import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import org.json.JSONObject; public class Myclass { public static void main(String[] args) throws Exception { try { URL url = new URL("https://parseapi.back4app.com/classes/City?count=1&limit=10&keys=name,population"); HttpURLConnection urlConnection = (HttpURLConnection)url.openConnection(); urlConnection.setRequestProperty("X-Parse-Application-Id", "mxsebv4KoWIGkRntXwyzg6c6DhKWQuit8Ry9sHja"); // This is the fake app's application id urlConnection.setRequestProperty("X-Parse-Master-Key", "TpO0j3lG2PmEVMXlKYQACoOXKQrL3lwM0HwR9dbH"); // This is the fake app's readonly master key try { BufferedReader reader = new BufferedReader(new InputStreamReader(urlConnection.getInputStream())); StringBuilder stringBuilder = new StringBuilder(); String line; while ((line = reader.readLine()) != null) { stringBuilder.append(line); } JSONObject data = new JSONObject(stringBuilder.toString()); // Here you have the data that you need System.out.println(data.toString(2)); } finally { urlConnection.disconnect(); } } catch (Exception e) { System.out.println("Error: " + e.toString()); } } } --------------------------------------------- This is the code I have so far for part 2. Am I doing this correctly? public class city { private String name; private int population; public City (String cityName, int cityPop) { name = cityName; population = cityPop; } }
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import org.json.JSONObject;
public class Myclass {
public static void main(String[] args) throws Exception {
try {
URL url = new URL("https://parseapi.back4app.com/classes/City?count=1&limit=10&keys=name,population");
HttpURLConnection urlConnection = (HttpURLConnection)url.openConnection();
urlConnection.setRequestProperty("X-Parse-Application-Id", "mxsebv4KoWIGkRntXwyzg6c6DhKWQuit8Ry9sHja"); // This is the fake app's application id
urlConnection.setRequestProperty("X-Parse-Master-Key", "TpO0j3lG2PmEVMXlKYQACoOXKQrL3lwM0HwR9dbH"); // This is the fake app's readonly master key
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
StringBuilder stringBuilder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
}
JSONObject data = new JSONObject(stringBuilder.toString()); // Here you have the data that you need
System.out.println(data.toString(2));
} finally {
urlConnection.disconnect();
}
} catch (Exception e) {
System.out.println("Error: " + e.toString());
}
}
}
---------------------------------------------
This is the code I have so far for part 2. Am I doing this correctly?
public class city
{
private String name;
private int population;
public City (String cityName, int cityPop)
{
name = cityName;
population = cityPop;
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

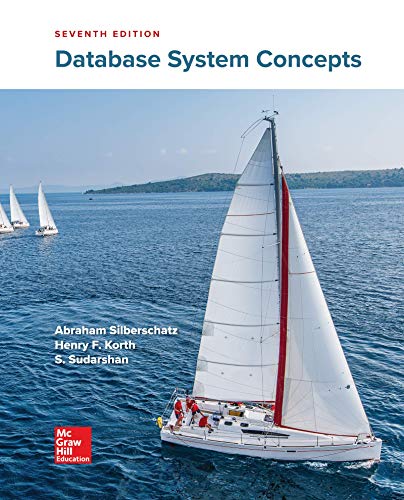
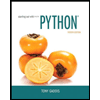
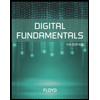
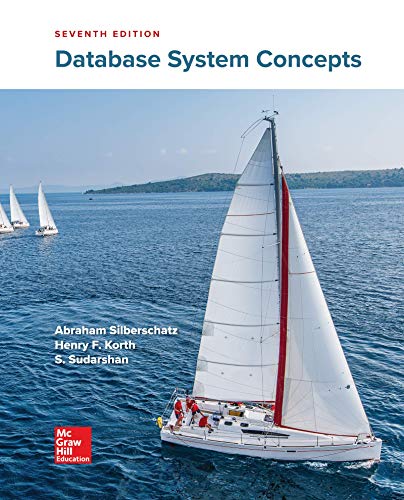
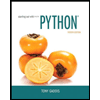
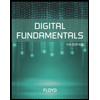
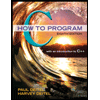
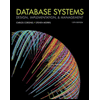
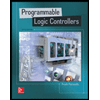