private Object expression(Node booleanCompare, HashMap variables2) { // TODO Auto-generated method stub return null; } public Object interpretVariableReferenceNode(VariableReferenceNode varRefNode, HashMap variables) { String name = varRefNode.getName(); if(variables.containsKey(name)) { return variables.get(name); } else { throw new RuntimeException("Variable '" + name + "' does not exist."); } } public Object interpretMathOpNode(MathOpNode mathOpNode, HashMap variables) { Object leftNode = expression(mathOpNode.getLeft(), variables); Object rightNode = expression(mathOpNode.getRight(), variables); if(!(leftNode instanceof Double) || !(rightNode instanceof Double)) { throw new RuntimeException("Cannot add/subtract/multiply/divide/modulo " + leftNode + " and " + rightNode); } Double left = (Double) leftNode; Double right = (Double) rightNode; Object result = null; switch(mathOpNode.getOp()) { case "+": result = left + right; break; case "-": result = left - right; break; case "*": result = left * right; break; case "/": result = left / right; break; case "%": result = left % right; break; default: throw new RuntimeException("Unrecognized arithmetic operation"); } }
Java Programming: There are lots of errors in this code. Please help me fix them. Attached is images of what the code must have and circled the errors in the code.
Interpreter.java
public class Interpreter {
private HashMap<String, Object> variables;
public void interpretFunction(FunctionNode fn) {
// Create a hash map for local variables
variables = new HashMap<String, Object>();
for(Node constantNode : fn.getConstants()) {
Object constant = constantNode.getValue();
variables.put(constantNode.getName(), constant);
}
for(Node localVarNode : fn.getVariables()) {
Object localVar = null;
variables.put(localVarNode.getName(), localVar);
}
interpretBlock(fn.getStatements(), variables);
}
public void interpretBlock(List<StatementNode> statementNodes, HashMap<String, Object> variables) {
for(StatementNode statement : statementNodes) {
if(statement instanceof IfNode) {
interpretIfNode((IfNode) statement, variables);
} else if(statement instanceof VariableReferenceNode) {
interpretVariableReferenceNode((VariableReferenceNode) statement, variables);
} else if(statement instanceof MathOpNode) {
interpretMathOpNode((MathOpNode) statement, variables);
} else if(statement instanceof BooleanCompareNode) {
interpretBooleanCompareNode((BooleanCompareNode) statement, variables);
} else if(statement instanceof ForNode) {
interpretForNode((ForNode) statement, variables);
} else if(statement instanceof RepeatNode) {
interpretRepeatNode((RepeatNode) statement, variables);
} else if(statement instanceof ConstantNode) {
interpretConstantNode((ConstantNode) statement, variables);
} else if(statement instanceof WhileNode) {
interpretWhileNode((WhileNode) statement, variables);
} else if(statement instanceof AssignmentNode) {
interpretAssignmentNode((AssignmentNode) statement, variables);
} else if(statement instanceof FunctionCallNode) {
interpretFunctionCallNode((FunctionCallNode) statement, variables);
}
}
}
private void interpretFunctionCallNode(FunctionCallNode statement, HashMap<String, Object> variables2) {
// TODO Auto-generated method stub
}
private void interpretAssignmentNode(AssignmentNode statement, HashMap<String, Object> variables2) {
}
private void interpretWhileNode(WhileNode statement, HashMap<String, Object> variables2) {
}
private void interpretConstantNode(ConstantNode statement, HashMap<String, Object> variables2) {
}
public void interpretIfNode(IfNode ifNode, HashMap<String, Object> variables) {
Object result = expression(ifNode.getBooleanCompare(), variables);
if(result != null && (result instanceof Boolean && (Boolean) result)) {
interpretBlock(ifNode.getStatements(), variables);
} else if(ifNode.getNextIfNode() != null) {
interpretIfNode(ifNode.getNextIfNode(), variables);
}
}
private Object expression(Node booleanCompare, HashMap<String, Object> variables2) {
// TODO Auto-generated method stub
return null;
}
public Object interpretVariableReferenceNode(VariableReferenceNode varRefNode, HashMap<String, Object> variables) {
String name = varRefNode.getName();
if(variables.containsKey(name)) {
return variables.get(name);
} else {
throw new RuntimeException("Variable '" + name + "' does not exist.");
}
}
public Object interpretMathOpNode(MathOpNode mathOpNode, HashMap<String, Object> variables) {
Object leftNode = expression(mathOpNode.getLeft(), variables);
Object rightNode = expression(mathOpNode.getRight(), variables);
if(!(leftNode instanceof Double) || !(rightNode instanceof Double)) {
throw new RuntimeException("Cannot add/subtract/multiply/divide/modulo " + leftNode + " and " + rightNode);
}
Double left = (Double) leftNode;
Double right = (Double) rightNode;
Object result = null;
switch(mathOpNode.getOp()) {
case "+":
result = left + right;
break;
case "-":
result = left - right;
break;
case "*":
result = left * right;
break;
case "/":
result = left / right;
break;
case "%":
result = left % right;
break;
default:
throw new RuntimeException("Unrecognized arithmetic operation");
}
}
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

This is NOT a complete code. Give me the complete code!!!!!!!!
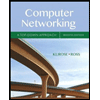
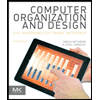
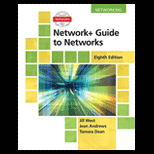
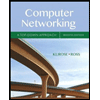
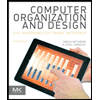
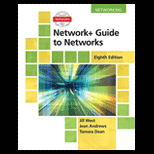
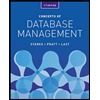
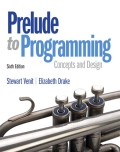
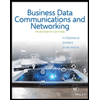