import java.util.Scanner; import java.util.HashSet; public class GroceryList { public static void main(String[] args) { Scanner scnr = new Scanner (System.in); HashSet groceries = new HashSet (); String userInput; groceries.add("milk"); groceries.add("cereal"); groceries.add("tomatoes"); groceries.add("bananas"); groceries.add("eggs"); groceries.add("ham"); userInput = scnr.nextLine(); while (userInput.compareTo ("done") != 0) { if (groceries.remove(userInput)) { System.out.println(""); } else { System.out.println(""); } userInput = scnr.nextLine(); } "1 System.out.println("Size: + groceries.size()); Input butter ham chips cereal milk done Output
import java.util.Scanner; import java.util.HashSet; public class GroceryList { public static void main(String[] args) { Scanner scnr = new Scanner (System.in); HashSet groceries = new HashSet (); String userInput; groceries.add("milk"); groceries.add("cereal"); groceries.add("tomatoes"); groceries.add("bananas"); groceries.add("eggs"); groceries.add("ham"); userInput = scnr.nextLine(); while (userInput.compareTo ("done") != 0) { if (groceries.remove(userInput)) { System.out.println(""); } else { System.out.println(""); } userInput = scnr.nextLine(); } "1 System.out.println("Size: + groceries.size()); Input butter ham chips cereal milk done Output
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![## Java Programming Exercise: Grocery List
### Programming Code:
```java
import java.util.Scanner;
import java.util.HashSet;
public class GroceryList {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
HashSet<String> groceries = new HashSet<String>();
String userInput;
groceries.add("milk");
groceries.add("cereal");
groceries.add("tomatoes");
groceries.add("bananas");
groceries.add("eggs");
groceries.add("ham");
userInput = scnr.nextLine();
while (userInput.compareTo("done") != 0) {
if (groceries.remove(userInput)) {
System.out.println("r");
} else {
System.out.println("n");
}
userInput = scnr.nextLine();
}
System.out.println("Size: " + groceries.size());
}
}
```
### Task:
Type the program's output as per the given inputs on the right.
### Explanation:
1. **Initialization & Setup:**
- Import `Scanner` and `HashSet` from Java utilities.
- Define a public class named `GroceryList`.
2. **Main Method:**
- Create a `Scanner` object (`scnr`) for user input.
- Create a `HashSet` named `groceries` to store the grocery items.
- Add items "milk", "cereal", "tomatoes", "bananas", "eggs", and "ham" to the `groceries` set.
3. **User Input and Processing:**
- Read the first line of user input.
- Use a while loop to continue reading inputs until the user types "done".
- For each input, check if the item is in the `groceries` set:
- If the item is found in the set and is successfully removed, print "r".
- If the item is not found in the set, print "n".
4. **Final Output:**
- After exiting the loop, print the size of the remaining items in the `groceries` set.
### Input and Expected Output:
**Input:**
```
butter
ham
chips
cereal
milk
done
```
**Output:**
```
n
r
n
r
r
Size: 3
```
### Diagram:
#### Input Box:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F92ef1733-4d13-4c5f-b698-2777de49beac%2F59deedaf-e083-498d-9114-8cdb2b28e3c3%2Fks8eog_processed.png&w=3840&q=75)
Transcribed Image Text:## Java Programming Exercise: Grocery List
### Programming Code:
```java
import java.util.Scanner;
import java.util.HashSet;
public class GroceryList {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
HashSet<String> groceries = new HashSet<String>();
String userInput;
groceries.add("milk");
groceries.add("cereal");
groceries.add("tomatoes");
groceries.add("bananas");
groceries.add("eggs");
groceries.add("ham");
userInput = scnr.nextLine();
while (userInput.compareTo("done") != 0) {
if (groceries.remove(userInput)) {
System.out.println("r");
} else {
System.out.println("n");
}
userInput = scnr.nextLine();
}
System.out.println("Size: " + groceries.size());
}
}
```
### Task:
Type the program's output as per the given inputs on the right.
### Explanation:
1. **Initialization & Setup:**
- Import `Scanner` and `HashSet` from Java utilities.
- Define a public class named `GroceryList`.
2. **Main Method:**
- Create a `Scanner` object (`scnr`) for user input.
- Create a `HashSet` named `groceries` to store the grocery items.
- Add items "milk", "cereal", "tomatoes", "bananas", "eggs", and "ham" to the `groceries` set.
3. **User Input and Processing:**
- Read the first line of user input.
- Use a while loop to continue reading inputs until the user types "done".
- For each input, check if the item is in the `groceries` set:
- If the item is found in the set and is successfully removed, print "r".
- If the item is not found in the set, print "n".
4. **Final Output:**
- After exiting the loop, print the size of the remaining items in the `groceries` set.
### Input and Expected Output:
**Input:**
```
butter
ham
chips
cereal
milk
done
```
**Output:**
```
n
r
n
r
r
Size: 3
```
### Diagram:
#### Input Box:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
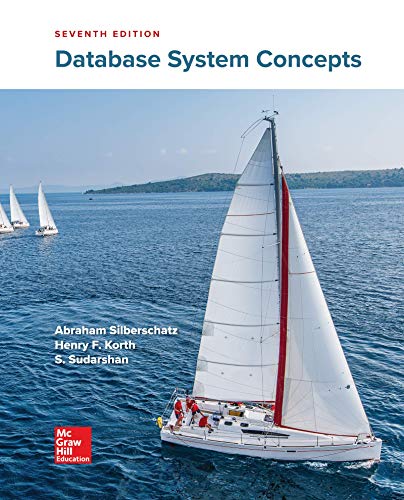
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
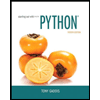
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
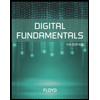
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
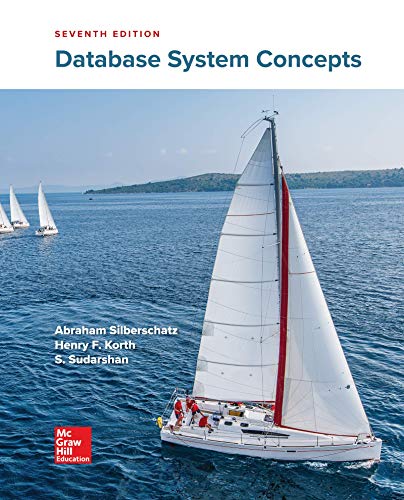
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
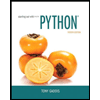
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
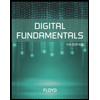
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
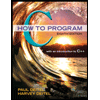
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
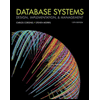
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
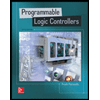
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education