Implement a Doubly linked list to store a set of Integer numbers (no duplicate) • Instance variable • Constructor • Accessor and Update methods 2. Define DLinkedList Class a. Instance Variables: # Node header # Node trailer # int size b. Constructor c. Methods # int getSize() //Return the number of nodes of the list. # int getSize() //Return the number of elements of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # E getFirst() //Return the value of the first node of the list. # E getLast()/ /Return the value of the Last node of the list. # addFirst(E e) //Add a new node to the front of the list. # addLast(E e) //Add a new node to the end of the list. # Node remove(Node n) //remove a node which has the reference n from the list # Node removeFirst() //Remove the first node of the list, and return the removed node or null if failed. # Node removeLast() //Remove the last node of the list, and return the removed node or null if failed. # addBetween(E e, Node predecessor, Node successor) //Add a new node between the predecessor and successor. # Node search(E key) //Search and return a node according to a given key # Node update(E key, E e) //update the value of a given k to a new value. # display() //Display all nodes of the list by traversing the linked list. # addAfter(E e, E key) // Add a new node e after a given key of the list. # addBefore(E e, E key) // Add a new node e before a given key of the list. # Node removeAt(E key) //Remove node based on a given key, return the removed node or null if failed
Implement a Doubly linked list to store a set of Integer numbers (no duplicate)
• Instance variable
• Constructor
• Accessor and Update methods
2. Define DLinkedList Class
a. Instance Variables:
# Node header
# Node trailer
# int size
b. Constructor
c. Methods
# int getSize() //Return the number of nodes of the list.
# int getSize() //Return the number of elements of the list.
# boolean isEmpty() //Return true if the list is empty, and false otherwise.
# E getFirst() //Return the value of the first node of the list.
# E getLast()/ /Return the value of the Last node of the list.
# addFirst(E e) //Add a new node to the front of the list.
# addLast(E e) //Add a new node to the end of the list.
# Node remove(Node n) //remove a node which has the reference n from the list
# Node removeFirst() //Remove the first node of the list, and return the removed node or null if failed.
# Node removeLast() //Remove the last node of the list, and return the removed node or null if failed.
# addBetween(E e, Node predecessor, Node successor) //Add a new node between the predecessor and successor.
# Node search(E key) //Search and return a node according to a given key
# Node update(E key, E e) //update the value of a given k to a new value.
# display() //Display all nodes of the list by traversing the linked list.
# addAfter(E e, E key) // Add a new node e after a given key of the list.
# addBefore(E e, E key) // Add a new node e before a given key of the list.
# Node removeAt(E key) //Remove node based on a given key, return the removed node or null if failed

Step by step
Solved in 3 steps with 6 images

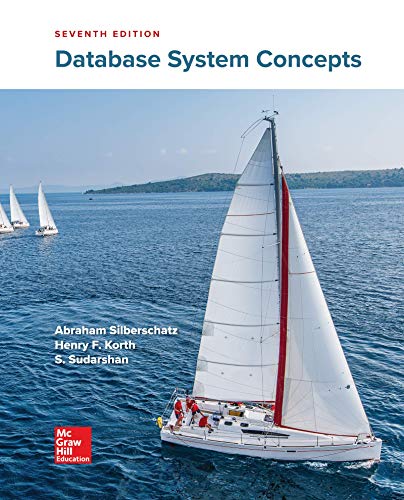
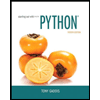
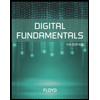
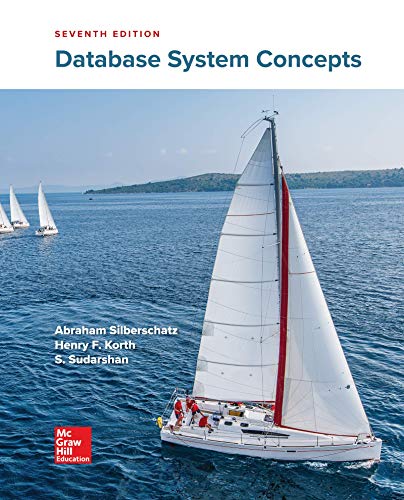
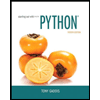
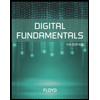
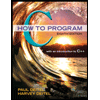
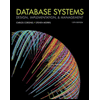
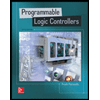