Implement LeafNode and InteriorNode classes for the expression tree as discussed on this page Use this template: Please don't change any function names Add any methods if necesssary TODO: Remove the pass statements and implement the methods. ''' class LeafNode: def __init__(self, data): self.data = data def postfix(self): return str(self) def __str__(self): return str(self.data) def prefix(self): pass def infix(self): pass def value(self): return self.data class InteriorNode: def __init__(self, op, left_op, right_op): self.op = op self.left_op = left_op self.right_op = right_op def postfix(self): return self.left_op.postfix() + " " + self.right_op.postfix() + " " + self.op def prefix(self): pass def infix(self): pass def value(self): pass if __name__ == "__main__": # TODO: (Optional) your test code here. a = LeafNode(4) b = InteriorNode('+', LeafNode(2), LeafNode(3)) c = InteriorNode('*', a, b) c = InteriorNode('-', c, b)
Implement LeafNode and InteriorNode classes for the expression tree as discussed on this page
Use this template:
Please don't change any function names
Add any methods if necesssary
TODO: Remove the pass statements and implement the methods.
'''
class LeafNode:
def __init__(self, data):
self.data = data
def postfix(self):
return str(self)
def __str__(self):
return str(self.data)
def prefix(self):
pass
def infix(self):
pass
def value(self):
return self.data
class InteriorNode:
def __init__(self, op, left_op, right_op):
self.op = op
self.left_op = left_op
self.right_op = right_op
def postfix(self):
return self.left_op.postfix() + " " + self.right_op.postfix() + " " +
self.op
def prefix(self):
pass
def infix(self):
pass
def value(self):
pass
if __name__ == "__main__":
# TODO: (Optional) your test code here.
a = LeafNode(4)
b = InteriorNode('+', LeafNode(2), LeafNode(3))
c = InteriorNode('*', a, b)
c = InteriorNode('-', c, b)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

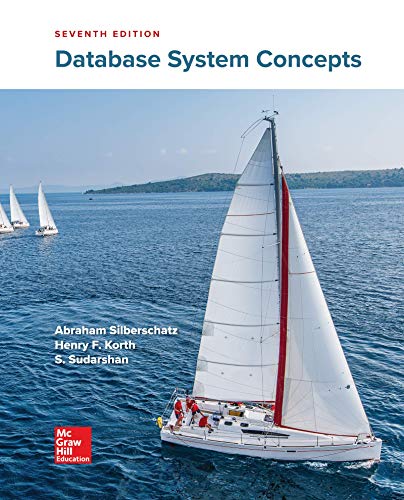
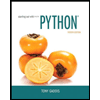
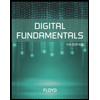
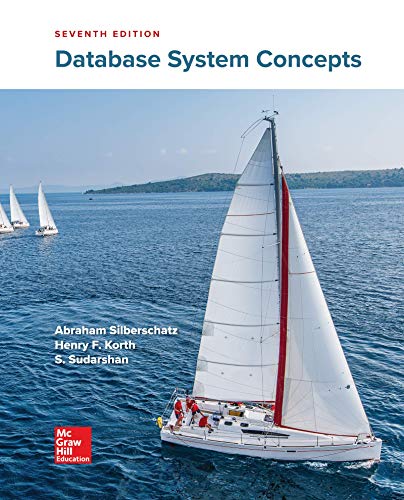
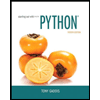
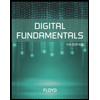
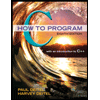
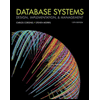
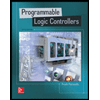