The picture is code tester public class WordVowels { // Step 2: Declare an instance variable to store the text // Step 3: Write the following constructor including // possible Javadoc tags /** * Constructs a Word object by initializing the * instance variable to the passed in parameter. * */ // Step 4: Write method getVowels() including // possible Javadoc tags /** * Gets all vowels of the stored text and returns * "" if the stored test does not have any vowels. * */ // Step 5: Write method vowelsFirst() including // possible Javadoc tags /** * Gets a string containing all vowels of the stored text * followed by all other chars of the stored text. * */ // Step 6: Write method longestVowelGroup() including // possible Javadoc tags /** * Gets the longest vowel group and returns "" * if the stored text does not have any vowels. * */ /** * Determines whether a char is a vowel. * * @param letter a char * @return true if the char is a vowel * false otherwise */ private boolean isVowel(char letter) { String vowels = "aeiou"; String str = "" + letter; return vowels.contains(str.toLowerCase()); } /** * Gets the stored text. * * @return the stored text */ @Override public String toString() { return text; } }
The picture is code tester public class WordVowels { // Step 2: Declare an instance variable to store the text // Step 3: Write the following constructor including // possible Javadoc tags /** * Constructs a Word object by initializing the * instance variable to the passed in parameter. * */ // Step 4: Write method getVowels() including // possible Javadoc tags /** * Gets all vowels of the stored text and returns * "" if the stored test does not have any vowels. * */ // Step 5: Write method vowelsFirst() including // possible Javadoc tags /** * Gets a string containing all vowels of the stored text * followed by all other chars of the stored text. * */ // Step 6: Write method longestVowelGroup() including // possible Javadoc tags /** * Gets the longest vowel group and returns "" * if the stored text does not have any vowels. * */ /** * Determines whether a char is a vowel. * * @param letter a char * @return true if the char is a vowel * false otherwise */ private boolean isVowel(char letter) { String vowels = "aeiou"; String str = "" + letter; return vowels.contains(str.toLowerCase()); } /** * Gets the stored text. * * @return the stored text */ @Override public String toString() { return text; } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
The picture is code tester
public class WordVowels
{
// Step 2: Declare an instance variable to store the text
// Step 3: Write the following constructor including
// possible Javadoc tags
/**
* Constructs a Word object by initializing the
* instance variable to the passed in parameter.
*
*/
// Step 4: Write method getVowels() including
// possible Javadoc tags
/**
* Gets all vowels of the stored text and returns
* "" if the stored test does not have any vowels.
*
*/
// Step 5: Write method vowelsFirst() including
// possible Javadoc tags
/**
* Gets a string containing all vowels of the stored text
* followed by all other chars of the stored text.
*
*/
// Step 6: Write method longestVowelGroup() including
// possible Javadoc tags
/**
* Gets the longest vowel group and returns ""
* if the stored text does not have any vowels.
*
*/
/**
* Determines whether a char is a vowel.
*
* @param letter a char
* @return true if the char is a vowel
* false otherwise
*/
private boolean isVowel(char letter)
{
String vowels = "aeiou";
String str = "" + letter;
return vowels.contains(str.toLowerCase());
}
/**
* Gets the stored text.
*
* @return the stored text
*/
@Override
public String toString()
{
return text;
}
}
![{
public static void main(String[] args)
{
WordVowels wordVowels1 = new WordVowels ("monopoly");
WordVowels wordVowels2 = new WordVowels("outrageous");
WordVowels wordVowels3 = new WordVowels("CS 46A");
WordVowels wordVowels4 = new WordVowels("CS 46B");
WordVowels wordVowels5 = new WordVowels ("Introduction to Programming");
10
11
12
13
14
15
16
// TTesting method getVowels()
System.out.println("Vowels of \"" + wordVowels1 + "\": " + wordVowels1.getVowels ());
System.out.println("Expected: 000");
System.out.println("Vowels of \"" + wordVowels2 + "\": " + wordVowels2.getVowels());
System.out.println("Expected: ouaeou");
System.out.println("Vowels of \"" + wordVowels3 + "\": " + wordVowels3.getVowels());
System.out.println("Expected: A");
System.out.println("Vowels of \"" + wordVowels4 + "\": " + wordVowels4.getVowels());
System.out.println("Expected: ");
System.out.println("Vowels of \"" + wordVowels5 + "\": " + wordVowels5.getVowels());
System.out.println("Expected: Iouioooai");
17
18
19
20
21
22
23
24
25
%3D
26
27
28
// Testing method vowelsFirst()
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels1, wordVowels1.vowelsFirst());
System.out.println("Expected: ooomnply");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels2, wordVowels2.vowelsFirst());
System.out.println("Expected: ouaeoutrgs");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels3, wordVowels3. vowelsFirst());
System.out.println("Expected: ACS 46");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels4, wordVowels4.vowelsFirst());
System.out.println("Expected: CS 46B");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels5, wordVowels5. vowelsFirst());
System.out.println("Expected: Iouioooaintrdctn t Prgrmmng");
29
30
31
32
33
34
35
36
37
38
39
40
// Testing method longestVowelGroup()
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels1, wordVowels1. longestVowelIGra
System.out.println("Expected: o");
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels2, wordVowels2. longestVowelGrou
System.out.println("Expected: eou");
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels3, wordVowels3. longestVowe lGrou
System.out.printin("Expected: A");
System.out.printf("Longest vowel group of \"%s\": %S. %n", wordVowels4, wordVowels4. longestVowelGrou
System.out.printin("Expected: ");
System.out.printf("Longest vowel group of \"%s\": %S.%n", wordVowels5, wordVowels5.
