Here is my code in Java: import java.util.Scanner; import java.util.HashMap; public class Inventory { /** This class demonstrates the inventory */ //Declarations Of inventory static final int LightBulb60W = 1; static final int LightBulb100W = 2; static final int BoltM5 = 3; static final int BoltM8 = 4; static final int Hose25 = 5; static final int Hose50 = 6; public static void main (String [] args) { //Scanner Object @SuppressWarnings("resource") Scanner keyboard = new Scanner(System.in); //Variables int month; int day; int year; double units; double cost = 0; //Declarations int total; HashMap productMap = new HashMap<>(); productMap.put(1,"Light Bulb 60W"); productMap.put(2,"Light Bulb 100W"); productMap.put(3,"Bolt M5"); productMap.put(4,"Bolt M8"); productMap.put(5,"Hose 25 feet"); productMap.put(6,"Hose 50 feet 60W"); while(true){ //Prints out inventory System.out.println("The Inventory: "); System.out.println("1 Light Bulb 60W 3 dollars 0 cents X"); System.out.println("2 Light Bulb 100W 5 dollars 99 cents X"); System.out.println("3 Bolt M5 0 dollars 15 cents Y"); System.out.println("4 Bolt M8 0 dollars 25 cents Y"); System.out.println("5 Hose 25 feet 10 dollars 0 cents Z"); System.out.println("6 Hose 50 feet 15 dollars 0 cents Z\n"); //Prints out desirable item number System.out.print("Which item number from the list do you desire?\n"); System.out.print("1, 2, 3, 4, 5 or 6?\n"); total = keyboard.nextInt(); System.out.print("Your choice: " + total + "\n"); // Units System.out.print("How many units do you desire?\n"); units = keyboard.nextInt(); System.out.print("Number of units: "); System.out.print("When do you plan your purchase?\n"); System.out.print("Month of purchase (mm): "); month = keyboard.nextInt(); System.out.print("Day of purchase (dd): "); day = keyboard.nextInt(); System.out.print("Year of purchase (yyyy): "); year = keyboard.nextInt(); System.out.print("Date: " + month + day + year + "\n"); // Switch Statement switch(total) { case(LightBulb60W): cost = 3.00; break; case(LightBulb100W): cost = 5.99; break; case(BoltM5): cost = 0.15; break; case(BoltM8): cost = 0.25; break; case(Hose25): cost = 10; break; case(Hose50): cost = 15; break; } System.out.println(total); System.out.print("Your total for " + productMap.get(new Integer(total)) + " is: " + "$" + cost * units + "\n"); // While Loop @SuppressWarnings("resource") Scanner scan = new Scanner(System.in); System.out.println("Do you want to continue to loop? (y/n)"); String s = scan.next(); while ( ! s.equals("n") ) { System.out.println("Do you want to continue to loop? (y/n)"); s = scan.next(); if (s == "n") break; } } } }
Here is my code in Java: import java.util.Scanner; import java.util.HashMap; public class Inventory { /** This class demonstrates the inventory */ //Declarations Of inventory static final int LightBulb60W = 1; static final int LightBulb100W = 2; static final int BoltM5 = 3; static final int BoltM8 = 4; static final int Hose25 = 5; static final int Hose50 = 6; public static void main (String [] args) { //Scanner Object @SuppressWarnings("resource") Scanner keyboard = new Scanner(System.in); //Variables int month; int day; int year; double units; double cost = 0; //Declarations int total; HashMap productMap = new HashMap<>(); productMap.put(1,"Light Bulb 60W"); productMap.put(2,"Light Bulb 100W"); productMap.put(3,"Bolt M5"); productMap.put(4,"Bolt M8"); productMap.put(5,"Hose 25 feet"); productMap.put(6,"Hose 50 feet 60W"); while(true){ //Prints out inventory System.out.println("The Inventory: "); System.out.println("1 Light Bulb 60W 3 dollars 0 cents X"); System.out.println("2 Light Bulb 100W 5 dollars 99 cents X"); System.out.println("3 Bolt M5 0 dollars 15 cents Y"); System.out.println("4 Bolt M8 0 dollars 25 cents Y"); System.out.println("5 Hose 25 feet 10 dollars 0 cents Z"); System.out.println("6 Hose 50 feet 15 dollars 0 cents Z\n"); //Prints out desirable item number System.out.print("Which item number from the list do you desire?\n"); System.out.print("1, 2, 3, 4, 5 or 6?\n"); total = keyboard.nextInt(); System.out.print("Your choice: " + total + "\n"); // Units System.out.print("How many units do you desire?\n"); units = keyboard.nextInt(); System.out.print("Number of units: "); System.out.print("When do you plan your purchase?