How can I / where can I add more / better comments and improve the overall Java code structure to enhance its readability and maintainability.
How can I / where can I add more / better comments and improve the overall Java code structure to enhance its readability and maintainability.
Source code:
package bankAccount;
import java.awt.Label;
import java.awt.TextField;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class GUIBankAccount extends JPanel implements ActionListener { //Detects when the user clicks an on screen button on panel.
//New Jbuttons
JButton deposit = new JButton("Deposit");
JButton withdrawal = new JButton("Withdrawal");
JButton balance = new JButton("Add Balance");
JButton exit = new JButton("Exit");
TextField depo, withdraw, bal, output; //Text fields
Label depositAccount, withdrawalAccount, addBalance, balanceTotal; //Labels
double accountBalance = 0.0; //initializing balance to zero.
public GUIBankAccount(JFrame frame) {
//Deposit section.
//Creating labels, boundaries, text box, action listener and adding them all to frame.
depositAccount = new Label("Deposit");
depositAccount.setBounds(90, 115, 60, 50);
frame.add(depositAccount);
depo = new TextField("");
depo.setBounds(150, 125, 150, 30);
frame.add(depo);
deposit.addActionListener(this);
deposit.setBounds(450, 125, 150, 30);
frame.add(deposit);
withdrawalAccount = new Label("Withdrawal");
withdrawalAccount.setBounds(80, 190, 60, 50);
frame.add(withdrawalAccount);
withdraw = new TextField("");
withdraw.setBounds(150, 200, 150, 30);
frame.add(withdraw);
withdrawal.addActionListener(this);
withdrawal.setBounds(450, 200, 150, 30);
frame.add(withdrawal);
addBalance = new Label("Add Balance");
addBalance.setBounds(70, 40, 70, 30);
frame.add(addBalance);
bal = new TextField("");
bal.setBounds(150, 40, 150, 30);
frame.add(bal);
balance.addActionListener(this);
balance.setBounds(450, 40, 150, 30);
frame.add(balance);
balanceTotal = new Label("Balance");
balanceTotal.setBounds(90, 280, 60, 30);
frame.add(balanceTotal);
output = new TextField("");
output.setBounds(150, 280, 150, 30);
frame.add(output);
exit.addActionListener(this);
exit.setBounds(450, 280, 150, 30);
frame.add(exit);
}
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.getContentPane().add(new GUIBankAccount(frame));
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(700, 400);
frame.setTitle("Bank Account GUI");
frame.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("Deposit")) {
System.out.println("Deposit button has been clicked");
String balanceTotal = depo.getText(); //Getting input.
double depositAccount = Double.parseDouble(balanceTotal); //Method returns a new double initialized to the value.
accountBalance = accountBalance + depositAccount; //Adding funds to balance.
output.setText(String.valueOf(accountBalance)); //Setting new value
depo.setText("");//Clearing text in deposit text box.
}
//Withdrawal section
else if (e.getActionCommand().equals("Withdrawal")) {
System.out.println("Withdrawal button has been clicked");
String balanceTotal = withdraw.getText();
double withdrawalAccount = Double.parseDouble(balanceTotal);
accountBalance = accountBalance - withdrawalAccount;
output.setText(String.valueOf(accountBalance));
withdraw.setText("");
}
else if (e.getActionCommand().equals("Add Balance")) {
System.out.println("Add Balance button has been clicked");
String balanceTotal = bal.getText();
double addBalance = Double.parseDouble(balanceTotal);
accountBalance = accountBalance + addBalance;
output.setText(String.valueOf(accountBalance));
bal.setText("");
}
else if(e.getActionCommand().equals("Exit")) {
System.out.println("User has pressed exit. Good Bye");
System.exit(0);
}
}
}

Step by step
Solved in 4 steps with 8 images

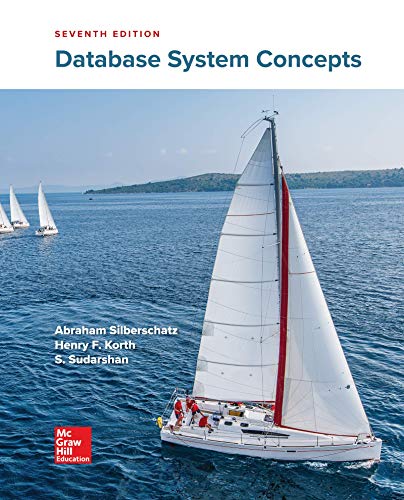
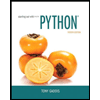
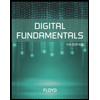
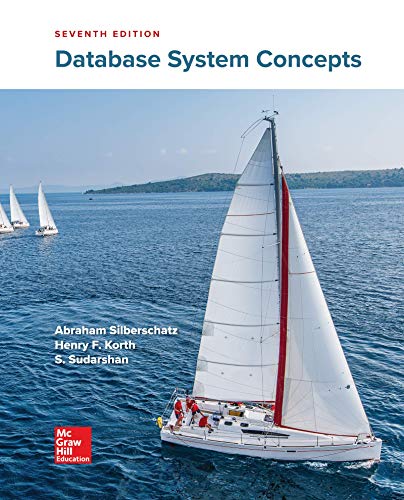
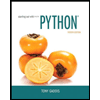
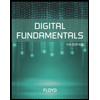
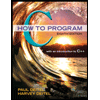
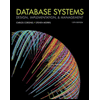
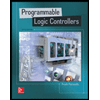