In netbeans using Java create a class Palindrome2 which replaces the while loop of problem 13 with a for loop.
In netbeans using Java create a class Palindrome2 which replaces the while loop of problem 13 with a for loop.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In netbeans using Java create a class Palindrome2 which replaces the while loop of problem 13 with a for loop.
![```java
package lab9;
import java.util.Scanner;
public class Palindrome1
{
public static void main(String[] args)
{
// Create a Scanner
Scanner input = new Scanner(System.in);
// Prompt the user to enter a string
System.out.print("Enter a string: ");
String s = input.nextLine();
// The index of the first character in the string
int low = 0;
// The index of the last character in the string
int high = s.length() - 1;
boolean isPalindrome = true;
while (low < high)
{
if (s.charAt(low) != s.charAt(high))
{
isPalindrome = false;
break;
}
low++;
high--;
}
if (isPalindrome)
{
System.out.println(s + " is a palindrome");
}
else
{
System.out.println(s + " is not a palindrome");
}
}
}
```
### Explanation
The provided Java program checks if the input string is a palindrome. A palindrome is a sequence of characters that reads the same backward as forward.
1. **Imports**: The program utilizes `java.util.Scanner` to obtain user input.
2. **Class and Method Definition**:
- The `Palindrome1` class contains the `main` method where the execution begins.
3. **Scanner for Input**:
- A `Scanner` object named `input` is created to capture user input from the console.
4. **User Prompt**:
- The user is prompted to enter a string.
5. **Index Initialization**:
- `low` is initialized to 0, representing the first character in the string.
- `high` is initialized to `s.length() - 1`, representing the last character in the string.
6. **Palindrome Check**:
- A `boolean` variable `isPalindrome` is set to `true` initially.
- A `while` loop iterates as long as `low < high`.
- Inside the loop, characters at positions `low` and `high` are compared.
- If they differ, `isPalindrome` is set to `false` and the loop is terminated.
- If they are the same, `low` is incremented, and `high` is decremented to further check inward pairs.
7](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4b17bd5b-54f9-4eb4-8579-a760e9fbe7f9%2Fe96e9c7a-2ddd-4574-ae01-f30258f904e3%2Fc8bpd9k_processed.png&w=3840&q=75)
Transcribed Image Text:```java
package lab9;
import java.util.Scanner;
public class Palindrome1
{
public static void main(String[] args)
{
// Create a Scanner
Scanner input = new Scanner(System.in);
// Prompt the user to enter a string
System.out.print("Enter a string: ");
String s = input.nextLine();
// The index of the first character in the string
int low = 0;
// The index of the last character in the string
int high = s.length() - 1;
boolean isPalindrome = true;
while (low < high)
{
if (s.charAt(low) != s.charAt(high))
{
isPalindrome = false;
break;
}
low++;
high--;
}
if (isPalindrome)
{
System.out.println(s + " is a palindrome");
}
else
{
System.out.println(s + " is not a palindrome");
}
}
}
```
### Explanation
The provided Java program checks if the input string is a palindrome. A palindrome is a sequence of characters that reads the same backward as forward.
1. **Imports**: The program utilizes `java.util.Scanner` to obtain user input.
2. **Class and Method Definition**:
- The `Palindrome1` class contains the `main` method where the execution begins.
3. **Scanner for Input**:
- A `Scanner` object named `input` is created to capture user input from the console.
4. **User Prompt**:
- The user is prompted to enter a string.
5. **Index Initialization**:
- `low` is initialized to 0, representing the first character in the string.
- `high` is initialized to `s.length() - 1`, representing the last character in the string.
6. **Palindrome Check**:
- A `boolean` variable `isPalindrome` is set to `true` initially.
- A `while` loop iterates as long as `low < high`.
- Inside the loop, characters at positions `low` and `high` are compared.
- If they differ, `isPalindrome` is set to `false` and the loop is terminated.
- If they are the same, `low` is incremented, and `high` is decremented to further check inward pairs.
7
Expert Solution

Step 1
For Loop
Programmers use the for loop, a conditional iterative statement, to check for specific criteria and then continually run a piece of code as long as those requirements are satisfied.
Step by step
Solved in 3 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
In netbeans using Java create a class Palindrome2 The class uses a for loop to determine whether a word (string) entered by the user is a palindrome.
![```java
package lab9;
import java.util.Scanner;
public class Palindrome1
{
public static void main(String[] args)
{
// Create a Scanner
Scanner input = new Scanner(System.in);
// Prompt the user to enter a string
System.out.print("Enter a string: ");
String s = input.nextLine();
// The index of the first character in the string
int low = 0;
// The index of the last character in the string
int high = s.length() - 1;
boolean isPalindrome = true;
while (low < high)
{
if (s.charAt(low) != s.charAt(high))
{
isPalindrome = false;
break;
}
low++;
high--;
}
if (isPalindrome)
{
System.out.println(s + " is a palindrome");
}
else
{
System.out.println(s + " is not a palindrome");
}
}
}
```
### Explanation:
This Java program checks if a given string is a palindrome. A palindrome is a word, phrase, or sequence that reads the same forward and backward (ignoring spaces, punctuation, and capitalization). Here’s a detailed explanation of the code:
1. **Package Declaration:**
- `package lab9;` - The code is part of the `lab9` package.