\n"); System.out.print("Month of purchase (mm): "); month = keyboard.nextInt(); System.out.print("Day of purchase (dd): "); day = keyboard.nextInt(); System.out.print("Year of purchase (yyyy): "); year = keyboard.nextInt(); System.out.print("Date: " + month + day + year + "\n"); // Switch Statement switch(total) { case(LightBulb60W): cost = 3.00; break; case(LightBulb100W): cost = 5.99; break; case(BoltM5): cost = 0.15; break; case(BoltM8): cost = 0.25; break; case(Hose25): cost = 10; break; case(Hose50): cost = 15; break; } System.out.println(total); System.out.print("Your total for " + productMap.get(new Integer(total)) + " is: " + "$" + cost * units + "\n"); // While Loop @SuppressWarnings("resource") Scanner scan = new Scanner(System.in); System.out.println("Do you want to continue to loop? (y/n)"); String s = scan.next(); while ( ! s.equals("n") ) { System.out.println("Do you want to continue to loop? (y/n)"); s = scan.next(); if (s == "n") break; } } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Here is my code in Java:
import java.util.Scanner;
import java.util.HashMap;
public class Inventory {
/**
This class demonstrates the inventory
*/
//Declarations Of inventory
static final int LightBulb60W = 1;
static final int LightBulb100W = 2;
static final int BoltM5 = 3;
static final int BoltM8 = 4;
static final int Hose25 = 5;
static final int Hose50 = 6;
public static void main (String [] args)
{
//Scanner Object
@SuppressWarnings("resource")
Scanner keyboard = new Scanner(System.in);
//Variables
int month;
int day;
int year;
double units;
double cost = 0;
//Declarations
int total;
HashMap<Integer, String> productMap = new HashMap<>();
productMap.put(1,"Light Bulb 60W");
productMap.put(2,"Light Bulb 100W");
productMap.put(3,"Bolt M5");
productMap.put(4,"Bolt M8");
productMap.put(5,"Hose 25 feet");
productMap.put(6,"Hose 50 feet 60W");
while(true){
//Prints out inventory
System.out.println("The Inventory: ");
System.out.println("1 Light Bulb 60W 3 dollars 0 cents X");
System.out.println("2 Light Bulb 100W 5 dollars 99 cents X");
System.out.println("3 Bolt M5 0 dollars 15 cents Y");
System.out.println("4 Bolt M8 0 dollars 25 cents Y");
System.out.println("5 Hose 25 feet 10 dollars 0 cents Z");
System.out.println("6 Hose 50 feet 15 dollars 0 cents Z\n");
//Prints out desirable item number
System.out.print("Which item number from the list do you desire?\n");
System.out.print("1, 2, 3, 4, 5 or 6?\n");
total = keyboard.nextInt();
System.out.print("Your choice: " + total + "\n");
// Units
System.out.print("How many units do you desire?\n");
units = keyboard.nextInt();
System.out.print("Number of units: ");
System.out.print("When do you plan your purchase?\n");
System.out.print("Month of purchase (mm): ");
month = keyboard.nextInt();
System.out.print("Day of purchase (dd): ");
day = keyboard.nextInt();
System.out.print("Year of purchase (yyyy): ");
year = keyboard.nextInt();
System.out.print("Date: " + month + day + year + "\n");
// Switch Statement
switch(total) {
case(LightBulb60W):
cost = 3.00;
break;
case(LightBulb100W):
cost = 5.99;
break;
case(BoltM5):
cost = 0.15;
break;
case(BoltM8):
cost = 0.25;
break;
case(Hose25):
cost = 10;
break;
case(Hose50):
cost = 15;
break;
}
System.out.println(total);
System.out.print("Your total for " + productMap.get(new Integer(total)) + " is: " + "$" + cost * units + "\n");
// While Loop
@SuppressWarnings("resource")
Scanner scan = new Scanner(System.in);
System.out.println("Do you want to continue to loop? (y/n)");
String s = scan.next();
while ( ! s.equals("n") )
{
System.out.println("Do you want to continue to loop? (y/n)");
s = scan.next();
if (s == "n")
break;
}
}
}
}

Transcribed Image Text:Thus, the first thing to do is create an abstract superclass and three subclasses. The superclass must
have as many members (fields and methods) common to type X, Y, and Z products as possible. Each
subclass must have its constructor and a method to calculate the total that overrides the superclass's
method.
The advantage of inheritance is the ability to create an array of superclass objects to be filled with
objects of different subclasses. Thus, your inventory is still in one array. Use the product's index in the
array to build the product's ID (I would recommend starting with 1). In the superclass, create a static
variable that holds the number of products available in the inventory. It must grow as reading from the
file progresses.
