X1240: HashTable for Student ID The student ID at a fictitious university encodes various pieces of information. The ID has the format 8YY-MMM-#### where YY is the year of admission, MMM is a code for the college of application, and #### is the sequential numeric order of processing. For example, a student with ID 822-123-0250 was admitted to the university in '22 into the college 123 and was the 250th admission processed that year. Given a string in this format, convert the string into a new string such as YY#### . Then use that key to store the full ID into a hashmap. Write a method that stores into the admissions hash map all of the recoded IDs. Remember, you add words to a HashMap by calling the put() method admissions.put( <>, <>). This admissions table is stored in a HashMap where the key is the converted student ID, and the value is the full representation for the student ID. So for the example above would result in an entry such as: admissions.put("220250", "822-123-0250") The solution looks like this:
X1240: HashTable for Student ID The student ID at a fictitious university encodes various pieces of information. The ID has the format 8YY-MMM-#### where YY is the year of admission, MMM is a code for the college of application, and #### is the sequential numeric order of processing. For example, a student with ID 822-123-0250 was admitted to the university in '22 into the college 123 and was the 250th admission processed that year. Given a string in this format, convert the string into a new string such as YY#### . Then use that key to store the full ID into a hashmap. Write a method that stores into the admissions hash map all of the recoded IDs. Remember, you add words to a HashMap by calling the put() method admissions.put( <>, <>). This admissions table is stored in a HashMap where the key is the converted student ID, and the value is the full representation for the student ID. So for the example above would result in an entry such as: admissions.put("220250", "822-123-0250") The solution looks like this:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Code to start with:
public void addStudents(String[] ids)
{
}
![**X1240: HashTable for Student ID**
The student ID at a fictitious university encodes various pieces of information. The ID has the format **8YY-MMM-####** where YY is the year of admission, MMM is a code for the college of application, and #### is the sequential numeric order of processing. For example, a student with ID 822-123-0250 was admitted to the university in ’22 into the college 123 and was the 250th admission processed that year.
Given a string in this format, convert the string into a new string such as **YY####**. Then use that key to store the full ID into a hashmap.
Write a method that stores into the `admissions` hash map all of the recoded IDs. Remember, you add words to a HashMap by calling the put() method `admissions.put( <encodedID>, <fullID> )`.
This `admissions` table is stored in a `HashMap<String, String>` where the key is the converted student ID, and the value is the full representation for the student ID. So for the example above would result in an entry such as:
```
admissions.put("220250", "822-123-0250")
```
The solution looks like this:
```java
void addStudents(String[] ids)
{
<<loop over all ids>>
<<convert id to YY#### format>>
<<put convertedID and originalID into admissions>>
}
```
In the provided code snippet, it outlines the basic structure for creating a hashmap and storing transformed student IDs alongside their full representations.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb6b9b709-ecc4-405f-be15-0cfcda40f349%2F16ec82d8-d08f-4080-8dda-0f1f3624a1d4%2Fba43x8u_processed.png&w=3840&q=75)
Transcribed Image Text:**X1240: HashTable for Student ID**
The student ID at a fictitious university encodes various pieces of information. The ID has the format **8YY-MMM-####** where YY is the year of admission, MMM is a code for the college of application, and #### is the sequential numeric order of processing. For example, a student with ID 822-123-0250 was admitted to the university in ’22 into the college 123 and was the 250th admission processed that year.
Given a string in this format, convert the string into a new string such as **YY####**. Then use that key to store the full ID into a hashmap.
Write a method that stores into the `admissions` hash map all of the recoded IDs. Remember, you add words to a HashMap by calling the put() method `admissions.put( <encodedID>, <fullID> )`.
This `admissions` table is stored in a `HashMap<String, String>` where the key is the converted student ID, and the value is the full representation for the student ID. So for the example above would result in an entry such as:
```
admissions.put("220250", "822-123-0250")
```
The solution looks like this:
```java
void addStudents(String[] ids)
{
<<loop over all ids>>
<<convert id to YY#### format>>
<<put convertedID and originalID into admissions>>
}
```
In the provided code snippet, it outlines the basic structure for creating a hashmap and storing transformed student IDs alongside their full representations.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
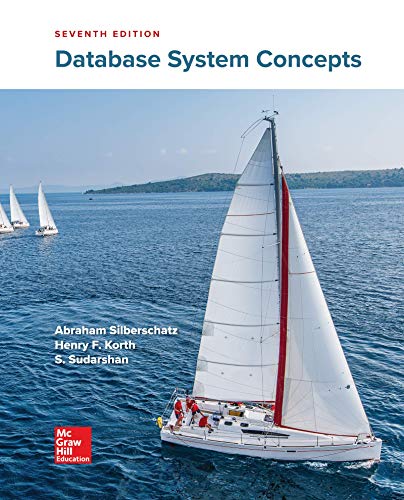
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
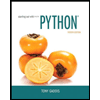
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
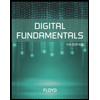
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
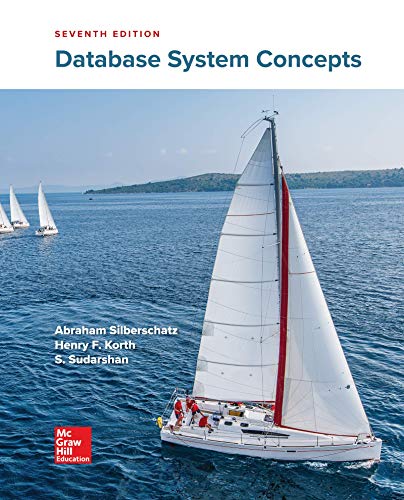
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
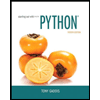
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
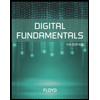
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
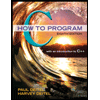
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
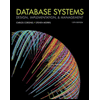
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
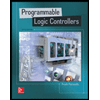
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education