2. **Import Statement:**
- `import java.util.Scanner;` - This imports the `Scanner` class, which is used to obtain user input.
3. **Class Declaration:**
- `public class Palindrome1` - The main class named `Palindrome1`.
4. **Main Method:**
- `public static void main(String[] args)` - The entry point of the program.
5. **Scanner Creation:**
- `Scanner input = new Scanner(System.in);` - Creates a `Scanner` object to get input from the user.
6. **User Prompt and Input:**
- `System.out.print("Enter a string: ");` - Prompts the user to enter a string.
- `String s = input.nextLine();` - Reads the entire line of input from the user and stores it in the string `s`.
7. **Index Initialization:**
- `int low = 0;` - Initializes the index for the first character of the string.
-](https://content.bartleby.com/qna-images/question/4b17bd5b-54f9-4eb4-8579-a760e9fbe7f9/fa3bae28-dd6e-4750-97aa-8aac5c1d7090/66kr87b_thumbnail.png)
Transcribed Image Text:```java
package lab9;
import java.util.Scanner;
public class Palindrome1
{
public static void main(String[] args)
{
// Create a Scanner
Scanner input = new Scanner(System.in);
// Prompt the user to enter a string
System.out.print("Enter a string: ");
String s = input.nextLine();
// The index of the first character in the string
int low = 0;
// The index of the last character in the string
int high = s.length() - 1;
boolean isPalindrome = true;
while (low < high)
{
if (s.charAt(low) != s.charAt(high))
{
isPalindrome = false;
break;
}
low++;
high--;
}
if (isPalindrome)
{
System.out.println(s + " is a palindrome");
}
else
{
System.out.println(s + " is not a palindrome");
}
}
}
```
### Explanation:
This Java program checks if a given string is a palindrome. A palindrome is a word, phrase, or sequence that reads the same forward and backward (ignoring spaces, punctuation, and capitalization). Here’s a detailed explanation of the code:
1. **Package Declaration:**
- `package lab9;` - The code is part of the `lab9` package.
2. **Import Statement:**
- `import java.util.Scanner;` - This imports the `Scanner` class, which is used to obtain user input.
3. **Class Declaration:**
- `public class Palindrome1` - The main class named `Palindrome1`.
4. **Main Method:**
- `public static void main(String[] args)` - The entry point of the program.
5. **Scanner Creation:**
- `Scanner input = new Scanner(System.in);` - Creates a `Scanner` object to get input from the user.
6. **User Prompt and Input:**
- `System.out.print("Enter a string: ");` - Prompts the user to enter a string.
- `String s = input.nextLine();` - Reads the entire line of input from the user and stores it in the string `s`.
7. **Index Initialization:**
- `int low = 0;` - Initializes the index for the first character of the string.
-
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
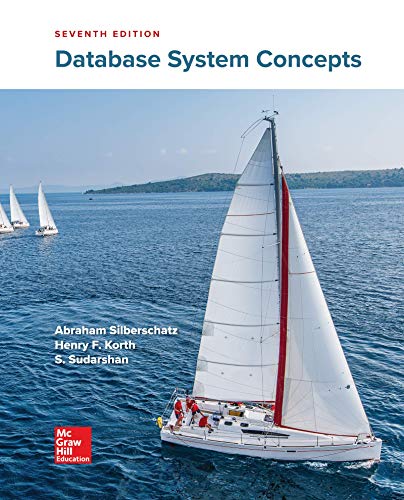
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
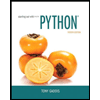
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
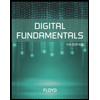
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
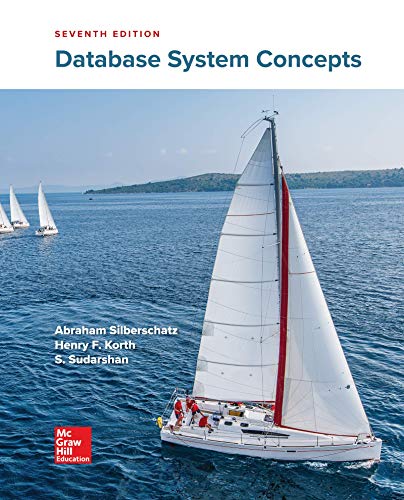
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
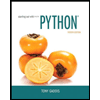
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
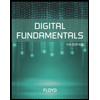
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
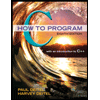
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
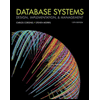
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
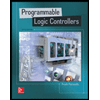
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education