O 0 A1
41
42
43
44
45
46
47
48
49
50
ngestVowelGroup
O Restricted Mode
MacBook Air
80
888
000
esc
F1
F2
F3
F4
F5
F6
F7
!
#
$
&
@](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F41fc4194-ca6a-4c74-975f-c41e6cb486ed%2F08ef44c4-e8bb-4b46-a497-032683c464ea%2Fex4e6r_processed.jpeg&w=3840&q=75)
Transcribed Image Text:{
public static void main(String[] args)
{
WordVowels wordVowels1 = new WordVowels ("monopoly");
WordVowels wordVowels2 = new WordVowels("outrageous");
WordVowels wordVowels3 = new WordVowels("CS 46A");
WordVowels wordVowels4 = new WordVowels("CS 46B");
WordVowels wordVowels5 = new WordVowels ("Introduction to Programming");
10
11
12
13
14
15
16
// TTesting method getVowels()
System.out.println("Vowels of \"" + wordVowels1 + "\": " + wordVowels1.getVowels ());
System.out.println("Expected: 000");
System.out.println("Vowels of \"" + wordVowels2 + "\": " + wordVowels2.getVowels());
System.out.println("Expected: ouaeou");
System.out.println("Vowels of \"" + wordVowels3 + "\": " + wordVowels3.getVowels());
System.out.println("Expected: A");
System.out.println("Vowels of \"" + wordVowels4 + "\": " + wordVowels4.getVowels());
System.out.println("Expected: ");
System.out.println("Vowels of \"" + wordVowels5 + "\": " + wordVowels5.getVowels());
System.out.println("Expected: Iouioooai");
17
18
19
20
21
22
23
24
25
%3D
26
27
28
// Testing method vowelsFirst()
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels1, wordVowels1.vowelsFirst());
System.out.println("Expected: ooomnply");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels2, wordVowels2.vowelsFirst());
System.out.println("Expected: ouaeoutrgs");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels3, wordVowels3. vowelsFirst());
System.out.println("Expected: ACS 46");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels4, wordVowels4.vowelsFirst());
System.out.println("Expected: CS 46B");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels5, wordVowels5. vowelsFirst());
System.out.println("Expected: Iouioooaintrdctn t Prgrmmng");
29
30
31
32
33
34
35
36
37
38
39
40
// Testing method longestVowelGroup()
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels1, wordVowels1. longestVowelIGra
System.out.println("Expected: o");
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels2, wordVowels2. longestVowelGrou
System.out.println("Expected: eou");
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels3, wordVowels3. longestVowe lGrou
System.out.printin("Expected: A");
System.out.printf("Longest vowel group of \"%s\": %S. %n", wordVowels4, wordVowels4. longestVowelGrou
System.out.printin("Expected: ");
System.out.printf("Longest vowel group of \"%s\": %S.%n", wordVowels5, wordVowels5.
O 0 A1
41
42
43
44
45
46
47
48
49
50
ngestVowelGroup
O Restricted Mode
MacBook Air
80
888
000
esc
F1
F2
F3
F4
F5
F6
F7
!
#
$
&
@

Transcribed Image Text:print th(
ed: A");
System.out.println("Vowels of \"" + wordVowels4 + "\": " + wordVowels4.getVowels ());
System.out.println("Expected: ");
System.out.println("Vowels of \"" + wordVowels5 + "\": " + wordVowels5.getVowels ());
System.out.println("Expected: Iouioooai");
// Testing method vowelsFirst()
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels1, wordVowels1.vowelsFirst());
System.out.println("Expected: o0omnply");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels2, wordVowels2.vowelsFirst());
System.out.println("Expected: ouaeoutrgs");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels3, wordVowels3.vowelsFirst());
System.out.println("Expected: ACS 46");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels4, wordVowels4.vowelsFirst();
System.out.println("Expected: cS 46B");
System.out.printf("Vowel first of \"%s\": %s.%n", wordVowels5, wordVowels5.vowelsFirst());
System.out.println("Expected: Iouioooaintrdctn t Prgrmmng");
// Testing method longestVowelGroup()
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels1, wordVowels1. longestVowelGroup());
System.out.println("Expected: o");
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels2, wordVowels2. longestVowelGroup());
System.out.println("Expected: eou");
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels3, wordVowels3. longestVowelGroup(0);
System.out.println("Expected: A");
System.out.printf("Longest vowel group of \"%s\": %s.%n", wordVowels4, wordVowels4. longestVowelGroup());
System.out.println("Expected: ");
System.out.printf("Longest vowel group of \"&s\": %s.%n", wordVowels5, wordVowels5. longestVowelGroup());
System.out.println("Expected: io");
3
54
icted Mode
O0 A1
MacBook Air
80
888
DI
F1
F2
F3
F4
F5
F6
F7
F8
@
#
$
%
&
*
1
2
3
4
5
6
7
8
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
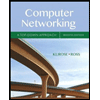
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
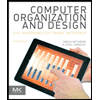
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
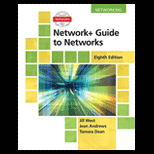
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
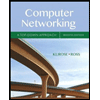
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
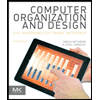
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
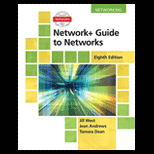
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
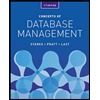
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
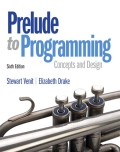
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
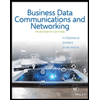
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY