } else {
18 throw new ParseException("Invalid function call: " + code);
19 }
20 }
21}
In this code, the ParseFuctionCall method checks if the input code matches the pattern of a function call. If it matches, it extracts the function name, parameters, and code block. If it doesn't match, it throws a Parse Exception with an error message.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

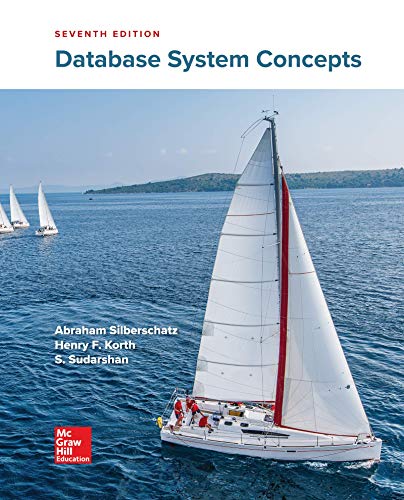
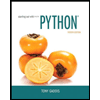
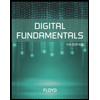
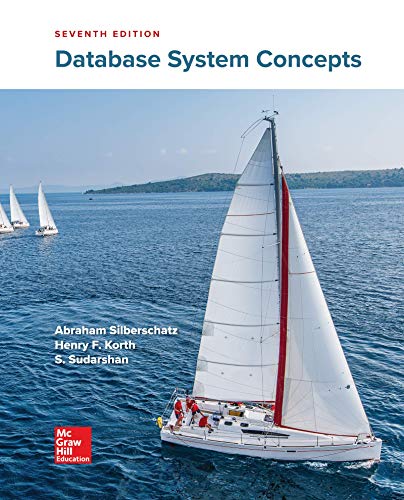
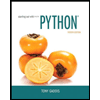
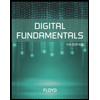
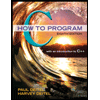
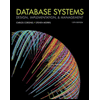
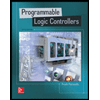