Override a default tostring () method for a superclass such that it shows product ID, name, regular
price, and type.
At this moment, make sure that all modifications you have made keep your application alive and
working.
The next step is to add three new classes to the project:
Create a class to represent the user - hardware store customer. You must decide what members
this class needs. Ask the user to input necessary information.
You may notice that the date is a complicated piece of data. Create a class Date that converts a
string of the form of "mm/dd/yyyy" into an object with three fields – month, day, and year. The
class constructor may do this job, but do not forget to check the string for correctness. Once you
have this class ready, input the date as a string with the following converting into an object of
type Date. Remember, if you read a string from the keyboard after a number, you may need to
handle the new line symbol left in the input buffer.
The result of your application is an order that must have the customer's information, the date,
the product information, and the total amount to pay. Thus, the third class to add is the class
Order. This class aggregates other classes. When all the information is collected, the order is
ready to be created and printed out. Outputting the order is the primary behavior of an object
of type Order, and it is the final step of your application. Thus, class Order aggregates the
customer's info, date, product info, and total. Please note that type X and type Y products need
only regular price and amount to calculate the total, while type Z products must also consider
the date.

Transcribed Image Text:The input and output examples for types Y and Z are
User: Vladimir Kuperman
The inventory:
1 Light Bulb 60W 3.0
2 Light Bulb 100W 5.99
0.15
0.25
Туре X
Туре х
Туре Y
Туре Y
Туре Z
Туре z
3 Steel Bolt M5
4 Steel Bolt M8
5 Hose 25 feet
10.0
6 Hose 50 feet
15.0
Your choice: 3
Number of units: 1000
What is the purchasing date? (mm/dd/yyyy)
12/12/2022
***
Date 12/12/2022
Vladimir Kuperman
Your item:
+----+-
+----+
|ID
| Name
|Price |Type|
+----+--
----+----+
13
Steel Bolt M5
0.15
Y
+----+
in the amount of 1000
*If you buy more than 1500 units you would pay $0.14 per unit.
****
Your total: 127 dollars 5 cents
Save to file? (Y/N) Y
User: Vladimir Kuperman
The inventory:
1 Light Bulb 60W
2 Light Bulb 100W 5.99
3 Steel Bolt M5
Туре X
Туре х
Туре Y
Туре Y
Туре 2
Туре Z
3.0
0.15
4 Steel Bolt M8
0.25
5 Hose 25 feet
10.0
6 Hose 50 feet
15.0
Your choice: 5
Number of units: 10
What is the purchasing date? (mm/dd/yyyy)
10/12/2022
***
*********
Date 10/12/2022
****
Vladimir Kuperman
Your item:
+----+-
+----+
|ID
| Name
|Price |Type|
+----+
-----+
----
15
Hose 25 feet
10.0
+----+
in the amount of 10
Your total: 70 dollars 0 cents
Save to file? (Y/N) Y
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
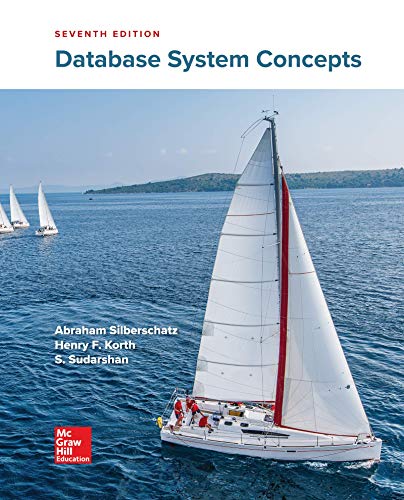
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
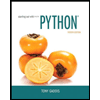
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
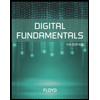
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
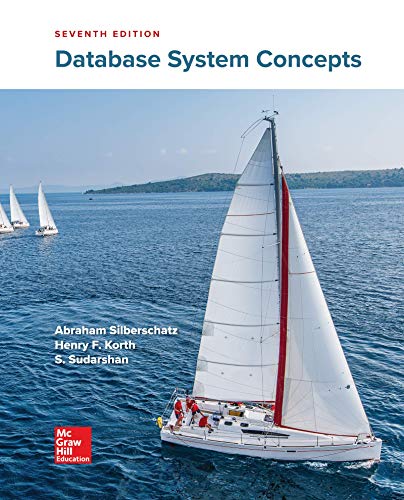
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
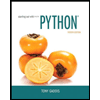
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
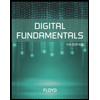
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
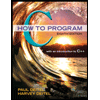
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
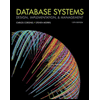
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
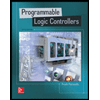
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education