*code is provided and the error is in the picture, please help with how to fix the error*
import java.util.*;
import java.io.*;
public class HuffmanCode {
private Queue<HuffmanNode> queue;
private HuffmanNode overallRoot;
public HuffmanCode(int[] frequencies) {
queue = new PriorityQueue<HuffmanNode>();
for (int i = 0; i < frequencies.length; i++) {
if (frequencies[i] > 0) {
HuffmanNode node = new HuffmanNode(frequencies[i]);
node.ascii = i;
queue.add(node);
}
}
overallRoot = buildTree();
}
public HuffmanCode(Scanner input) {
overallRoot = new HuffmanNode(-1);
while (input.hasNext()) {
int asciiValue = Integer.parseInt(input.nextLine());
String code = input.nextLine();
overallRoot = reconstructTree(overallRoot, code, asciiValue);
}
}
private HuffmanNode buildTree() {
if (queue.size() == 1) {
return queue.remove();
} else {
HuffmanNode nodeLeft = queue.remove();
HuffmanNode nodeRight = queue.remove();
HuffmanNode nodeNew = new HuffmanNode(nodeLeft.frequency + nodeRight.frequency);
nodeNew.left = nodeLeft;
nodeNew.right = nodeRight;
queue.add(nodeNew);
return buildTree();
}
}
private HuffmanNode reconstructTree(HuffmanNode root, String code, int asciiValue) {
if (code.isEmpty()) {
root.ascii = asciiValue;
return root;
} else {
char next = code.charAt(0);
if (next == '0') {
if (root.left == null) {
root.left = new HuffmanNode(-1);
}
root.left = reconstructTree(root.left, code.substring(1), asciiValue);
} else if (next == '1') {
if (root.right == null) {
root.right = new HuffmanNode(-1);
}
root.right = reconstructTree(root.right, code.substring(1), asciiValue);
}
}
return root;
}
public void save(PrintStream output) {
save(output, overallRoot, "");
}
private void save(PrintStream output, HuffmanNode root, String code) {
if (root != null) {
if (root.ascii != -1) {
output.println(root.ascii);
output.println(code);
}
save(output, root.left, code + "0");
save(output, root.right, code + "1");
}
}
public void translate(BitInputStream input, PrintStream output) {
while (input.hasNextBit()) {
translate(overallRoot, input, output);
}
}
private void translate(HuffmanNode curr, BitInputStream input, PrintStream output) {
if (curr.left == null && curr.right == null) {
output.write(curr.ascii);
} else {
int token = input.nextBit();
if (token == 0) {
translate(curr.left, input, output);
} else {
translate(curr.right, input, output);
}
}
}
private static class HuffmanNode implements Comparable<HuffmanNode> {
public int frequency;
public int ascii;
public HuffmanNode left;
public HuffmanNode right;
public HuffmanNode(int frequency) {
this.frequency = frequency;
this.ascii = -1;
}
public int compareTo(HuffmanNode other) {
return this.frequency - other.frequency;
}
}
}
*code is provided and the error is in the picture, please help with how to fix the error*


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

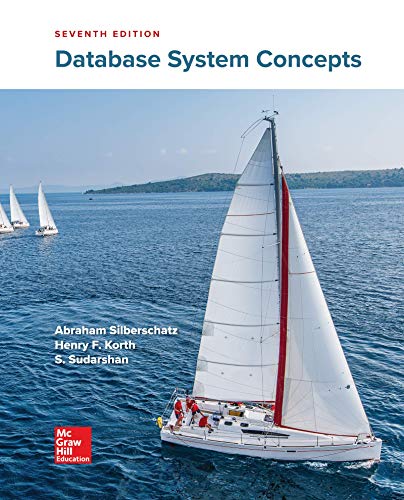
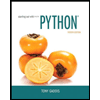
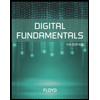
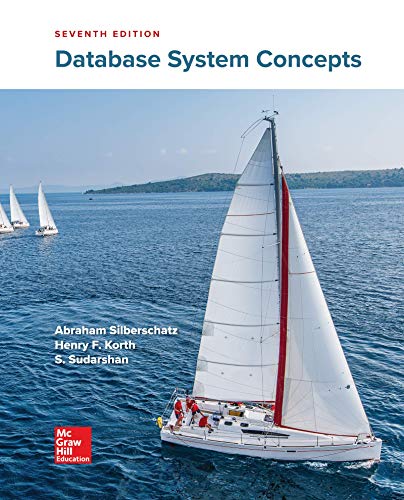
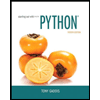
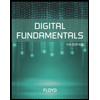
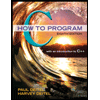
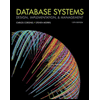
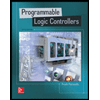