Change the __str__ method of the Queue class (provided below) so that it prints each object in the queue along with its order in the queue (see sample output below).
Change the __str__ method of the Queue class (provided below) so that it prints each object in the queue along with its order in the queue (see sample output below).
class Queue():
def __init__(self):
self.queue = [] # implement with Python lists!
# start of physical Python list == front of a queue
# end of physical Python list == back of a queue
def enqueue(self, new_obj):
self.queue.append(new_obj);
def dequeue(self):
return self.queue.pop(0)
def peek(self):
return self.queue[0]
def bad_luck(self):
return self.queue[-1]
def __str__(self):
return str(self.queue) # let's try a more fun way
Sample output:
>>> my_queue = Queue()
>>> everyone = ["ESC", "ABC", "YOLO", "HTC"]
>>> for initials in everyone:
>>> my_queue.enqueue(initials)
>>> print(my_queue)
Output:
1: ESC
2: ABC
3: YOLO
4: HTC

Step by step
Solved in 4 steps with 2 images

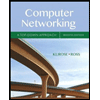
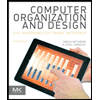
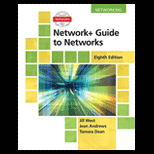
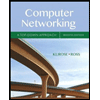
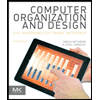
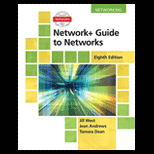
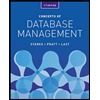
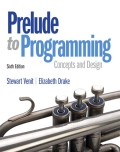
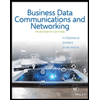