Line: 2 3 4 + + Result: 9 Line: 12 6 / 4 * 4 + Result: 12 Line: 2 2 3 3 * ** Result: 36 Line: 7 5 / 5 + 17 - Result: -11 Line: 4 3 - 5 6 + 4
VERY EASY RPN PROBLEM.
read the directions
please answer thank you
public class Node <T>
{
private T data;
private Node<T> next;
public Node(T data, Node<T> next)
{
this.data = data;
this.next = next;
}
public T getData()
{
return data;
}
public Node<T> getNext()
{
return next;
}
}
import java.util.Stack;
public class StackArray<T> implements Stack<T> {
private T [] elements;
private int top;
private int size;
public StackArray (int size)
{
elements = (T[]) new Object[size];
top = -1;
this.size = size;
}
public boolean empty()
{
return top == -1;
}
public boolean full()
{
return top == size - 1;
}
public boolean push(T el)
{
if (full())
return false;
else
{
top++;
elements[top] = el;
return true;
}
}
public T pop()
{
T el = elements[top];
top--;
return el;
}
}
// Generic Stack Reference Implementation
public class StackRef<T> implements Stack<T>
{
private Node<T> top;
public StackRef (int size)
{
top = null;
}
public StackRef()
{
top = null;
}
public boolean empty()
{
return top == null;
}
public boolean full()
{
return false;
}
public boolean push(T el)
{
Node<T> node = new Node<>(el, top);
top = node;
return true;
}
public T pop()
{
if (empty())
return null;
T el = top.getData();
top = top.getNext();
return el;
}
}
// Generic Stack Interface
public interface Stack<T>
{
public boolean empty();
public boolean full();
public boolean push(T el);
public T pop();
}
import java.util.Scanner;
import java.util.Stack;
import java.util.Set;
import java.io.*;
import java.util.*;
public class RPN {
private static Stack<String> stack;
private static FileReader file;
private static Scanner in;
private static Set<String> operators;
}



Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

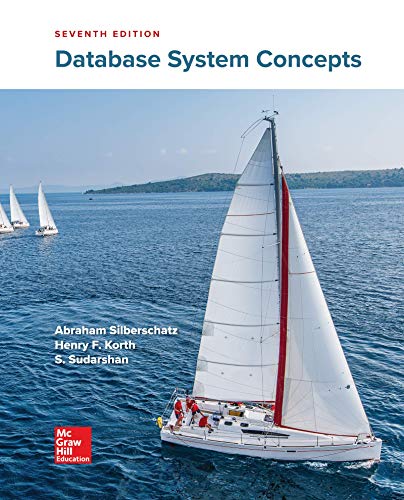
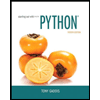
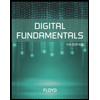
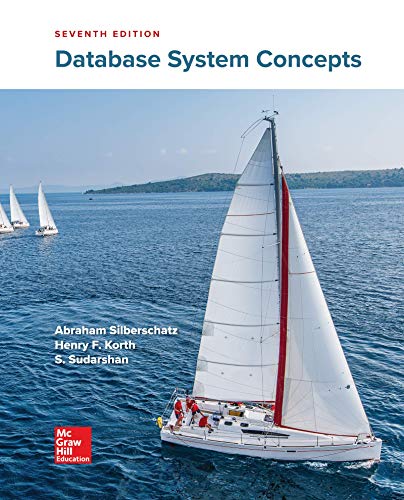
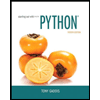
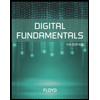
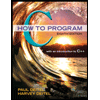
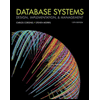
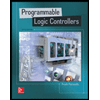