import java.util.*;
public class BST
{
// instance variables
private BSTNode m_root;
private int m_size;
// constructor
public BST()
{
m_root = null;
m_size = 0;
}
// This method returns the number of elements in the tree.
// Do not make any changes to this method!
public int size()
{
return m_size;
}
// This method clears the content of the tree.
// Do not make any changes to this method!
public void clear()
{
m_root = null;
m_size = 0;
}
// This non-recursive method takes a string and inserts it into the binary
// search tree, keeping the tree ordered.
public void add(String value)
{
// TODO: implement this method using a non-recursive solution
}
// This non-recursive method returns a string that represents the in-order traversal
// of the binary search tree.
public String inOrder()
{
// TODO: implement this method using a non-recursive solution
return ""; // replace this statement with your own return
}
// This method returns the largest element in the binary search tree. You
// are not allowed to create any additional structures, including but not
// limited to arrays, stacks, queues, or other trees.
public String max()
{
// TODO: implement this method
return ""; // replace this statement with your own return
}
// This method takes a reference to the root of the expression, evaluates
// the tree, and returns the result as an int.
public int evaluate(BSTNode node)
{
// TODO: implement this method
return -1; // replace this statement with your own return
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

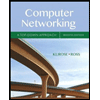
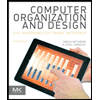
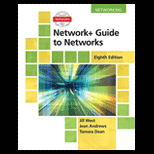
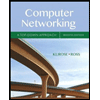
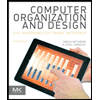
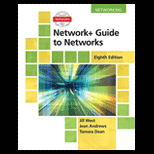
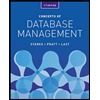
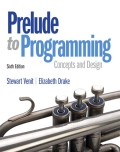
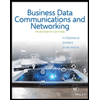